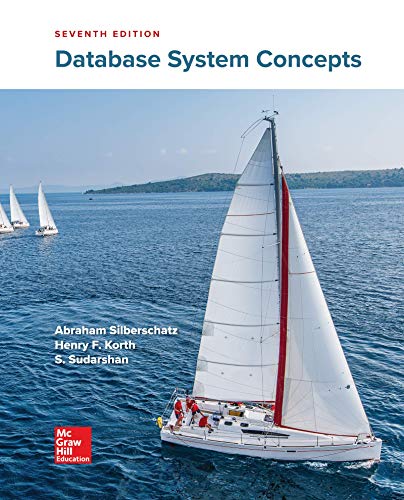
- getRandomLib.h:
// This library provides a few helpful functions to return random values
// * getRandomInt() - returns a random integer between min and max values // * getRandomFraction() - returns a random float between 0 and 1
// * getRandomFloat() - returns a random float between min and max values // * getRandomDouble() - returns a random double between min and max values
// * getRandomBool() - returns a random bool value (true or false)
// * getRandomUpper() - returns a random char between 'A' and 'Z', or between min/max values
// * getRandomLower() - returns a random char between 'a' and 'z', or between min/max values
// * getRandomAlpha() - returns a random char between 'A' and 'Z' or 'a' and 'z'
// * getRandomDigit() - returns a random char between '0' and '9', or between min/max values
// * getRandomChar() - returns a random printable char //
// #define GET_RANDOM_LIB_H #include <cstdlib> #include <random> #include <ctime> using namespace std; const int MIN_UPPER = 65; const int MAX_UPPER = 90; const int MIN_LOWER = 97; const int MAX_LOWER = 122; const int MIN_DIGIT = 48; const int MAX_DIGIT = 57; const int MIN_CHAR = 33; const int MAX_CHAR = 126;
//unsigned seed = time(0); int getRandomInt(int min, int max) {
//seed random engine static default_random_engine gen((unsigned int)time(0));
// if max < min, then swap if (min > max) { int temp = min; min = max; max = temp; }
//set up random generator uniform_int_distribution<int> dis(min, max); //range
//need to throw away the first call, as it is always max... static int firstCall = dis(gen); return dis(gen); }
//end getRandomInt() float getRandomFraction() {
//seed random engine static unsigned seed = time(0); return rand()
/ static_cast<float>(RAND_MAX); }
//end getRandomFraction() float getRandomFloat(float min, float max) { if (min > max) { float temp = min; min = max; max = temp; } return (getRandomFraction() * (max - min)) + min; } //end getRandomFloat() double getRandomDouble(double min, double max) { if (min > max) { double temp = min; min = max; max = temp; } return (static_cast<double>(getRandomFraction()) * (max - min)) + min; } //end getRandomDouble() bool getRandomBool() { if (getRandomInt(0,1) == 0) return false; else return true; } //end getRandomBool char getRandomUpper() { return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER)); } //end getRandomUpper() char getRandomUpper(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomUpper() char getRandomLower() { return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER)); } //end getRandomLower() char getRandomLower(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomLower() char getRandomAlpha() { if (getRandomBool()) return getRandomUpper(); else return getRandomLower(); } //end getRandomAlpha() char getRandomDigit() { return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT)); } //end getRandomDigit() char getRandomDigit(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); } //end getRandomDigit() char getRandomChar() { return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR)); } //end getRandomChar()
//end getRandomFloat() double getRandomDouble(double min, double max) { if (min > max) { double temp = min; min = max; max = temp; } return (static_cast<double>(getRandomFraction()) * (max - min)) + min; }
//end getRandomDouble() bool getRandomBool() { if (getRandomInt(0,1) == 0) return false; else return true; }
//end getRandomBool char getRandomUpper() { return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER)); }
//end getRandomUpper() char getRandomUpper(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomUpper() char getRandomLower() { return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER)); }
//end getRandomLower() char getRandomLower(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomLower() char getRandomAlpha() { if (getRandomBool()) return getRandomUpper(); else return getRandomLower(); }
//end getRandomAlpha() char getRandomDigit() { return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT)); }
//end getRandomDigit() char getRandomDigit(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max))); }
//end getRandomDigit() char getRandomChar() { return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR)); }
//end getRandomChar()
#endif
- responses.txt
Yes, of course!
Without a doubt, yes.
You can count on it.
For sure! Ask me later.
I'm not sure.
I can't tell you right now.
I'll tell you after my nap.
No way! I don't think so.
Without a doubt, no.
The answer is clearly NO.
Create a new file (in Dev C++) and save it as lab13_XYZ.cpp (replace XYZ with your initials).
