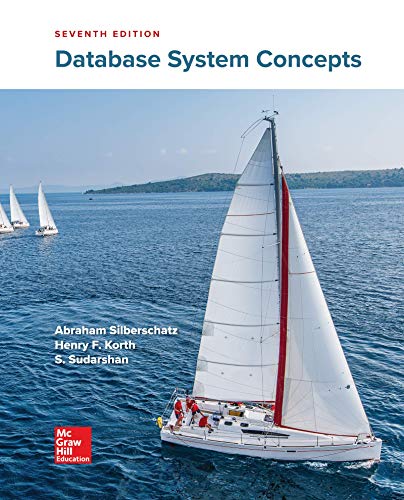
*Please use PYTHON to write a code for this problem*
Your task is to find all social security numbers listed within a particular file. You are given a file (info.txt) which contains text including some social security numbers. You have to read the file and find all social security numbers within it and output those numbers to a new file (ssn.txt).
All social security numbers are of the form XXX-XX-XXXX where X is a digit between 0 and 9 inclusive. The outputted social security numbers should be on separate lines.
Note: You may assume Info.txt is in the same folder as your python py file. You should create the required output file in the same folder.
Keep in mind, to test this program you will have to create your own Info.txt and save it in the same folder as your python py file.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- The function print_last_line in python takes one parameter, fname, the name of a text file. The function should open the file for reading, read the lines of the file until it reaches the end, print out the last line in the file, report the number of lines contained in the file, and close the file. Hint: Use a for loop to iterate over the lines and accumulate the count. For example: Test Result print_last_line("wordlist1.txt") maudlin The file has 5 lines.arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a `map' of all the underground resources in the mine. This map comes as a file. The file has n rows. Each row has n space-separated integers. Each integer is between zero and one hundred (inclusive). The file should be understood to represent a grid, n wide by n deep. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and n. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on.…arrow_forwardThis Python Lab 9 Lab: Write a file copying program. The program asks for the name of the file to copy from (source file) and the name of the file to copy to (destination file). The program opens the source file for reading and the destination file for writing. As the program reads each line from the source file and it writes the line to the destination file. When every line from the source file has been written to the destination file, it close both files and print “Copy is successful.” In the sample run, “add.py” is the source file and “add-copy.py” is the destination file. Note that both “add-copy.py” is identical to “add.py” because “add-copy.py” is a copy of “add.py”. Sample run: Enter file to copy from: add.py Enter file to copy to : add-copy.py Copy is successful. Source file: add.py print("This program adds two numbers") a = int(input("Enter first number: ")) b = int(input("Enter second number: ")) print(f"{a} + {b} = {a+b}") Destination file:…arrow_forward
- Make the following modifications for the solution above: name the output/input file "myRandomNumbers.txt" use a loop to output 17 random numbers to the file after creating the file, close it open the file for input and read the contents and then total the numbers in the file determine the average of the numbers in the file determine the highest and lowest numbers in the file DO NOT USE PYTHON BUILTIN FUNCTIONS TO CALCULATE THE AVERAGE OR THE HIGHEST AND LOWEST NUMBERS an expected number of modules/functions would be in the 5-8 range Display your results: the numbers generated on a single horizontal line the calculated total and average the highest and lowest number in the file Be sure to use clear prompts/labeling for input and output.arrow_forwardPython programming language Write a program that asks the user for a number. Write the number to a file called total.txt. Then ask the user for a second number. Write the new number on line 2 with the total for both numbers next to it separated by a comma. Then ask the user for a third number and do the same. Keep asking for numbers until the person puts in a zero to terminate the program. Close the file. Be sure to use at least 2 functions [main() and total_numbers(number, total)]. Put all inputs and outputs into the main function, with all calculations into the total_numbers function. Give me a number: 13 Give me another number: 14 14 + 13 = 27 Give me another number: 3 3 + 27 = 30 Give me another number: 4 4 + 30 = 34 Give me another number (0 for quit): 0 Your total is 34. Write a program that displays the lines from the total.txt file in the following output. Use a try catch phrase to check for errors. Use only one function for this portion [main()]. 13 14, 27 3,…arrow_forwardComputer Science C++ Create a text file "input.txt" with a certain amount of integers (you decide how many). Write a program that reads these numbers from the file, adds them, and when you have reached the end of the file, calculates the average of these numbers. Print a message and the average to the console. Code this program twice, demonstrating the two methods to detect the end of the file, part A: reading a value from Instream and storing it (boolean expression) in the while loop part B: using the eof() member functionarrow_forward
- can you do it pythonarrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardIn this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forward
- JAVA PPROGRAM Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found. While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. The file may contain up to 100 names. You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList. Input validation: a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…arrow_forward<<Write in Java>> - Challenge 7 File encryption is the science of writing the contents of a file in a secret code. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded contents out to a second file. The second file will be a version of the first file, but written in a secret code. Although there are complex encryption techniques, you should come up with a simple one of your own. For example, you could read the first file one character at a time, and add 10 to the character code of each character before it is written to the second file. - Challenge 8 Write a program that decrypts the file produced by the program in Programming Challenge 7. The decryption program should read the contents of the coded file, restore the data to its original state, and write it to another filearrow_forwardUsing Java PrintWriter, write a program that prompts the user for information and saves it to a text file File name is musicians.txt Use this information below: George,Thoroughgood,54321 Ludwig,Van Beethoven,90111 Edward,Van Halen,12345arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
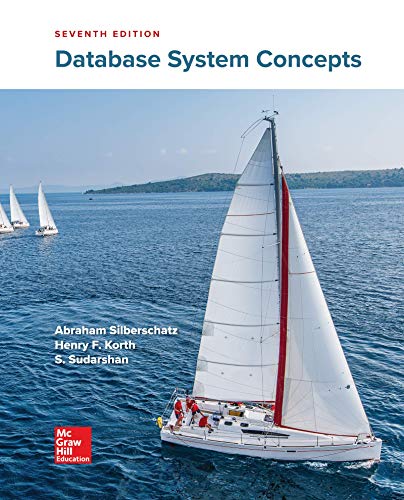
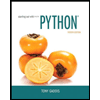
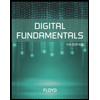
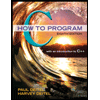
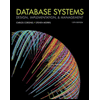
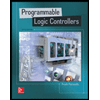