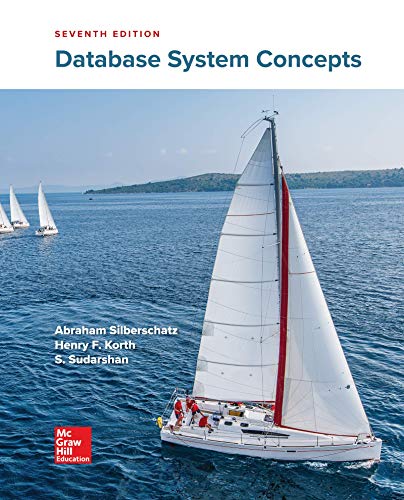
Using these files
BinaryFileRead.cpp
// BinaryFileRead.cpp : Defines the entry point for the console application. //
#include "stdafx.h"
#include <iostream>
using namespace std;
int main()
{
int *arrayToSort;
char fileName[50];
int size,readVal;
cout << "Enter a filename to sort => ";
cin >> fileName;
FILE *inFile;
fopen_s(&inFile,fileName, "rb");
fread(&size, sizeof(size), 1, inFile);
arrayToSort = new int[size];
for (int i = 0; i < size; i++) {
fread(&readVal, sizeof(readVal), 1, inFile);
arrayToSort[i] = readVal;
}
fclose(inFile);
return 0;
}
Timing.cpp
// Timing.cpp : Defines the entry point for the console application. //
#include "stdafx.h"
#include <iostream>
#include <time.h> //ctime
#include <sys/timeb.h> //_timeb _ftime_s
using namespace std;
int main()
{
struct _timeb timebuffer;
char timeline[26];
_ftime_s(&timebuffer);
ctime_s(timeline,sizeof(timeline), &(timebuffer.time));
printf("The time is %.19s.%hu %s", timeline, timebuffer.millitm, &timeline[20]);
system("pause");
return 0;
}
Insertion Sort:

C++ program that allows the user to sort data from a binary file using Bubble Sort, Selection Sort, Insertion Sort, and Shell Sort. It also measures and prints the time before and after sorting and allows the user to specify a lower and upper bound for displaying a portion of the sorted data.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- QUESTION 6 Write the query that set sell to true to for all documents with a milk value greater than 5arrow_forward#include <bits/stdc++.h>using namespace std;int main() { double matrix[4][3]={{2.5,3.2,6.0},{5.5, 7.5, 12.6},{11.25, 16.85, 13.45},{8.75, 35.65, 19.45}}; cout<<"Input no in first row of matrix"<<endl; for(int i=0;i<3;i++){ double t; cin>>t; matrix[0][i]=t; } cout<<"Contents of the last column in matrix"<<endl; for(int i=0;i<4;i++){ cout<<matrix[i][2]<<" "; } cout<<"Content of first row and last column element in matrix is: "<<matrix[0][3]<<endl; matrix[3][2]=13.6; cout<<"Updated matrix is :"<<endl; for(int i=0;i<4;i++){ for(int j=0;j<3;j++){ cout<<matrix[i][j]<<" "; }cout<<endl; } return 0;} Please explain this codearrow_forwardInstructions: You are strictly not allowed to use anything other than pointers and dynamic memory. One function should perform one functionality only. Task 1 Write a program in C++ that reads data from a file. Create dynamic memory according to the data. Now your task is to perform the following task. Row wise Sum Column wise Sum Diagonal wise Sum Example data.txt 4 5 1.6 10.2 33.7 99 20.5 3 44 50 96.1 2 8 9 4 74 50 99 19.1 Output: Sum row wise: 165, 191, 17, 242.1 Sum col wise: 127.6, 120.1, 228.8, 118.1, 20.5 Sum diagonal wise: Not Possible Note: You are restricted to use pointers and your function should be generic. Avoid memory wastage, memory leakage, dangling pointer. Use regrow or shrink concepts if required.arrow_forward
- initial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forwardThe template shown below must be incorporated into the code showed after the template Template: // -- brief statement as to the file’s purpose //XXX XXX- ADD YOUR SECTION NUMBER //Include statements #include #include using namespace std; //Global declarations: Constants and type definitions only -- no variables //Function prototypes int main() { //In cout statement below SUBSTITUTE your name and lab number cout << "Your name -- Lab Number" << endl << endl; //Variable declarations //Program logic //Closing program statements system("pause"); return 0; } //Function definitions Code: #include<iostream>#include <iomanip> using namespace std; //function prototypesfloat convToFeet(float, float, float); //main functionint main() {// variable declarationchar woodType;float totalCharge = 0.0, eachCost = 0.0, woodWidth, woodHeight, woodLength;;int noOfpc;cout << setprecision(2) << fixed; //infinite loop until it obtains the character 'T'do{cout…arrow_forwardC++ I cannot run the code. Please help me fix it. Below is the requirement of the code. Design and write a C++ class that reads text, binary and csv files. The class functions: Size: Returns the file sizeName: Returns the file nameRaw: Returns the unparsed raw data (use vector here to get the unparsed raw data)Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse. With binary files, need to use RegEx to do the parse(). [My code doesn't have this part] Please refer to the Callback.cpp example on how to setup a Call-Back. CallBack.cpp: #include <string>#include <functional>#include <iostream>#include <vector>using namespace std; string ToLower(string s){ string temp; for (char c : s)…arrow_forward