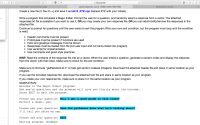

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- What happens when an object such as an array is no longer referenced by a variable?arrow_forwardAssignment 5A: Multiple Frequencies. In the last assignment, we calculated the frequency of a coin flip. This required us to have two separate variables, which we used to record the number of heads and tails. Now that we know about arrays, we can track the frequency of all numbers in a randomly generated sequence. For this program, you will ask the user to provide a range of values (from 1 to that number, inclusive) and how long of a number sequence you want to generate using that number range. You will then generate and save the sequence in an array. After that, you will count the number of times each number occurs in the sequence, and print the frequency of each number. Hints: You can use multiple arrays for this assignment. One array should hold the number sequence, and another could keep track of the frequencies of each number. Sample Output #1: What's the highest number you want to generate?: 5 How Long of a number sequence do you want to generate?: 10 Okay, we'll generate 10…arrow_forwarddef tpr(tp, fn): # TODO return def TEST_tpr(): tpr_score = tpr(tp=100, fn=1) todo_check([ (np.isclose(tpr_score,0.99009, rtol=.01),"tpr_score is incorrect") ]) TEST_tpr() garbage_collect(['TEST_tpr'])arrow_forward
- Computer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forwardCan you fix the code please on the first picture shows the error output. // Corrected code #define _CRT_SECURE_NO_WARNINGS #include "LibraryManagement.h" #include "Books.h" #include "DigitalMedia.h" #include "LibraryConfig.h" #include #include #include #include // Include the necessary header for boolean data type // Comparison function for qsort to sort Digital Media by ID int compareDigitalMedia(const void* a, const void* b) { return ((struct DigitalMedia*)a)->id - ((struct DigitalMedia*)b)->id; } // initializing library struct Library initializeLibrary() { struct Library lib; lib.bookCount = 0; lib.ebookCount = 0; lib.digitalMediaCount = 0; // Initialize book array for (int i = 0; i < MAX_BOOK_COUNT; i++) { lib.books[i].commonAttributes.id = -1; // Set an invalid ID to mark empty slot } // Initialize ebook array for (int i = 0; i < MAX_EBOOK_COUNT; i++) { lib.ebooks[i].commonAttributes.id = -1; }…arrow_forward
- my_round(number, integer): Based on the built-in round(...) function. Takes an integer or float and returns a rounded float value that is the number rounded to the second argument's decimal place. First argument can be any float or integer. Second argument must be an integer. Examples: my_round(1234.5678, 2) and round(1234.5678, 2) should return 1234.57. my_round(1234.5678, -2) and round(1234.5678, -2) should return 1200.0.arrow_forward//Assignment 06 */public static void main[](String[] args) { String pass= "ICS 111"; System.out.printIn(valPassword(pass));} /* public static boolean valPassword(String password){ if(password.length() > 6) { if(checkPass(password) { return true; } else { return false; } }else System.out.print("Too small"); return false;} public static boolean checkPass (String password){ boolean hasNum=false; boolean hasCap = false; boolean hasLow = false; char c; for(int i = 0; i < password.length(); i++) { c = password.charAt(1); if(Character.isDigit(c)); { hasNum = true; } else if(Character.isUpperCase(c)) { hasCap = true; } else if(Character.isLowerCase(c)) { hasLow = true; } } return true; { return false; } }arrow_forwardFleet class: Instance Variables An array that stores Aircraft that represents Delta Airlines entire fleet A variable that represents the count for the number of aircraft in the fleet Methods: o Constructor-One constructor that instantiates the array and sets the count to aero readFile()-This method accepts a string that represents the name of the file to be read. It will then read the file. Once the data is read, it should create an aircraft and then pass the vehicle to the addAircraft method. It should not allow any duplication of records. Be sure to handle all exceptions. o writeFile()-This method accepts a string that represents the name of the file to be written to and then writes the contents of the array to a file. This method should call a sort method to sort the amay before writing to it. sortArray()-This method returns a sorted array. The array is sorted by registration number.u addAircraft- This method accepts an aircraft and adds it to the fleet(the array only if it is not…arrow_forward
- Counting hashtags Write Python code to count the frequency of hashtags in a twitter feed. Your code assumes a twitter feed variable tweets exists, which is a list of strings containing tweets. Each element of this list is a single tweet, stored as a string. For example, tweets may look like: tweets = ["Happy #IlliniFriday!", "It is a pretty campus, isn't it, #illini?", "Diving into the last weekend of winter break like... #ILLINI #JoinTheFight", "Are you wearing your Orange and Blue today, #Illini Nation?"] Your code should produce a sorted list of tuples stored in hashtag_counts, where each tuple looks like (hashtag, count), hashtag is a string and count is an integer. The list should be sorted by count in descending order, and if there are hashtags with identical counts, these should be sorted alphabetically, in ascending order, by hashtag. From the above example, our unsorted hashtag_counts might look like: [('#illini', 2), ('#jointhefight', 1),…arrow_forwardA file USPopulation.txt contains the population of the US starting in year 1950 and then each subsequent record has the population for the following year. USPopulation.txtDownload USPopulation.txt Write a program that uses an array with the file that displays these in a menu and then produces the results. This is not an Object Oriented Program but should be a procedural program, calling methods to do the following: 1: Displays the year and the population during that year 2. The average population during that time period (Add up the populations of all records and divide by the number of years). 3. The year with the greatest increase in population - print the year and that population and that amount. To figure this out, compare the difference in population before of say year 1950 and 1951, store that difference somewhere. Compare 1951 with 1952, find that difference. Is that difference greater than the stored difference? If so, move that the the maximum place. 4.…arrow_forwardCreate a Console application that does the following:This program must use a single array to store integers and find the minimum value.1. Create an array that can hold up to 100 integers.2. Prompt the user for the number of integers to be stored in the array.3. Prompt the user for the integers to be stored in the array.4. Use a loop to iterate through the array and determine the minimum value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
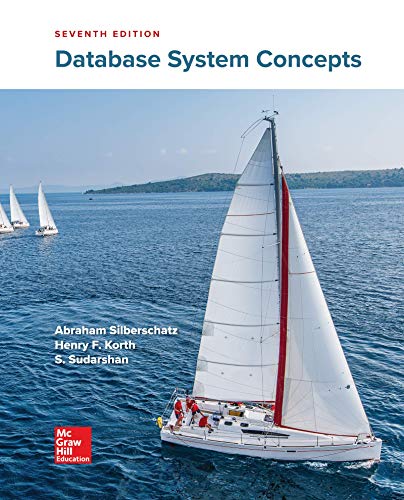
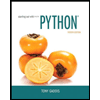
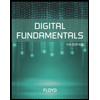
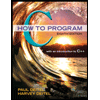
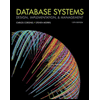
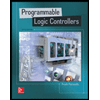