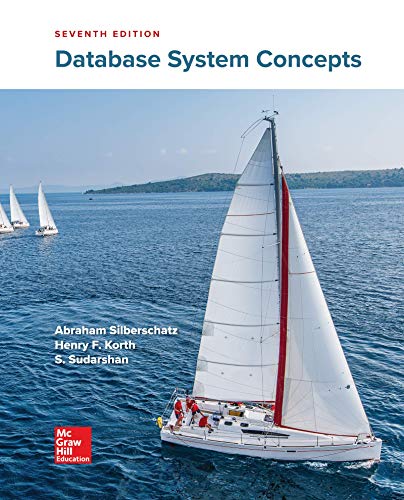
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
Course course = new Course();
int beforeCount;
// Example students for testing
course.addStudent(new Student("Henry", "Nguyen", 3.5));
course.addStudent(new Student("Brenda", "Stern", 2.0));
course.addStudent(new Student("Lynda", "Robison", 3.2));
course.addStudent(new Student("Sonya", "King", 3.9));
Student student = course.findStudentHighestGpa();
System.out.println("Top student: " + student);
}
}
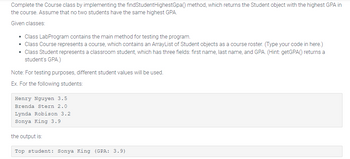
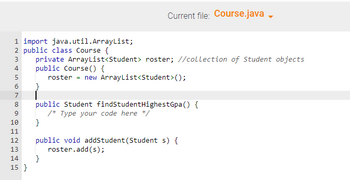

1. Create a class named Student with private fields: first (String), last (String), and gpa (double).
2. Implement a constructor in the Student class to initialize first, last, and gpa.
3. Implement getGPA() method in the Student class to retrieve the GPA of the student.
4. Override toString() method in the Student class to return a formatted string representation of the student.
5. Create a class named Course with an ArrayList of Student objects named roster.
6. Implement a method named findStudentHighestGpa() in the Course class.
7. Initialize a Student object variable (highestGpaStudent) to null to keep track of the student with the highest GPA.
8. Iterate through the roster list:
a. For each student, compare their GPA with the GPA of highestGpaStudent.
b. If the student's GPA is higher, update highestGpaStudent with the current student.
9. After iterating through the roster, highestGpaStudent will contain the student with the highest GPA.
10. Return highestGpaStudent from the findStudentHighestGpa() method.
Step by stepSolved in 4 steps with 4 images

- public class Artwork { // TODO: Declare private fields - title, yearCreated // TODO: Declare private field artist of type Artist // TODO: Define default constructor // TODO: Define get methods: getTitle(), getYearCreated() // TODO: Define second constructor to initialize // private fields (title, yearCreated, artist) // TODO: Define printInfo() method // Call the printInfo() method in Artist.java to print an artist's information }} public class ArtworkLabel { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userTitle, userArtistName; int yearCreated, userBirthYear, userDeathYear; userArtistName = scnr.nextLine(); userBirthYear = scnr.nextInt(); scnr.nextLine(); userDeathYear = scnr.nextInt(); scnr.nextLine(); userTitle = scnr.nextLine(); yearCreated =…arrow_forwardJava A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forwardC# List the differences between CommissionEmployee class and BasePlusCommissionEmployee class public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; private decimal commissionRate; private decimal baseSalary; public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; CommissionRate = commissionRate; BaseSalary = baseSalary; } public decimal GrossSales { get { return grossSales; } set { if (value < 0) // validation { throw new ArgumentOutOfRangeException(nameof(value),…arrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardProgram: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include <stdlib.h> #include "GamePurse.h" #include<iostream> #include<time.h> using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the…arrow_forwardSubclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forward
- interface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forwardC# Solve this error using System; namespace RahmanA3P1BasePlusCEmployee { public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; // gross weekly sales private decimal commissionRate; // commission percentage private decimal baseSalary; // base salary per week // six-parameter constructor public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { // implicit call to object constructor occurs here FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; // validates gross sales CommissionRate = commissionRate; // validates commission rate BaseSalary = baseSalary; // validates base…arrow_forward// This class discounts prices by 10% public class DebugFour4 { public static void main(String args[]) { final double DISCOUNT_RATE = 0.90; int price = 100; double price2 = 100.00; tenPercentOff(price DISCOUNT_RATE); tenPercentOff(price2 DISCOUNT_RATE); } public static void tenPercentOff(int p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println("Ten percent off " + p); System.out.println(" New price is " + newPrice); } public static void tenPercentOff(double p, final double DISCOUNT_RATE) { double newPrice = p * DISCOUNT_RATE; System.out.println"Ten percent off " + p); System.out.println(" New price is " + newprice); } }arrow_forward
- public class Author2 {3 private String name;4 private int numberOfAwards;5 private boolean guildMember;6 private String[] bestsellers;78 public String Author()9 {10 name = "Grace Random";11 numberOfAwards = 0;12 guildMember = false;13 bestsellers = {"Minority Report", "Ubik", "The Man in the HighCastle"};14 }1516 public void setName(String n)17 {18 name = n;19 }20 public String getName()21 {22 return name;23 }2425 public void winsAPulitzer()26 {27 System.out.println(name + " gave a wonderful speech!");28 }29 } 1. This class includes a constructor that begins on line 8; however, it contains an error. Describe the error. 2. Rewrite the constructor header on line 8 with the error identied in part c of this question corrected. 3. Demonstrate how you might overload the constructor for this class. Write only the header. 4. Write a line of Java code to create an instance (object) of this class. Name it someAuthor. 5. Write a line of code to demonstrate how you would use the someAuthor object…arrow_forwardJava script. class Chameleon { static colorChange(newColor) { this.newColor = newColor; return this.newColor; } constructor({ newColor = 'green' } = {}) { this.newColor = newColor; } } const freddie = new Chameleon({ newColor: 'purple' }); console.log(freddie.colorChange('orange'));.arrow_forwardpublic class Cat extends Pet { private String breed; public void setBreed(String userBreed) { breed = userBreed; } public String getBreed() { return breed; }} public class Pet { protected String name; protected int age; public void setName(String userName) { name = userName; } public String getName() { return name; } public void setAge(int userAge) { age = userAge; } public int getAge() { return age; } public void printInfo() { System.out.println("Pet Information: "); System.out.println(" Name: " + name); System.out.println(" Age: " + age); } } import java.util.Scanner; public class PetInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); // create a generic Pet and a Cat Pet myPet = new Pet(); Cat myCat = new Cat(); // declare variables for pet and cat info String petName, catName, catBreed; int petAge,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
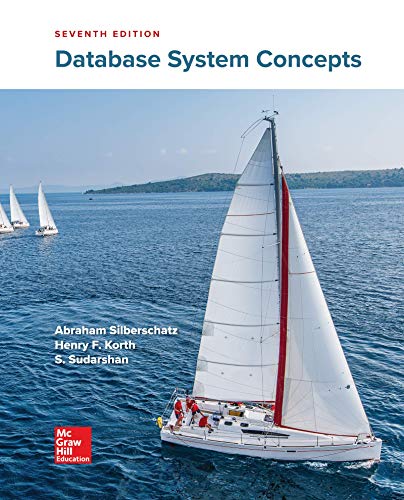
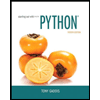
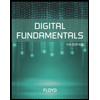
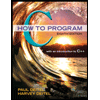
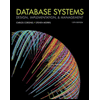
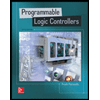