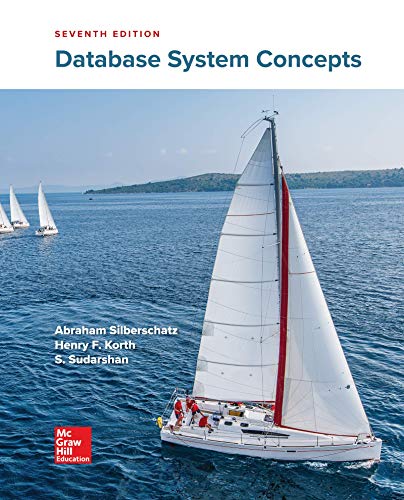
...
ex2_main.cpp
#include <stdio.h>
#include <stdlib.h>
#include "lab2_ex2.h"
#define FILENAME "statement11.txt"
int main()
{
// 1. Acquire the text from file
char *text = read_text_file(FILENAME); // Note: Caller should free the memory allocated
printf("%s\n", text);
// 2. Compute the readability and display the grade level
float readability = get_readability(text);
printf("Readability index = %f\n", readability);
display_level(readability);
// 3. Free resources, e.g. allocated memory by read_text_file() function
free(text);
return 0;
}
...
lab2_ex2.cpp
/*
EDIT THIS FILE TO SOLVE THE LABORATORY EXERCISES
*/
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#include <math.h>
#include <stdlib.h>
#include "lab2_ex2.h"
/*
Assume that a letter is any lowercase character from a to z
or any uppercase character from A to Z
*/
int alpanumeric_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
Assume that any sequence of characters separated by spaces or new line or tab , etc. should count as a word.
' ' space
'\t' horizontal tab
'\n' newline
'\r' carriage return
*/
int word_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
Assume that any occurrence of a period, exclamation point, or
question mark indicates the end of a sentence.
*/
int sentence_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
This function computes the readability according to the formula:
Coleman–Liau Index Formula
CLI = 0.0588 * L - 0.296 * S - 15.8
https://en.wikipedia.org/wiki/Coleman%E2%80%93Liau_index
The Coleman–Liau index is a readability test designed by Meri Coleman and T. L. Liau to
gauge the understandability of a text. Like the Flesch–Kincaid Grade Level, Gunning fog index,
SMOG index, and Automated Readability Index, its output approximates the U.S. grade level thought
necessary to comprehend the text.
Like the ARI but unlike most of the other indices, Coleman–Liau relies on characters instead of
syllables per word. Although opinion varies on its accuracy as compared to the syllable/word and
complex word indices, characters are more readily and accurately counted by computer programs than
are syllables.
The Coleman–Liau index was designed to be easily calculated mechanically from samples of hard-copy text.
Unlike syllable-based readability indices, it does not require that the character content of words be
analyzed, only their length in characters. Therefore, it could be used in conjunction with theoretically
simple
*/
float get_readability(const char *text)
{
/* YOUR CODE HERE!!! */
return 0.0f;
}
/*
This function accepts readability and displays the readability grade level for the text
*/
void display_level(float readability)
{
int level = round(readability);
if (level < 1)
{
printf("Before Grade level 1\n");
}
else if (level > 16)
{
printf("Grade level 16+\n");
}
else
{
printf("Grade level %i\n", level);
}
}
/*
This function reads in a text file given the filename
WARN: The caller needs to free the memory to avoid leaks
*/
char *read_text_file(const char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL)
{
printf("Error: could not open file %s", filename);
return NULL;
}
fseek(fp, 0, SEEK_END);
long length = ftell(fp);
fseek(fp, 0, SEEK_SET);
char *buffer = (char *)malloc(length + 1);
buffer[length] = '\0';
fread(buffer, 1, length, fp);
fclose(fp);
return buffer;
}
...
lab2_ex2.h
#ifndef lab2_ex2_h
#define lab2_ex2_h
int sentence_count(const char *);
int alpanumeric_count(const char *);
int word_count(const char *);
float get_readability(const char *);
void display_level(float);
char *read_text_file(const char *);
...
