Case Diagram and UML Class Diagram for this java code : (Below the code give some constructive critiques about the UML class diagram)…. Thanks import java.util.*; public class BeverlyBreads{public static void main(String[] args){Scanner input = new Scanner(System.in); //Array for breads String[] breads = new String[5]; //Bread choices breads[0] = "White Bread"; breads[1] = "Wheat Bread"; breads[2] = "Rye Bread"; breads[3] = "Pumpernickel Bread"; breads[4] = "Baguette"; //Prices for the breads double[] prices = new double[5]; prices[0] = 2.99; prices[1] = 3.49; prices[2] = 3.99; prices[3] = 4.49; prices[4] = 4.99; //Customer's Name System.out.println("Enter customer's name: "); String name = input.nextLine(); //Customer's Phone Number System.out.println("Enter customer's phone number: "); String phoneNumber = input.nextLine(); //Bread Selection System.out.println("Which bread would you like to order?"); for(int i = 0; i < breads.length; i++) { System.out.println(breads[i] + " " + prices[i]); } int breadSelection = input.nextInt(); switch(breadSelection) { case 1: System.out.println("You've selected " + breads[0] + " " + prices[0]); break; case 2: System.out.println("You've selected " + breads[1] + " " + prices[1]); break; case 3: System.out.println("You've selected " + breads[2] + " " + prices[2]); break; case 4: System.out.println("You've selected " + breads[3] + " " + prices[3]); break; case 5: System.out.println("You've selected " + breads[4] + " " + prices[4]); break; default: System.out.println("You've made an invalid selection"); break; } //Confirmation Page System.out.println("Name: " + name); System.out.println("Phone Number: " + phoneNumber); System.out.println("Bread Selection: " + breads[breadSelection - 1]); System.out.println("Price: " + prices[breadSelection - 1]); } }
Case Diagram and UML Class Diagram for this java code : (Below the code give some constructive critiques about the UML class diagram)…. Thanks
import java.util.*; public class BeverlyBreads{public static void main(String[] args){Scanner input = new Scanner(System.in); //Array for breads String[] breads = new String[5];
//Bread choices breads[0] = "White Bread"; breads[1] = "Wheat Bread"; breads[2] = "Rye Bread"; breads[3] = "Pumpernickel Bread"; breads[4] = "Baguette";
//Prices for the breads double[] prices = new double[5]; prices[0] = 2.99; prices[1] = 3.49; prices[2] = 3.99; prices[3] = 4.49; prices[4] = 4.99;
//Customer's Name System.out.println("Enter customer's name: "); String name = input.nextLine(); //Customer's Phone Number System.out.println("Enter customer's phone number: "); String phoneNumber = input.nextLine(); //Bread Selection System.out.println("Which bread would you like to order?"); for(int i = 0; i < breads.length; i++) { System.out.println(breads[i] + " " + prices[i]); } int breadSelection = input.nextInt(); switch(breadSelection) { case 1: System.out.println("You've selected " + breads[0] + " " + prices[0]); break; case 2: System.out.println("You've selected " + breads[1] + " " + prices[1]); break; case 3: System.out.println("You've selected " + breads[2] + " " + prices[2]); break; case 4: System.out.println("You've selected " + breads[3] + " " + prices[3]); break; case 5: System.out.println("You've selected " + breads[4] + " " + prices[4]); break; default: System.out.println("You've made an invalid selection"); break; } //Confirmation Page System.out.println("Name: " + name); System.out.println("Phone Number: " + phoneNumber); System.out.println("Bread Selection: " + breads[breadSelection - 1]); System.out.println("Price: " + prices[breadSelection - 1]); } }

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

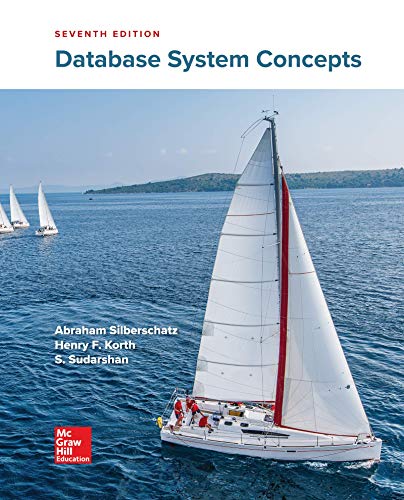
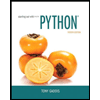
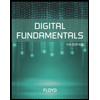
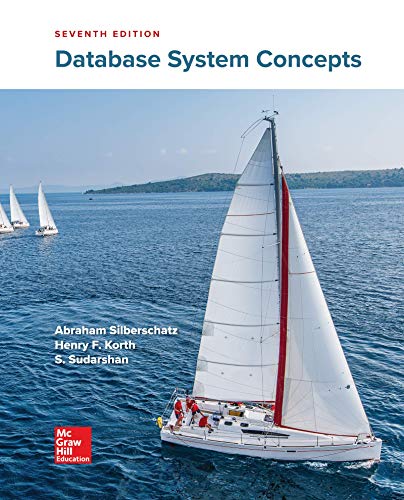
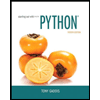
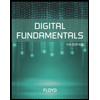
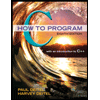
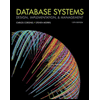
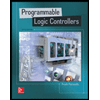