- In C++ Write a code snippet to automatically increase the size of the dynamic array playerToolbox[10] if it becomes full while reading data in from a file.arrow_forwardAll files are included below, seperated by the dashes. "----" //driver.cpp #include <iostream> #include <string> #include "stackLL.h" #include "queueLL.h" #include "priorityQueueLL.h" using namespace std; int main() { /////////////Test code for stack /////////////// stackLLstk; stk.push(5); stk.push(13); stk.push(7); stk.push(3); stk.push(2); stk.push(11); cout<<"Popping: "<<stk.pop() <<endl; cout<<"Popping: "<<stk.pop() <<endl; stk.push(17); stk.push(19); stk.push(23); while( ! stk.empty() ) { cout<<"Popping: "<<stk.pop() <<endl; } // output order: 11,2,23,19,17,3,7,13,5 stackLLstkx; stkx.push(5); stkx.push(10); stkx.push(15); stkx.push(20); stkx.push(25); stkx.push(30); stkx.insertAt(-100, 3); stkx.insertAt(-200, 7); stkx.insertAt(-300, 0); //output order: -300,30,25,20,-100,15,10,5,-200 while( ! stkx.empty() ) cout<<"Popping: "<<stkx.pop() <<endl; ///////////////////////////////////////…arrow_forwardCode below will not create the file needed for the descrambling word game below: Help me create the file needed to complete the game in C++ and make the game timed. #include <iostream>#include <algorithm>#include <fstream>#include <string> using namespace std; string sortString(string word){ transform(word.begin(), word.end(), word.begin(), ::toupper); sort(word.begin(), word.end()); return word;} void jumbledString(string jumble){ string checkPerWord = ""; string userEnteredAfterSorting; userEnteredAfterSorting = sortString(jumble); ifstream word("Game.txt"); if (word) { while (getline(word, checkPerWord)) { string Ch = sortString(checkPerWord); if (Ch == userEnteredAfterSorting) { cout << checkPerWord << endl; } } word.close(); }} int main(){ string string = "tac"; jumbledString(string); return 0;}int main1(){ string string =…arrow_forward
- Please answer in C++arrow_forwardstruct employee{int ID;char name[30];int age;float salary;}; (A) Using the given structure, Help me with a C program that asks for ten employees’ name, ID, age and salary from the user. Then, it writes the data in a file named out.txt (B) For the same structure, read the contents of the file out.txt and print the name of the highest salaried employee and youngest employee names name in the outputscreen.arrow_forward#include <iostream>#include <string>#include "hashT.h" using namespace std; class stateData{ friend ostream& operator<<(ostream&, const stateData&); // used to print state data on screen friend istream& operator>>(istream&, stateData&); // used to load data from file public: // setting values to the class object void setStateInfo(string sName, string sCapital, double stateArea, int yAdm, int oAdm); // retrieving data with call-by reference from the class object void getStateInfo(string& sName, string& sCapital, double& stateArea, int& yAdm, int& oAdm); string getStateName(); //return state name string getStateCapitalName(); //return state capital's name double getArea(); //return state's area int getYearOfAdmission();//return state's admision year int getOrderOfAdmission();//return state's order of admission //print stateName,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
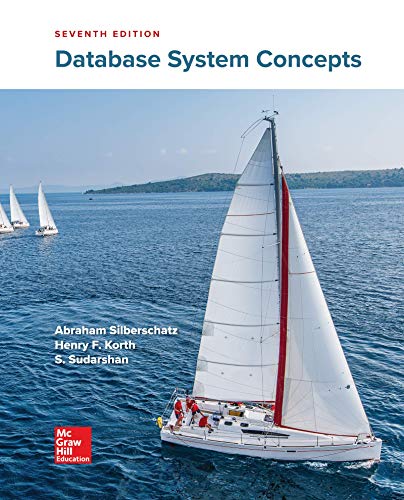
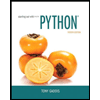
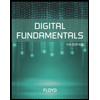
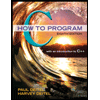
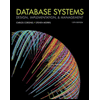
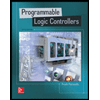