VERY IMPORTANT NOTE: Modify/edit/fix only lab2_ex1.cpp to make the program work.
i'm using VS Code on Windows

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 2 images

- Send the code in c++. Pseudocode: Open file Read header While Not End-Of-File Read line Increment record count Close file Declare array with length based on record count Read Header While Not End-Of-File Read line Split line into field(s) Convert numeric values to numeric data types Add field(s) to array or parallel arrays Close filearrow_forwardFile Stream #include<iostream>#include<string>#include<fstream>using namespace std;class Caesar{public: void encrypt(char *inp,char *out,int key);void decrypt(char *inp,char *out,int key);void readText(char *inp);void encrypt1();void decrypt1();};void Caesar::encrypt(char *inp,char *out,int key){ifstream input;ofstream output;char buf;input.open(inp);output.open(out);buf=input.get();while(!input.eof()){if(buf>='a'&&buf<='z'){buf-='a';buf+=key;buf%=26;buf+='A';}output.put(buf);buf=input.get();}input.close();output.close();readText(inp);readText(out);}void encrypt1(){char message[100], ch;int i, key;ofstream myfile ("encrypt.dat"); cout << "Enter a message to encrypt: ";cin>>message;cout << "\nEnter key: ";cin >> key; for(i = 0; message[i] != '\0'; ++i){ch = message[i]; if(ch >= 'a' && ch <= 'z'){ch = ch + key; if(ch > 'z'){ch = ch - 'z' + 'a' - 1;} message[i] = ch;}else if(ch >= 'A' && ch <= 'Z'){ch =…arrow_forward#include #includecstdlib.h> int main(void){ int number , reverse = 0, digit, temp; FILE *infile, "outfile; int status; //open the input file and check if an error occurred infile = fopen( "numbers.txt" , "r" );//open the input file if(infile == NULL){ printf("Error: File \"numbers.txt\" not foundl\n"); exit (1); //open the output file and check if error occurred outfile = fopen( "Report.txt", "w" ); if(outfile == NULL)X printf("Error: File \"Report.txt\" can not be created!\n"); exit (1); status = fscanf(infile, "%d" , &number); /read first number from the file while(status != EOF){ printf("%d is read from the file\n" , number); fprintf(outfile, "%8d" , number); //To read all the numbers in the file //compute the reverse of the number temp = number ; reverse = 0; while(temp != 0)X digit = temp % 10; reverse = reverse * 10 + digit ; temp = temp/10; printf("The reverse is: %d \n", reverse); fprintf(outfile , "%8d" , reverse); //check whether the number is palindrome if(number ==…arrow_forward
- What does fp point to in the program? #include int main() { } FILE *fp; fp=fopen("trial", "r"); return 0; The first character in the file ⓇA structure which contains a char pointer which points to the first character of a file. The name of the file. The last character in the file.arrow_forwardYou have the following function. 1 def funct(a): file = open('file.txt') 3 while True: line = file.readline() if a in line: 4 print(line) file.close() 7 The contents of the file named file.txt is as follows: python python is great! python is the best python is amazing! What would the value of a be if the output is: python is great! python is amazing! Answer:arrow_forwardChapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage est Case 1 Please enter the file name or type QUIT to exit:\ninput1.txtENTERTotal: 11.800\nAverage: 2.950\n Test Case 2 Please enter the file name or type QUIT to exit:\ninput2.txtENTERTotal: 17.300\nAverage: 3.460\n Test Case 3 Please enter the file name or type QUIT to exit:\ninput3.txtENTERTotal: 1.124\nAverage: 1.124\n Test Case 4 Please enter the file name or type QUIT to exit:\ninput4.txtENTERFile input4.txt is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\ninput5.txtENTERFile: input5.txt does not exist.\nPlease enter the file name again or type QUIT to…arrow_forward
