Language: Python Write a function named factorial(x) that computes the factorial of a number. Include a docstring. Make sure you throw an error (such as ValueError) if x is negative or not an integer. You must implement this yourself (you can't use math.factorial). You can use the following procedure for the factorial: Set the result to 1 Loop over the values 1 to x, multiplying the result each time Return the result Here (as in later problems), I'll put ... where you should write code. Fill in the following: -> # write a function that calculates the factorial of an integer def factorial(x):... # !Check for input is integer or not, must be integer # print something that says integer or not # do calculations and return final result (several lines) result = 1 # use for-loop to calculate the factorial given x and return result ... # your input (you may use input command to ask user input) x = 10 factorial(x)
Language: Python Write a function named factorial(x) that computes the factorial of a number. Include a docstring. Make sure you throw an error (such as ValueError) if x is negative or not an integer. You must implement this yourself (you can't use math.factorial). You can use the following procedure for the factorial: Set the result to 1 Loop over the values 1 to x, multiplying the result each time Return the result Here (as in later problems), I'll put ... where you should write code. Fill in the following: -> # write a function that calculates the factorial of an integer def factorial(x):... # !Check for input is integer or not, must be integer # print something that says integer or not # do calculations and return final result (several lines) result = 1 # use for-loop to calculate the factorial given x and return result ... # your input (you may use input command to ask user input) x = 10 factorial(x)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Language: Python
Write a function named factorial(x) that computes the factorial of a number. Include a docstring. Make sure you throw an error (such as ValueError) if x is negative or not an integer. You must implement this yourself (you can't use math.factorial). You can use the following procedure for the factorial:
- Set the result to 1
- Loop over the values 1 to x, multiplying the result each time
- Return the result
Here (as in later problems), I'll put ... where you should write code.
Fill in the following:
->
# write a function that calculates the factorial of an integer
def factorial(x):...
# !Check for input is integer or not, must be integer
# print something that says integer or not
# do calculations and return final result (several lines)
result = 1
# use for-loop to calculate the factorial given x and return result
...
# your input (you may use input command to ask user input)
x = 10
factorial(x)
![Problem 1: Getting started
Write a function named factorial(x) that computes the factorial of a number. Include a docstring. Make sure you throw an error (such as
ValueError) if x is negative or not an integer. You must implement this yourself (you can't use math.factorial). You can use the following
procedure for the factorial:
Set the result to 1
• Loop over the values 1 to x, multiplying the result each time
Return the result
Here (as in later problems), I'll put ... where you should write code.
[8] # write a function that calculates the factorial of an integer
def factorial(x):...
# !Check for input is integer or not, must be integer
#print something that says integer or not
# do calculations and return final result (several lines)
result = 1
# use for-loop to calculate the factorial given x and return result
# your input (you may use input command to ask user input)
X = 10
factorial(x)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdfbbf43f-b5c2-4d87-8106-243aab91da52%2F1c0b4e70-26cc-442f-b67e-dd805fb40806%2Fmxc20m_processed.png&w=3840&q=75)
Transcribed Image Text:Problem 1: Getting started
Write a function named factorial(x) that computes the factorial of a number. Include a docstring. Make sure you throw an error (such as
ValueError) if x is negative or not an integer. You must implement this yourself (you can't use math.factorial). You can use the following
procedure for the factorial:
Set the result to 1
• Loop over the values 1 to x, multiplying the result each time
Return the result
Here (as in later problems), I'll put ... where you should write code.
[8] # write a function that calculates the factorial of an integer
def factorial(x):...
# !Check for input is integer or not, must be integer
#print something that says integer or not
# do calculations and return final result (several lines)
result = 1
# use for-loop to calculate the factorial given x and return result
# your input (you may use input command to ask user input)
X = 10
factorial(x)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
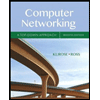
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
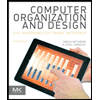
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
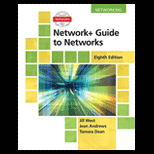
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
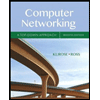
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
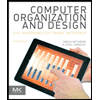
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
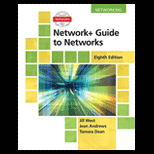
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
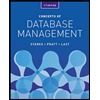
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
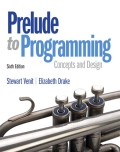
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
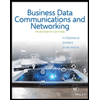
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY