Need to fill the blanks. #include #include #include<__A__> // this library is needed to use exit(0) // Define upper (tallest) and lower bounds to check chances. Convert 5'11 and 8'11 to inches #define upper 107 #define lower 71 /*Return False (invalid) if: # of feet is negative, # of inches is negative, # of inches is 12 or more. and True otherwise */ __B__ checkValid(__C__ ft, __D__ in) { __E__ ((ft <= 0) || (__F__ <= 0) || (__G__ __H__ 12)) ? false : true; } /*Check height against the lower and upper bound defined above lower = 5'11 = 71 inches upper = 8'11 = 107 inches and print according messages - slim chance: < 5’11 - good chance: between 5’11 and 8’11 inclusive (tallest person record) - Guinness record: > 8’11 */ __I__ chances(__J__ height) { if(__K__ < __L__) printf("Your chance is pretty slim, but not impossible standing at %d inches tall\n", __M__); else if ((__N__ >= __O__) && (__P__ <= __Q__)) printf("Looking good! Your chance is pretty high at %d inches tall.\n", __R__); else printf("You should go for the Guinness record at %d inches tall\n",__S__); } /* Get student height, then call checkValid function to check for inputted height If height is invalid, exit the program If height is valid, return the height in inches */ __T__ getHeight() { int ft, in; printf("Need 2 numbers. Enter height (feet then inches): "); __U__(" %__V__ %__W__", &__X__, &__Y__); /* check for valid height. look at called function header to parameters return false if height is not valid, and true otherwise */ __Z__ isValid = checkValid(ft, __AA__); if(__BB__) // check for invalid input { printf("Invalid input! Exiting..."); exit(0); //terminate the program } //return height in inches by multiply # of feet by 12 plus # of inches __CC__ (__DD__ * 12 + __EE__); } int main() { int num_students; printf("How many students please: "); scanf("__FF__",__GG__); // loop through all students for(int i = 0 ; i < __HH__; i++) { printf("Processing Student %d :\n",i+1); __II__ height = getHeight(); // get student height chances(__JJ__); //get their chances } return 0; }
Need to fill the blanks.
#include<stdio.h>
#include<stdbool.h>
#include<__A__> // this library is needed to use exit(0)
// Define upper (tallest) and lower bounds to check chances. Convert 5'11 and 8'11 to inches
#define upper 107
#define lower 71
/*Return False (invalid) if:
# of feet is negative,
# of inches is negative,
# of inches is 12 or more. and True otherwise
*/
__B__ checkValid(__C__ ft, __D__ in)
{ __E__ ((ft <= 0) || (__F__ <= 0) || (__G__ __H__ 12)) ? false : true;
}
/*Check height against the lower and upper bound defined above
lower = 5'11 = 71 inches
upper = 8'11 = 107 inches
and print according messages
- slim chance: < 5’11
- good chance: between 5’11 and 8’11 inclusive (tallest person record)
- Guinness record: > 8’11
*/
__I__ chances(__J__ height)
{ if(__K__ < __L__)
printf("Your chance is pretty slim, but not impossible standing at %d inches tall\n", __M__);
else if ((__N__ >= __O__) && (__P__ <= __Q__))
printf("Looking good! Your chance is pretty high at %d inches tall.\n", __R__);
else
printf("You should go for the Guinness record at %d inches tall\n",__S__);
}
/*
Get student height, then call checkValid function to check for inputted height
If height is invalid, exit the program
If height is valid, return the height in inches
*/
__T__ getHeight()
{ int ft, in;
printf("Need 2 numbers. Enter height (feet then inches): ");
__U__(" %__V__ %__W__", &__X__, &__Y__);
/*
check for valid height. look at called function header to parameters return false if height is not valid, and true otherwise
*/
__Z__ isValid = checkValid(ft, __AA__);
if(__BB__) // check for invalid input
{ printf("Invalid input! Exiting...");
exit(0); //terminate the program }
//return height in inches by multiply # of feet by 12 plus # of inches
__CC__ (__DD__ * 12 + __EE__);
}
int main()
{
int num_students;
printf("How many students please: ");
scanf("__FF__",__GG__);
// loop through all students
for(int i = 0 ; i < __HH__; i++)
{
printf("Processing Student %d :\n",i+1);
__II__ height = getHeight(); // get student height
chances(__JJ__); //get their chances
}
return 0;
}

Step by step
Solved in 2 steps

The above codes have many errors. Would you please recheck and run the code before posting the answer? Thank you.
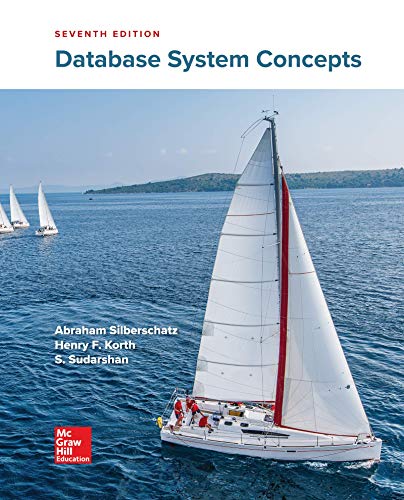
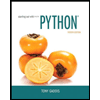
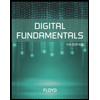
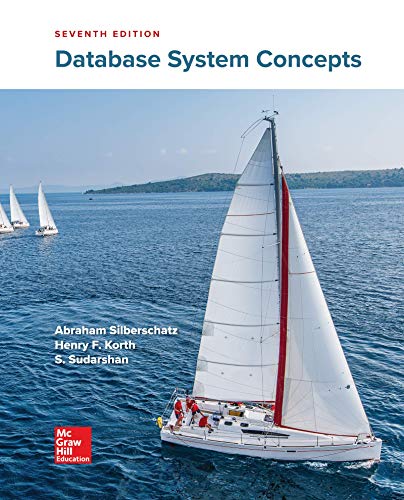
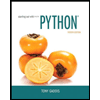
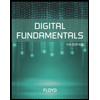
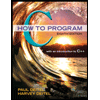
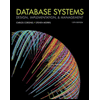
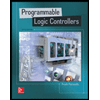