i have a parent class called Person containing the name and ID number of students and i need to write the program for a child class named student that extends from the parent class and also adds two more attributes below is a copy of my Parent class public class Person { private String name; private int id; public Person( ) { name = "not defined"; id = 0; } public Person(String newName, int newId ) { name = newName; id = newId; } public String getName( ) { return name; } public int getId( ) { return id; } public void setName(String newName) { name = newName; } public void setId(int newId) { id = newId; } public void set(String newName, int newId) { name = newName; id = newId; } public String toString( ) { return "Name = " + name + "ID is " + id; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
i have a parent class called Person containing the name and ID number of students and i need to write the program for a child class named student that extends from the parent class and also adds two more attributes
below is a copy of my Parent class
public class Person
{
private String name;
private int id;
public Person( )
{
name = "not defined";
id = 0;
}
public Person(String newName, int newId )
{
name = newName;
id = newId;
}
public String getName( )
{
return name;
}
public int getId( )
{
return id;
}
public void setName(String newName)
{
name = newName;
}
public void setId(int newId)
{
id = newId;
}
public void set(String newName, int newId)
{
name = newName;
id = newId;
}
public String toString( )
{
return "Name = " + name + "ID is " + id;
}
}

Here I have defined the class named Student.
While defining the class I have used extend keyword to inherit the parent class Person.
Inside the class Student, I have defined 2 data members to record marks and the city of the student.
Next, I have created getters and setters for the defined attributes.
Then, I have created the constructor and inside the parameterized constructor I have called the constructor of Person using super().
In the end, I have overridden the toString() method, and inside the method, I have called the parent's toString() using the super keyword.
Step by step
Solved in 2 steps

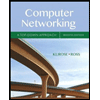
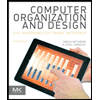
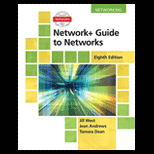
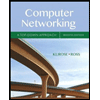
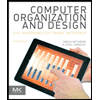
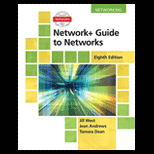
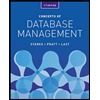
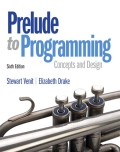
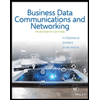