This code is correct but can you add to the code this instructions: If the father's age is equal to the mother's age and the age is odd, then the child is Female The code should call `createKidPerson` in main. #include using namespace std; typedef struct { int age; char gender; } Person; void displayPerson(Person); Person createKidPerson(Person father, Person mother); int main(void) { Person father; Person mother; father.gender = 'M'; mother.gender = 'F'; //ask the user for the age of the Male Person and the age of the Female Person. cout<<"Enter the Male Person's age: "; cin>>father.age; cout<<"Enter the Female Person's age: "; cin>>mother.age; //create a new Person and call createKidPerson() function Person kid = createKidPerson(father, mother); displayPerson(kid); return 0; } Person createKidPerson(Person father, Person mother) { Person p; if(father.age>=mother.age) //if father age more or same as mother age p.gender='M'; else //if mother age is more p.gender='F'; p.age=1; //set age return p; } void displayPerson(Person p) { cout << "\nPERSON DETAILS:" << endl; cout << "Age: " << p.age << endl; cout << "Gender: "; if(p.gender == 'M') { cout << "Male"; } else { cout << "Female"; } }
This code is correct but can you add to the code this instructions:
- If the father's age is equal to the mother's age and the age is odd, then the child is Female
- The code should call `createKidPerson` in main.
#include <iostream>
using namespace std;
typedef struct {
int age;
char gender;
} Person;
void displayPerson(Person);
Person createKidPerson(Person father, Person mother);
int main(void) {
Person father;
Person mother;
father.gender = 'M';
mother.gender = 'F';
//ask the user for the age of the Male Person and the age of the Female Person.
cout<<"Enter the Male Person's age: ";
cin>>father.age;
cout<<"Enter the Female Person's age: ";
cin>>mother.age;
//create a new Person and call createKidPerson() function
Person kid = createKidPerson(father, mother);
displayPerson(kid);
return 0;
}
Person createKidPerson(Person father, Person mother)
{
Person p;
if(father.age>=mother.age) //if father age more or same as mother age
p.gender='M';
else //if mother age is more
p.gender='F';
p.age=1; //set age
return p;
}
void displayPerson(Person p)
{
cout << "\nPERSON DETAILS:" << endl;
cout << "Age: " << p.age << endl;
cout << "Gender: ";
if(p.gender == 'M') {
cout << "Male";
} else {
cout << "Female";
}
}

Step by step
Solved in 4 steps with 3 images

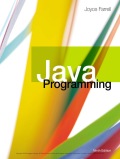
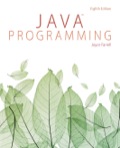
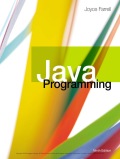
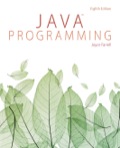