mplement a program that identifies a person based on their DNA. $ python3 dna.py databases/large.csv sequences/5.txt Lavender [https://drive.google.com/drive/folders/1uEjrE0VNWG5HZYcmsMD8ZJUWgeW2whK6?usp=sharing] Download the file that you're going to use for this problem. Extract this file and you will see a directory of sample databases and a directory of sample sequences. Specification In a file called dna.py, implement a program that identifies to whom a sequence of DNA belongs. The program should require as its command-line argument that name of a CSV file containing the STR counts for a list of individuals and should require as its second command-line argument the name of a text file containing the DNA sequence to identify. If your program is executed with the incorrect number of command-line arguments, your program should print an error message of your choice. If the correct number of arguments are provided, you may assume that the first argument is indeed a filename of a valid CSV file, and that the second argument is the filename of a valid text file. Your program should open the CSV file and read its contents into memory. You may assume that the first row of the CSV file will be the column names. The first column will be the word name and the remaining columns will be STR sequences themselves. Your program should open the DNA sequence and read its contents into memory. For each of the STRs (from the first line of the CSV file), your program should compute the longest run of consecutive repeats of the STR in the DNA sequence to identify. If the STR counts match exactly with any of the individuals in the CSV file, your program should print out the name of the matching individual. You may assume that the STR counts will not match more than one individual. If the STR counts do not match exactly with any of the individuals in the CSV file. your program should print "No match". Sample Runs $ python3 dna.py databases/large.csv sequence/5.txt Lavender$ python3 dna.py Usage: python3 dna.py data.csv sequence.txt$ python3 dna.py data.csv Usage: python3 dna.py data.csv sequence.txt Testing You can use the following test cases to test the correctness of your program. $ python3 dna.py databases/small.csv sequences/1.txt Bob $ python3 dna.py databases/small.csv sequences/2.txt No match $ python3 dna.py databases/small.csv sequences/3.txt No match $ python3 dna.py databases/small.csv sequences/4.txt Alice $ python3 dna.py databases/large.csv sequences/5.txt Lavender
Implement a
$ python3 dna.py
[https://drive.google.com/drive/folders/1uEjrE0VNWG5HZYcmsMD8ZJUWgeW2whK6?usp=sharing]
Download the file that you're going to use for this problem. Extract this file and you will see a directory of sample databases and a directory of sample sequences.
Specification
In a file called dna.py, implement a program that identifies to whom a sequence of DNA belongs.
- The program should require as its command-line argument that name of a CSV file containing the STR counts for a list of individuals and should require as its second command-line argument the name of a text file containing the DNA sequence to identify.
- If your program is executed with the incorrect number of command-line arguments, your program should print an error message of your choice.
- If the correct number of arguments are provided, you may assume that the first argument is indeed a filename of a valid CSV file, and that the second argument is the filename of a valid text file.
- Your program should open the CSV file and read its contents into memory.
- You may assume that the first row of the CSV file will be the column names.
- The first column will be the word name and the remaining columns will be STR sequences themselves.
- Your program should open the DNA sequence and read its contents into memory.
- For each of the STRs (from the first line of the CSV file), your program should compute the longest run of consecutive repeats of the STR in the DNA sequence to identify.
- If the STR counts match exactly with any of the individuals in the CSV file, your program should print out the name of the matching individual.
- You may assume that the STR counts will not match more than one individual.
- If the STR counts do not match exactly with any of the individuals in the CSV file. your program should print "No match".
Sample Runs
$ python3 dna.py databases/large.csv sequence/5.txt Lavender$ python3 dna.py Usage: python3 dna.py data.csv sequence.txt$ python3 dna.py data.csv Usage: python3 dna.py data.csv sequence.txt
Testing
You can use the following test cases to test the correctness of your program.
$ python3 dna.py databases/small.csv sequences/1.txt Bob
$ python3 dna.py databases/small.csv sequences/2.txt No match
$ python3 dna.py databases/small.csv sequences/3.txt No match
$ python3 dna.py databases/small.csv sequences/4.txt Alice
$ python3 dna.py databases/large.csv sequences/5.txt Lavender



Step by step
Solved in 2 steps

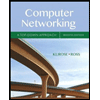
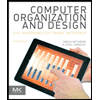
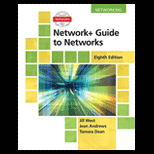
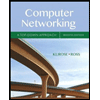
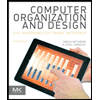
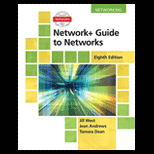
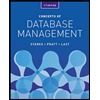
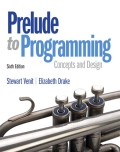
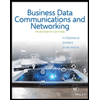