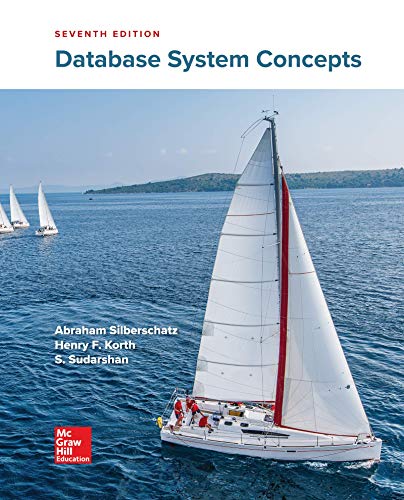
Concept explainers
C++ Programming It should align exactly like the screenshot, sample output.
The local Driver’s License Office has asked you to write a program that grades the written portion of the driver’s license exam. The exam has 20 multiple choice questions. Here are the correct answers:
1. A
2. D
3. B
4. B
5. C
6. B
7. A
8. B
9. C
10.D
11. A
12. C
13. D
14. B
15. D
16. C
17. C
18. A
19. D
20. B
Your program should store the correct answers shown above in an array. It should ask the user to enter the student’s answers for each of the 20 questions, and the answers should be stored in another array. After the student’s answers have been entered, the program should display a message indicating whether the student passed or failed the exam. (A student must correctly answer 15 of the 20 questions to pass the exam.) It should then display the total number of correctly answered questions, the total number of incorrectly answered questions, and a list showing the question numbers of the incorrectly answered questions.
Input Validation: Only accept the letters A, B, C, or D as answers.
You are to have 3 functions for this assignment:
void readAnswers(char []) – Takes an array and fills it with the
students answers. This function should validate the student
answer.
int gradeExam(char [], char []) – Compares answers and returns
the number of correct answers.
void printResults(int, char [], char []) – Prints whether the
student passed the exam or not, also the amount correct and not
correct and then prints out all answers and correct answers (see
below)
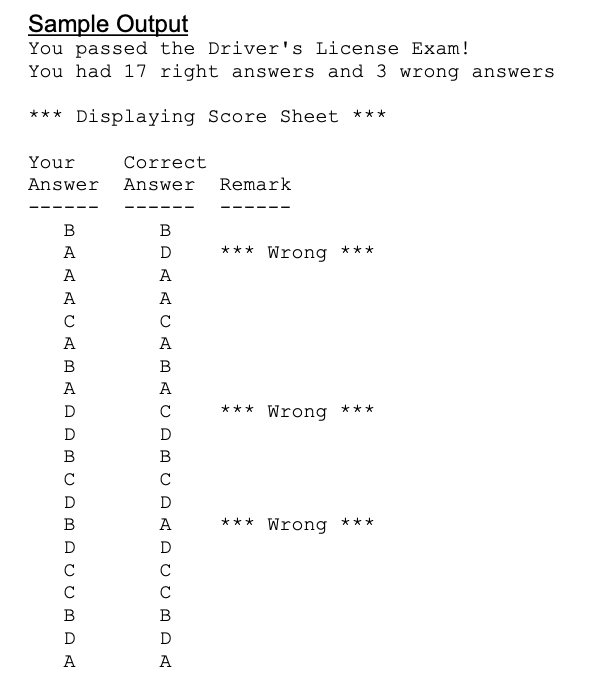
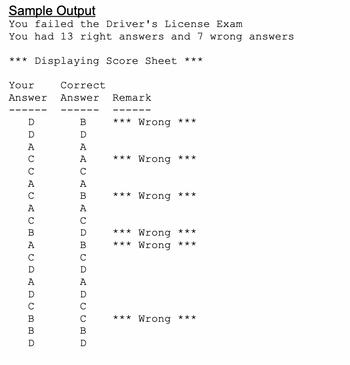

#include
Step by stepSolved in 3 steps with 3 images

- Validating User Input Summary In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops…arrow_forwardIn C++ please, using basic programming one coding The main menu should show the available options: Show the roster: Displays all the player names and jersey numbers in a numbered list. Ex: 1. Susan Martinez (33), 2. Jake Smith (21), etc. Add a player: Prompts the user to type the player's full name and jersey number. Then adds the player to the roster. Remove a player: Displays the roster and prompts the user to enter the number of the player to remove. Then removes the player from the roster. Quit: Quits the program. The program should display the main menu after the user shows the roster, adds a player, or removes a player. The program only terminates when the user chooses the quit option. Data validation should be used where appropriate. Ex: The user should not be able to choose an option that doesn't exist or delete a player that doesn't exist. The roster should be kept in alphabetic order by the players' full names. Ex: If Alice Chang (9) is added to a roster with Jake Smith and…arrow_forwardPart 1: Complete the survey. Part 2: Peek-a-boo is a fun game that little kids like to play. To simulate this game on the computer, write a program that will generate a random number between 1 and 4. Then, will print to the screen the animal name associated to that number. The animal names used will be: pig when a 1 is generated cow when a 2 is generated chicken when a 3 is generated horse when a 4 is generated If your program generates a 3, the output will be: chicken The player will then enter a 1 if they would like to play again or anything else to exit the program. If the player enters "1 1 1 0", the output will be: horse chicken cow horse For coding simplicity, follow each output animal by a space, even the last one. Hint: To make testing easier, seed your random number generator with 0.arrow_forward
