Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) • Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex: If the program has 4 students enrolled in the course, the output looks like: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4
Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) • Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex: If the program has 4 students enrolled in the course, the output looks like: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 18RQ
Related questions
Question
public class LabProgram {
public static void main(String args[]) {
Course course = new Course();
String first; // first name
String last; // last name
double gpa; // grade point average
first = "Henry";
last = "Cabot";
gpa = 3.5;
course.addStudent(new Student(first, last, gpa)); // Add 1st student
first = "Brenda";
last = "Stern";
gpa = 2.0;
course.addStudent(new Student(first, last, gpa)); // Add 2nd student
first = "Jane";
last = "Flynn";
gpa = 3.9;
course.addStudent(new Student(first, last, gpa)); // Add 3rd student
first = "Lynda";
last = "Robison";
gpa = 3.2;
course.addStudent(new Student(first, last, gpa)); // Add 4th student
course.printRoster();
}
}
// Class representing a student
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average
// Student class constructor
public Student(String first, String last, double gpa) {
this.first = first; // first name
this.last = last; // last name
this.gpa = gpa; // grade point average
}
public double getGPA() {
return gpa;
}
public String getLast() {
return last;
}
// Returns a String representation of the Student object, with the GPA formatted to one decimal
public String toString() {
DecimalFormat fmt = new DecimalFormat("#.0");
return first + " " + last + " " + "(GPA: " + fmt.format(gpa) + ")";
}
}

Transcribed Image Text:Complete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the
total number of students in the course.
Given classes:
• Class LabProgram contains the main method for testing the program.
• Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here)
• Class Student represents a classroom student, which has three fields: first name, last name, and GPA.
Ex: If the program has 4 students enrolled in the course, the output looks like:
Henry Cabot (GPA: 3.5)
Brenda Stern (GPA: 2.0)
Jane Flynn (GPA: 3.9)
Lynda Robison (GPA: 3.2)
Students: 4

Transcribed Image Text:2
3 public class Course {
4
private ArrayList<Student> roster; //collection of Student objects
567 00
8
9
10
11
12
13
14
15
16
17
18 }
public Course() {
roster=new ArrayList<Student> ();
}
public void printRoster () {
/* Type your code here */
Current file: Course.java →
}
public void addStudent (Student s) {
roster.add(s);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
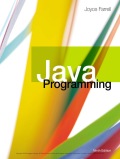
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
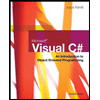
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
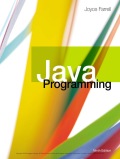
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
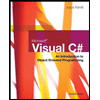
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage