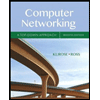
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
#include
#include
#include
// input: file with integer on each line
// output: sum of numbers
int main()
{
// data.txt must be in same directory as program
char path[256] = "data.txt";
std::ifstream file(path);
if (!file.is_open())
{
std::cout << "ERROR: the file " << path << " did not open" << std::endl;
return -1;
}
// buffer to hold single line from file, up to some maximum number of characters
const int max_length = 1024;
char buffer[max_length] = {};
int sum = 0;
while (file.getline(buffer, max_length - 1))
{
// read "stoi" as "s-to-i" or "string to integer"
int number = std::stoi(buffer); // convert c-string to integer
sum += number;
}
std::cout << "sum = " << sum << std::endl;
return 0;
}
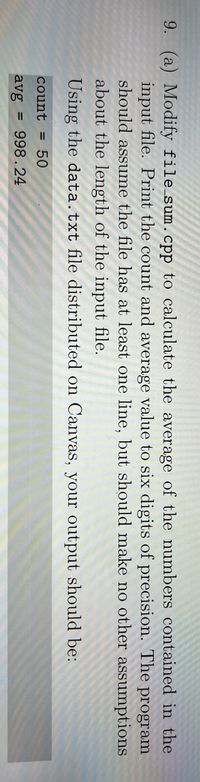
Transcribed Image Text:9. (a) Modify file sum.cpp to calculate the average of the numbers cotained in the
input file. Print the count and average value to six digits of precision. The
should assume the file has at least one line, but should make no other assumptions
about the length of the input file.
Using the data.txt file distributed on Canvas, your output should be:
program
count = 50
avg = 998.24
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- #include #include #include #include #include #include void dft(uint8_t*, double*, int); void dft print(double, int, double); #define N 512 int main(int argc, char **argv) { char *port = argv[1]; /* Open the file */ int fd = open(port, O_RDONLY); // code to set communication rate to 9600 bits per second struct termios tio; tcgetattr(fd, &tio); cfset speed(&tio, B9600); tcsetattr(fd, 0, &tio); // reopen the serial port so that the speed change takes effect close(fd); fd = open(port, O_RDONLY); if (fd #include #include #include void dft(uint8_t* x, double* X, int N) { double Xr[N]; double Xi[N]; for (int k=0; k 70) count = 70; printf("%031d", Irint (bounds[k]*fs/N)); for (int j=0; j < count; j++) { } } printf("*"); printf("\n"); for (int k=0; k < NBUCKETS; k++) { printf("\033[A"); } }arrow_forwardIn C++ Create a program that outputs 10 strings (that the user inputs) to a file.arrow_forwardflowchrt for the code below //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forward
- Please use C++ code and only use the libraries <iostream> and <fstream>. Thank you!arrow_forwardCreate a program that asks the user for the name of a file and then rearranges the contents of the file so that its lines are in alphabetical order. The program should modify the file, not just print its contents to the screen. Use the STL algorithm sort. C++arrow_forwardC++ Write a code fragment to: declare two file streams, infile and outfile Open them and connect them to the files file1.dat and file2.dat read an int from file1.dat and print it to file2.dat This should only be a half dozen lines of code.arrow_forward
- Using these files BinaryFileRead.cpp // BinaryFileRead.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include <iostream> using namespace std; int main() { int *arrayToSort; char fileName[50]; int size,readVal; cout << "Enter a filename to sort => "; cin >> fileName; FILE *inFile; fopen_s(&inFile,fileName, "rb"); fread(&size, sizeof(size), 1, inFile); arrayToSort = new int[size]; for (int i = 0; i < size; i++) { fread(&readVal, sizeof(readVal), 1, inFile); arrayToSort[i] = readVal; } fclose(inFile); return 0; } Timing.cpp // Timing.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include <iostream> #include <time.h> //ctime #include <sys/timeb.h> //_timeb _ftime_s using namespace std; int main() { struct _timeb timebuffer; char timeline[26]; _ftime_s(&timebuffer); ctime_s(timeline,sizeof(timeline), &(timebuffer.time)); printf("The time is %.19s.%hu %s",…arrow_forward9b_act2. Please help me answer this in python programming.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
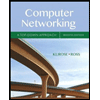
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
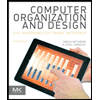
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
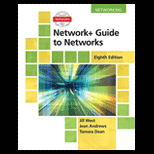
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
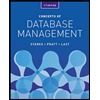
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
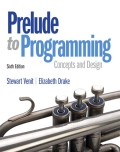
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
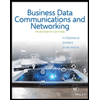
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY