Create a new file in c++. In this lab, you will add some functionality to the table ofmenu. Your boss (me) has decided that letters should be used for menu items instead of digits (because there are more alpha characters than numeric ones, so more additional menu items could be added in the future). We all know that programmers are lazy, but so are most users! So, the user should be able to enter either uppercase (A) or lowercase (a) for their menu selection. Also, it is important to let the user know if they entered an invalid choice. Write a program that displays the following menu and prompts the user for a selection. Once the selection has been made, display the selection back to the user. Then, ask the user for two numbers and perform the selected operation (+, -, *, /). Display the results back to the user (e.g. 2 + 3 = 5). When dividing, display an error message if the second number is 0. A) Add two numbers B) Subtract two numbers C) Multiply two numbers D) Divide two numbers X) Exit program The program should: display a "hello" message before presenting the menu display the menu prompt user for selection - accept either uppercase or lowercase for selection - display error message if invalid selection is made (example: E) display the selection back to the user prompt for two numbers perform the operation and display the results display error message for division when second number is 0 display a "goodbye" message before exiting the program HINT: The switch statement is ideal for menu-driven programs... SAMPLE OUTPUT FOR VALID MENU SELECTION You entered b, subtract two numbers SAMPLE OUTPUT FOR INVALID MENU SELECTION You entered E, an invalid selection SAMPLE OUTPUT FOR ADD Please enter two numbers, separated by a space: 2 3 2 + 3 = 5 SAMPLE OUTPUT FOR DIVISION ERROR Please enter two numbers, separated by a space: 2 0 Division by zero is not possible Submit the completed CPP file (not the EXE file) back to this assignment.
Create a new file in c++.
In this lab, you will add some functionality to the table ofmenu. Your boss (me) has decided that letters should be used for menu items instead of digits (because there are more alpha characters than numeric ones, so more additional menu items could be added in the future). We all know that programmers are lazy, but so are most users! So, the user should be able to enter either uppercase (A) or lowercase (a) for their menu selection. Also, it is important to let the user know if they entered an invalid choice.
Write a
A) Add two numbers B) Subtract two numbers C) Multiply two numbers D) Divide two numbers X) Exit program
The program should:
- display a "hello" message before presenting the menu
- display the menu
- prompt user for selection
- - accept either uppercase or lowercase for selection
- - display error message if invalid selection is made (example: E)
- display the selection back to the user
- prompt for two numbers
- perform the operation and display the results
- display error message for division when second number is 0
- display a "goodbye" message before exiting the program
HINT: The switch statement is ideal for menu-driven programs...
SAMPLE OUTPUT FOR VALID MENU SELECTION You entered b, subtract two numbers
SAMPLE OUTPUT FOR INVALID MENU SELECTION You entered E, an invalid selection
SAMPLE OUTPUT FOR ADD Please enter two numbers, separated by a space: 2 3 2 + 3 = 5
SAMPLE OUTPUT FOR DIVISION ERROR Please enter two numbers, separated by a space: 2 0 Division by zero is not possible
Submit the completed CPP file (not the EXE file) back to this assignment.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

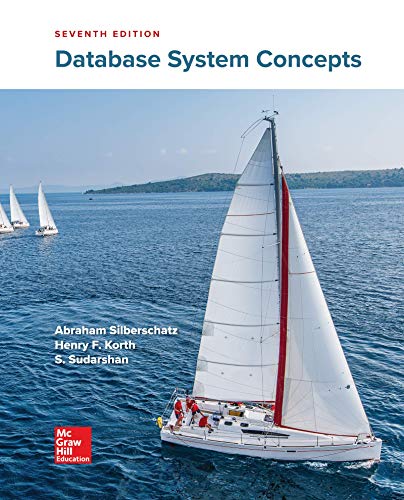
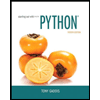
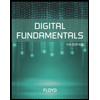
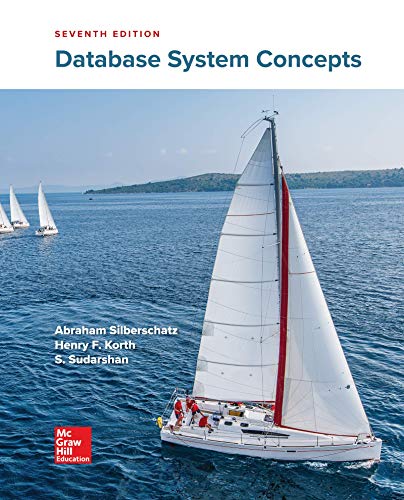
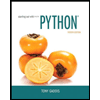
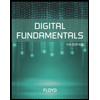
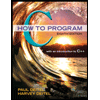
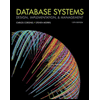
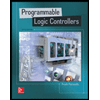