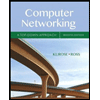
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Take the following code snippets (see below) and put them into a class called Employee.
- Then create two concrete (non-abstract) classes: Cashier and Supervisor
- Set the base salary of cashiers to be $30,000. Set the base salaries of supervisors to be $60,000. (use their constructors to do this).
- Create different methods for getMontlySalary() for the Cashier and Supervisor classes.
- a) for the Cashier class, just divide the basic salary by 12 to get the monthly.
- b) for the Supervisor class, also add a 10% bonus. So a supervisor before any
raises would earn $5500 per month. (60,000/12 + 10%)
- Create an employee tester class. Add an array of 10 employees. put 8 cashiers and 2 supervisors into the array. Print out the complete records of all 10 employees using a for loop. (Hint: Overload the toString() on all Employee class).
abstract class Employee {
private String name, address;
private int basicSalary;
public String getName(){ return name; }
public String getAddress(){ return address; }
public int getBasicSalary(){ return basicSalary; }
public void setAddress(String add){ address = add; }
public void setName(String nm){ name = nm; }
public void setBasicSalary(int sal){ basicSalary = sal; }
public abstract int getMonthlySalary();
} // end class Employee
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Similar questions
- Write all the code necessary for a class Truck. A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas tank is not between 15 and 20 gallons (inclusive). . That's 3 constructors in total. Each constructor, once done executing, should have fully initialized all of the object's properties .a default constructor Do not implement a toString method; assume one is properly defined for you. . Do not implement any getters or setters. In javaarrow_forwardPig Laten: Pig Latin is a farcical "language" used to entertain children, but also to teach them some valuable language concepts along the way. Translating English to Pig Latin is simple: Your challenge is to implement the method `pigLatinize` that takes a string phrase and translates it to Pig Latin. You're free to add additional classes, variables, and methods if you would like.arrow_forwardCreate a class JobApplicant that holds data about a job applicant. Include a name and four boolean fields that represent whether the applicant is skilled in each of the following areas: word processing, spread sheets, data base and security. The following shows a simple UML diagram with instance variables, constructor, an eligible method, and a display info method. Add in the accessor, mutator etc methods in your program. A qualified applicant has at least three of the four skills. In the eligible method you need to provide such test. The displayInfo method displays the info of an applicant (see below the sample format of printing). In the main method, you must construct at least 4 objects for 4 possible types of applicants. The following show the run time display:arrow_forward
- Defined the class circleType to implement the basic properties of a circle. (Add the function print to this class to output the radius, area, and circumference of a circle.) Now every cylinder has a base and height, where the base is a circle. Design a class cylinderType that can capture the properties of a cylinder and perform the usual operations on the cylinder. Derive this class from the class circleType designed in Chapter 10. Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Also, write a program to test various operations on a cylinder.arrow_forwardWhich of the following are true? If we derive a class from an abstract class, we can select which methods we choose to implement in our derived class. Abstract classed cannot have concrete methods. We can declare an instance of an abstract class. All of the above statements are false.arrow_forwardNeed help asap:arrow_forward
- Write a method for the farmer class that allows a farmer object to pet all cows on the farm. Do not use arrays.arrow_forward4. Write a class "machine" and "washing machine". Class "washing machine" should inherit from class "machine". Attributes of the machine will be: name, type, year. The washing machine should have the attributes: volume, depth, weight. In both classes should be method "show_attributes" which prints the attributes. Demonstrate in main function the usage of these classes.arrow_forwardWrite a class encapsulating the concept of the weather forecast, assuming that it has the following attributes: the temperature and the sky conditions (e.g. sunny, snowy, cloudy, rainy, etc.). Include a default constructor, an overloaded constructor, accessors and mutators, and the methods, toString() and equals(). Temperature, in Fahrenheit, should be between -50 and +150; the default value is 70, if needed. The default sky condition is sunny. In Addition, include a method that converts Fahrenheit to Celsius. Celsius temperature = (Fahrenheit temperature – 32) * 5/9. Also include a boolean method that checks whether the weather attributes are consistent (there are two cases where they are not consistent: when the temperature is below 32 and it is not snowy, and when the temperature is above 100 and it is not sunny). Write a client class to test all the methods in your class.arrow_forward
- This project has 3 classes which are already defined for you. Do not change any of these: Vehicle: an abstract class for any make and model of vehicle that can possibly travel some distance. LimitedRange: an interface for something that has a limit to the distance it can travel. Main: a test program which will work after you have written the code below. Your job is to write the following 3 classes so that this program produces the sample output shown at the end of Main. Specifically you will need: ElectricCar: a vehicle with a limited range. So it must be a subclass of Vehicle, and it must implement LimitedRange. Thus it needs an instance variable for its range, and a travel method (required by Vehicle. Please use @Override) that outputs "Zoom!" if it has enough range to go the distance specified (and update its range). It must have a constructor with 3 arguments as called by main. (Remember that the first line of the constructor must be a call to "super": Vehicle's constructor…arrow_forwardOverride the testOverriding() method in the Triangle class. Make it print “This is theoverridden testOverriding() method”. (add it to triangle class don't make a new code based on testOverriding in SimpleGeometricObject class.) (this was the step before this one) Add a void testOverriding() method to the SimpleGeometricObject class. Make it print“This is the testOverriding() method of the SimpleGeometricObject class”arrow_forwardwrite all the code necessary for a class Toaster as described here. A toaster can be described as having a model (string), manufacture(string), and price. include the following methods in your class definition.(in java) 1. a default constructor2. an overloaded constructor takes the model and manufacture as input.3. an accessor method to acquire the price.4. a tostring method that returns a reasonable description of the toaster.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
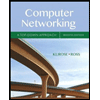
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
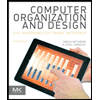
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
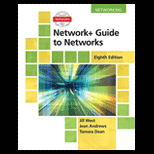
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
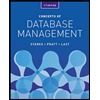
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
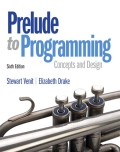
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
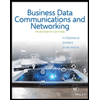
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY