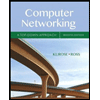
python:
def txt_to_csv(input_file):
"""
Question 3
- cats.txt contains information about cat breeds and their various characteristics.
- Read the cats.txt with FILE I/O.
- These are TAB separated values
- Use a list of lists to create a CSV file with the appropriate data WITHOUT WHITESPACE (remove \t) named "cleaned_cats.csv".
- Exclude the "body_type" column
- If a row has a length < 5, remove it.
- For strings containing extra quotes, strip the extra quotes.
- Return the list of lists.
Args:
input_file (cats.txt)
Returns:
List of lists
Output:
[['breed', 'type', 'coat_type', 'coat_pattern'],
['Abyssinian', 'Natural', 'Short', 'Ticked tabby'],
['Aegean', 'Natural', 'Semi-long', 'Multi-color'],
['American Bobtail', 'Mutation', 'Semi-long', 'All'],
['American Curl', 'Mutation', 'Semi-long', 'All'],
['American Ringtail', 'Mutation', 'Semi-long', 'All'],
['American Shorthair', 'Natural', 'Short', 'All'],
...
['Ukrainian Levkoy', 'Crossbreed between the Donskoy and Scottish Fold', 'Hairless', 'Solid gray'],
['York Chocolate', 'Natural', 'Long', 'Solid chocolate, solid lilac and solid taupe or any of these colors with white']]
Length:
96
"""
print(txt_to_csv("cats.txt"))
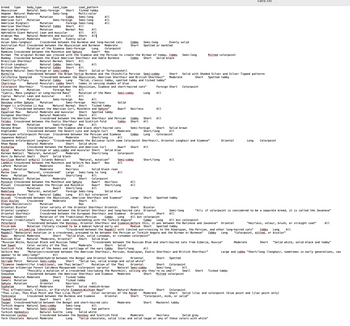

In this question we have to write a python function that reads data from a tab-separated text file, cleans the data, and writes it to a CSV file. The function takes the name of the text file as an input and returns the cleaned data as a list of lists. The code implements the requirements specified in the question, such as excluding the "body_type" column, removing rows with a length less than 5, and stripping extra quotes from strings.
Let's code, hope this helps. You may use threaded question feature to ask your further queries if any.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Modify the code below the #S11icq Q5 comment as you enter code for this task. Modify the code that creates the variable sf to assign the value in index position 2 of the list fish Modify the loop to repeat exactly four times . Click on the Run Current Script function (use menu at top of Thonny). Match the following: 1. 18500967 2. 237233811 updated value of sm 3. 35 updated value of sg_h 4. 44 updated value of sg_c 5. 2 6. 3 6arrow_forwardIn pythonarrow_forwardthis is my code so far but it its not accurate, not sure if i should def function and then for loop? import csv with open ('TopUni.csv', mode='r') as csv_file: csvReader = csv.reader(csv_file) line = [] for line in csvReader: sum(line) print(line)arrow_forward
- Filename: runlength_decoder.py Starter code for data: hw4data.py Download hw4data.py Download hw4data.pyYou must provide a function named decode() that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the…arrow_forward| Which data structure to use? Suppose you need to store a list of elements, if the number of elements in the program is fixed, what data structure should you use? (array, ArrayList, or LinkedList) If you have to add or delete the elements at the beginning of a list, should you use ArrayList or LinkedList? If most of operations on a list involve retrieving an element at a given index, should you use ArrayList or LinkedList?arrow_forwardWhich of the following assignment statements creates a list with 4 Integer elements? Click on the Correct Response A) my list=[7, 2, -8, 16] B) my_list = integer(4) C) my_list = [4] D) my_list = ['1', '2', '3', '4']arrow_forward
- {7,14,26,43,46,50,81} trace the binary search code and fill out the table for 3 different cases: Search for the value that is in the table. Search for the value that is greater than any value in the list. Search for the value that is smaller than any value in the list. low high Low<high mid callarrow_forwardyour", Write a function find value that finds the locations of tamily, propore? that finds the locations rarget value En a list- 12 and shoeef return the list of the location indexes target. my-list d function should return em)arrow_forwardAdd a prompt to ask the user to input their last name (no spaces ; please use underscores if needed), age and a balance of their choice. Create a new entry in the patient_list array with this information [Important note: you can statically modify the code to have the patient_list array hold an extra entry ; no need to do any dynamic memory allocation to accommodate the new entry]. #include <iostream> #include <cstdlib> #include <cstring> using namespace std; // Declaring a new struct to store patient data struct patient { int age; char name[20]; float balance; }; // TODO: // IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY AGE // THE FUNCTION RETURNS AN INTEGER AS FOLLOWS: // -1 IF THE AGE OF THE FIRST PATIENT IS LESS // THAN THE SECOND PATIENT'S AGE // 0 IF THE AGES ARE EQUAL // 1 OTHERWISE // TODO: // IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY BALANCE DUE // THE FUNCTION RETURNS AN INTEGER AS…arrow_forward
- Your goal is to implement an application that reads a file, modify its content, and writes the modification back to the same file. The file includes 2 lines of integers. The first line indicates which integer on the second line has been selected (active). And the second line lists all the integers in the list (maximum of 10). Your application should have a menu that is constantly displayed on the screen (see the menu below). Right below the menu, the program should display list of all integers in the file (second line). Also, it should show which integer is currently selected (active) by displaying an arrow to its left. The user should be able to select a menu item by entering its associated key and pressing the Enter key on the keyboard. “Select Down” selects the next item in the list. If the item at the end of the list is selected, this option selects the first item in the list. “Select Up” selects the previous item in the list. If the first item is selected, this option selects the…arrow_forwardPOEM_LINE = str POEM = List[POEM_LINE] PHONEMES = Tuple[str] PRONUNCIATION_DICT = Dict[str, PHONEMES] def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int], word_to_phonemes: PRONUNCIATION_DICT) -> bool: """Return True if and only if the lines from poem_lines with index in lines_to_check all rhyme, according to word_to_phonemes. Precondition: lines_to_check != [] >>> poem_lines = ['The mouse', 'in my house', 'electric.'] >>> lines_to_check = [0, 1] >>> word_to_phonemes = {'THE': ('DH', 'AH0'), ... 'MOUSE': ('M', 'AW1', 'S'), ... 'IN': ('IH0', 'N'), ... 'MY': ('M', 'AY1'), ... 'HOUSE': ('HH', 'AW1', 'S'), ... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K', ... 'T', 'R', 'IH0', 'K')} >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True…arrow_forwardCreate a .txt file with 3 rows of various movies data of your choice in the following table format: Movie ID number of viewers rating release year Movie name 0000012211 174 8.4 2017 "Star Wars: The Last Jedi" 0000122110 369 7.9 2017 "Thor: Ragnarok" Create a class Movie which instantiates variables corresponds to the table columns. Implement getters, setters, constructors and toString. ----Important 1. Implement two search methods to search Movies by their rating from unsorted array and by year (first) and a rating (second) from unsorted List. Test your methods and explain their time complexity.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
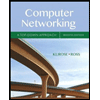
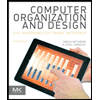
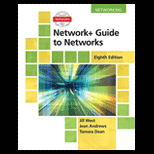
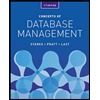
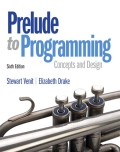
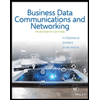