- Do not copy paste from any source else downvote Write a void function that will merge the contents of two text files containing chemical elements sorted by atomic number and will produce a sorted file of binary records. The function’s parameters will be three file pointers. Each text file line will contain an integer atomic number followed by the element name, chemical symbol, and atomic weight. Here are two sample lines: 11 Sodium Na 22.99 20 Calcium Ca 40.08 The function can assume that one file does not have two copies of the same element and that the binary output file should have this same property. Hint: When one of the input files is exhausted, do not forget to copy the remaining elements of the other input file to the result file.arrow_forward2. Message Decoder Create a MessageDecoder program that reads from a file (one character at a time), and writes all non-numeric characters to a file called DecodedMessage.txt, displaying a secret (and motivational) message. ***Hint: .read() will return an int, but this can be type cast as a char. int and char are interchangeable (via type-caşting). You could run a loop on a condition like charInFile != (char) -1 Don't forget that -1 is a special character that denotes the end of a file!arrow_forwardPlease explain this codearrow_forward
- 1. Create a file that contains 20 integers or download the attached file twenty-integers.txttwenty-integers.txt reads:12 20 13 45 67 90 100 34 56 89 33 44 66 77 88 99 20 69 45 20 Create a program that: 2. Declares a c-style string array that will store a filename. 3. Prompts the user to enter a filename. Store the file name declared in the c-string. 4. Opens the file. Write code to check the file state. If the file fails to open, display a message and exit the program. 5. Declare an array that holds 20 integers. Read the 20 integers from the file into the array. 6. Write a function that accepts the filled array as a parameter and determines the MAXIMUM value in the array. Return the maximum value from the function (the function will be of type int). 7. Print ALL the array values AND print the maximum value in the array using a range-based for loop. Use informational messages. Ensure the output is readable.arrow_forwardUsing these files BinaryFileRead.cpp // BinaryFileRead.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include <iostream> using namespace std; int main() { int *arrayToSort; char fileName[50]; int size,readVal; cout << "Enter a filename to sort => "; cin >> fileName; FILE *inFile; fopen_s(&inFile,fileName, "rb"); fread(&size, sizeof(size), 1, inFile); arrayToSort = new int[size]; for (int i = 0; i < size; i++) { fread(&readVal, sizeof(readVal), 1, inFile); arrayToSort[i] = readVal; } fclose(inFile); return 0; } Timing.cpp // Timing.cpp : Defines the entry point for the console application. // #include "stdafx.h" #include <iostream> #include <time.h> //ctime #include <sys/timeb.h> //_timeb _ftime_s using namespace std; int main() { struct _timeb timebuffer; char timeline[26]; _ftime_s(&timebuffer); ctime_s(timeline,sizeof(timeline), &(timebuffer.time)); printf("The time is %.19s.%hu %s",…arrow_forwardNEED URGENT!!!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
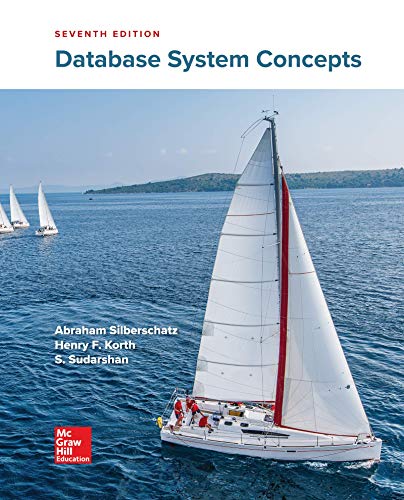
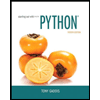
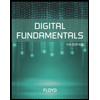
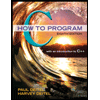
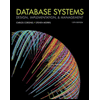
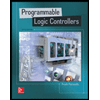