In this part of the course work, you are required to design and implement a class called HP_Laptop with instance variables specific to this type of items, a constructor with parameters which initialises class data members and invokes the Item_in_Stock constructor when it is invoked using inheritance concept in java and initialises data members of Item_in_stock class as well. This class should be designed and implemented in a way that it overrides getItemCategory() that returns the category of the item, getItemName() that returns HP_Laptop and getItemDescription() that returns “HP_I5_11G_8GB_1TB” ”. The Class should also override the get_item_details() method to display the complete details of an item. Task 2.1. Re-draw UML class diagram which was drawn in part 1 of the course work with this new class “HP_Laptop”. Relationships should also be represented. Task 2.2. Implement a class called Item_check with a main method to test HP_Laptop class by defining object of the class and invoking methods.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In this part of the course work, you are required to design and implement a class called HP_Laptop with instance variables specific to this type of items, a constructor with parameters which initialises class data members and invokes the Item_in_Stock constructor when it is invoked using inheritance concept in java and initialises data members of Item_in_stock class as well. This class should be designed and implemented in a way that it overrides getItemCategory() that returns the category of the item, getItemName() that returns HP_Laptop and getItemDescription() that returns “HP_I5_11G_8GB_1TB” ”. The Class should also override the get_item_details() method to display the complete details of an item.
Task 2.1. Re-draw UML class diagram which was drawn in part 1 of the course work with this new class “HP_Laptop”. Relationships should also be represented.
Task 2.2. Implement a class called Item_check with a main method to test HP_Laptop class by defining object of the class and invoking methods.
Testing is an integral part of development. Write suitable test cases in the given format below for your classes.
Test Case |
Purpose |
Expected result |
|
|
|
|
|
|
OUTPUT:
Refer to output in part I, item category, item name and item description should be according to the Items.

![public class StockedItem {
Run | Debug
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ItemInStock laptop = new ItemInStock (itemCode: "C15", quantity: 5, itemPrice: 180);
System.out.println(x: "Creating a stock with 5 units Laptops i-5 11g 8gb 1tb, price R.O 180 per item, and item code C15");
System.out.println();
System.out.println(x: "Laptops item stock information:");
laptop.getItemDetails();
System.out.println();
int option = -1;
while (option != 0) {
System.out.println(x: "What do you want to do?");
System.out.println(x: "1. Add items");
System.out.println(x: "2. Sell items");
System.out.println(x: "0. Exit");
option = scanner.nextInt ();
switch (option) {
case 1:
laptop.addItem();
System.out.println(x: "Adding 1 more item");
System.out.println();
System.out.println(x: "Printing item stock information:");
laptop.getItemDetails();
System.out.println();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff271ff2e-b53e-41b7-811c-c36006f06ab8%2F24c7e6c2-f15e-41a9-ba66-4330e7dc2ba8%2Fhpt5y4j_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

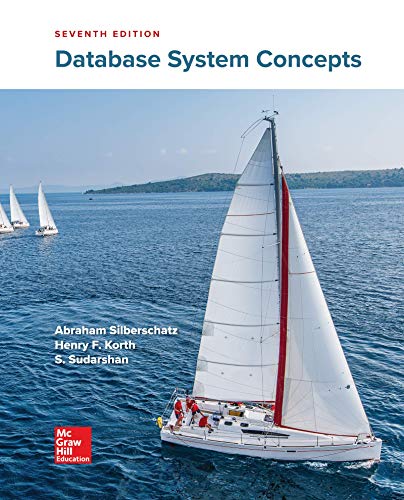
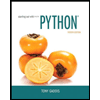
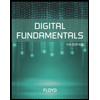
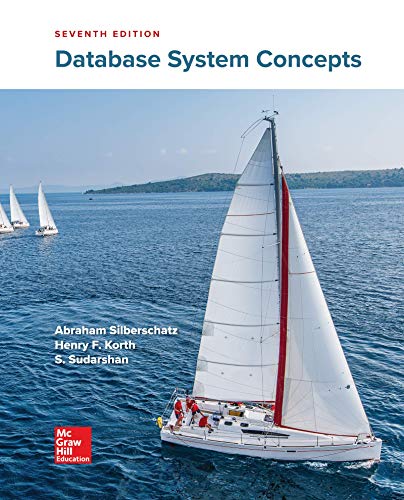
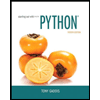
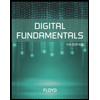
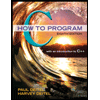
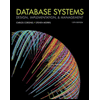
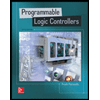