Here you can find the java code and photo of challenge: import java.io.*; import java.math.*; import java.security.*; import java.text.*; import java.util.*; import java.util.concurrent.*; import java.util.function.*; import java.util.regex.*; import java.util.stream.*; public class Solution { public static class DirectedGraph { /* Adjacency List representation of the given graph */ private Map> adjList = new HashMap>(); public String toString() { StringBuffer s = new StringBuffer(); for (Integer v : adjList.keySet()) s.append("\n " + v + " -> " + adjList.get(v)); return s.toString(); } public void add(Integer vertex) { if (adjList.containsKey(vertex)) return; adjList.put(vertex, new ArrayList()); } public void add(Integer source, Integer dest) { add(source); add(dest); adjList.get(source).add(dest); } /* Indegree of each vertex as a Map */ public Map inDegree() { Map result = new HashMap(); for (Integer v : adjList.keySet()) result.put(v, 0); for (Integer from : adjList.keySet()) { for (Integer to : adjList.get(from)) { result.put(to, result.get(to) + 1); } } return result; } public Map outDegree () { Map result = new HashMap(); for (Integer v: adjList.keySet()) result.put(v, adjList.get(v).size()); return result; } } // Complete the isDAG function below. public static boolean isDag(DirectedGraph digraph) { } public static void main(String[] args) throws IOException { BufferedWriter bufferredWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH"))); BufferedReader bufferredReader = new BufferedReader(new InputStreamReader(System.in)); DirectedGraph digraph = new DirectedGraph(); String line; while ((line = bufferredReader.readLine()) != null) { String[] v = line.split(" "); digraph.add(Integer.parseInt(v[0]), Integer.parseInt(v[1])); } bufferredWriter.write((isDag(digraph) ? "1" : "0")); bufferredReader.close(); bufferredWriter.close(); } }
Here you can find the java code and photo of challenge:
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
public class Solution {
public static class DirectedGraph {
/* Adjacency List representation of the given graph */
private Map<Integer, List<Integer>> adjList = new HashMap<Integer, List<Integer>>();
public String toString() {
StringBuffer s = new StringBuffer();
for (Integer v : adjList.keySet())
s.append("\n " + v + " -> " + adjList.get(v));
return s.toString();
}
public void add(Integer vertex) {
if (adjList.containsKey(vertex))
return;
adjList.put(vertex, new ArrayList<Integer>());
}
public void add(Integer source, Integer dest) {
add(source);
add(dest);
adjList.get(source).add(dest);
}
/* Indegree of each vertex as a Map<Vertex, IndegreeValue> */
public Map<Integer, Integer> inDegree() {
Map<Integer, Integer> result = new HashMap<Integer, Integer>();
for (Integer v : adjList.keySet())
result.put(v, 0);
for (Integer from : adjList.keySet()) {
for (Integer to : adjList.get(from)) {
result.put(to, result.get(to) + 1);
}
}
return result;
}
public Map<Integer,Integer> outDegree () {
Map<Integer,Integer> result = new HashMap<Integer,Integer>();
for (Integer v: adjList.keySet()) result.put(v, adjList.get(v).size());
return result;
}
}
// Complete the isDAG function below.
public static boolean isDag(DirectedGraph digraph) {
}
public static void main(String[] args) throws IOException {
BufferedWriter bufferredWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
BufferedReader bufferredReader = new BufferedReader(new InputStreamReader(System.in));
DirectedGraph digraph = new DirectedGraph();
String line;
while ((line = bufferredReader.readLine()) != null) {
String[] v = line.split(" ");
digraph.add(Integer.parseInt(v[0]), Integer.parseInt(v[1]));
}
bufferredWriter.write((isDag(digraph) ? "1" : "0"));
bufferredReader.close();
bufferredWriter.close();
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

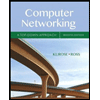
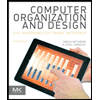
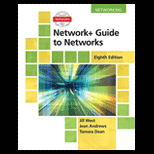
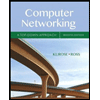
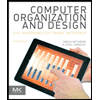
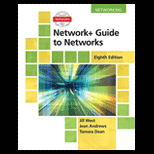
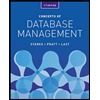
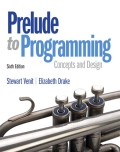
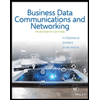