- Question 2: Write a program to check responses of a student to the multiple-choice final exam. The final exam consists of 20 questions, and answer sheet is abedeabcdeabedeabcde. Your screen should look like: Please, write your answers: abbdeabbdeabbdeabbde Please, write your answers: or aaaaaaaaaaaaaaaaaaaa Your score is 16 out of 20. Your score is 4 out of 20.arrow_forwardC++ continued you already gave me the help from the first part. now they have added another set of instructions. original code used. Explanation Here I have created the main method. Inside the main method, I have taken input from the user for height and width and stored them into different variables. Next, I have created a loop that runs till it reaches the height of the rectangle. Inside the loop, I have created another loop that runs till the width of the rectangle. In the inner loop, I have printed the asterisk symbol. After the inner loop, I have used endl to give break and move the pointer to the new line. arrow_forward Answer C++ code: #include<iostream>using namespace std; int main(){ int height, width; // user input cin >> height >> width; // outer loop for(int i = 0; i < height; i++) { // inner loop for(int j = 0; j < width; j++) { //printing the * symbol cout << "*"; }…arrow_forwardUsing a Sentinel Value to Control a while Loop in Java Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the…arrow_forward
- Microwaves / Radio Waves If a scientist knows the wavelength of an electromagnetic wave she can determine what type of radiation it is. Write a program that asks for the wavelength in meters of an electromagnetic wave and then displays what that wave is according to the following chart. (For example, a wave with a wavelength of 1E-10 meters would be an X-ray.) 1x 10-11 1 x 10-8 4 x 10-7 7x 10-7 1x 10-3 1 x 10-2 Gamma Rays. X Rays Ultraviolet Visible Light Infraredarrow_forwardIn cengage mindtap The credit plan at TidBit Computer Store specifies a 10% down payment and an annual interest rate of 12%. Monthly payments are 5% of the listed purchase price, minus the down payment. Write a program that takes the purchase price as input. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance × rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed.arrow_forwardIn C++ Programming, write a program that calculates and prints the monthly paycheck for an employee. The net pay is calculated after taking the following deductions: Federal Income Tax: 15% State Tax: 3.5% Social Security Tax: 5.75% Medicare/Medicaid Tax: 2.75% Pension Plan: 5% Health Insurance: $75.00 Your program should prompt the user to input the gross amount and the employee name. The output will be stored in a file. Format your output to have two decimal places. A sample output follows: Bill Robinson Gross Amount: .......................... $3575.00 Federal Tax: ................................ $ 536.25 State Tax: .....................................$ 125.13 Social Security Tax: ................... $ 205.56 Medicare/Medicaid Tax: ......... $ 98.31 Pension Plan: .............................. $ 178.75 Health Insurance: .......................$ 75.00 Net Pay: ....................................... $2356.00 NOTE: The first column is left-justified, and the right column is…arrow_forward
- This example creates a program to teach a first grade child how to learn subtractions. The program randomly generates two single- digit integers number1 and number2 with number1 > number2 and displays a question such as "What is 9 – 2?" to the student. After the student types the answer, the program displays a message to indicate whether the answer is correct.arrow_forwardPython please: If the score is between 90 and 100, the grade is A, and the teacher comment is 'Exceptional work' for 100 only 'Excellent work' for anything other than 100 If the score is between 80 and 89, the grade is B, and the teacher comment is 'Very Good, can do better' If the score is between 70 and 79, the grade is C, and the teacher comment is 'Good, but need to work harder' If the score is between 60 and 69, the grade is D, and there are no teacher comments. If the score is below 60, the grade is F, and the teacher comment is 'Fail, need to appear for a retest' For example if the input (student test score) is 100 the output is The Student grade is A and the teacher comment is 'Exceptional work'arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
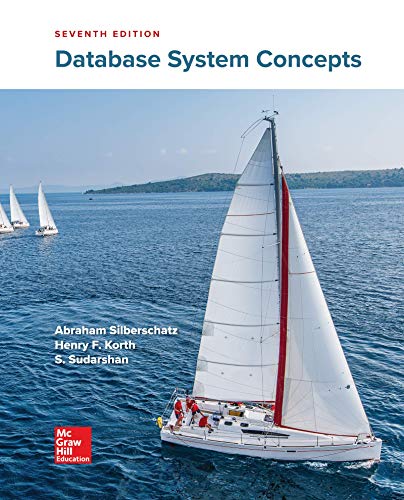
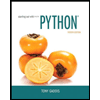
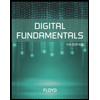
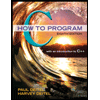
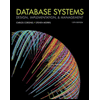
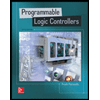