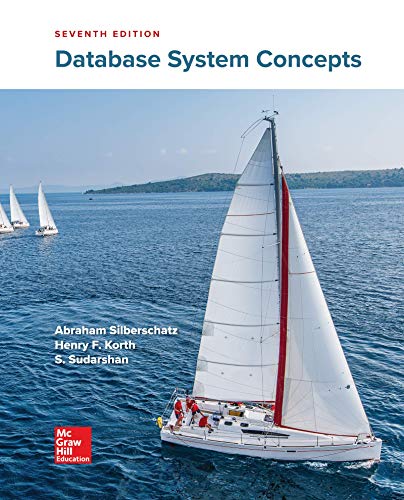
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
JAVA: I have the following attributes and I am trying to get the square root of the last 4 digits of student Id without decimal places
class Student{
private String studentId = "4596";
//with getters and setters
public void generateId(){
Integer.parseInt(this.getStudentId());
}
}
another class Test{
//main
Student std1 = new Student();
std1.generateId();
//I am trying to print out the square root of the last digit of the studentId
System.out.println("Square root of last four digit of studentId: " + Math.sqrt(Integer.parseInt(std1.getStudentId().substring(2))) );
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java Instance data:Variable mpg for fuel efficiency (miles per gallon = mpg)Variable gas to save how many gallons of gas left in the tank Constructors:Default constructor with no parameter. Use 0 as initial values.Overloaded constructor with two parameters Methods:getMPG() & setMPG()(getGas() & setGas()toString() methoddrive() to simulate that the car is driven for certain miles. For example, v1.drive(100) means vehicle v1 is driven 100 miles. You need to calculate the gas cost and update the gas tank: gas = gas - miles/mpg. You also need to check if there is enough gas left since gas should not be negative. You need to figure out the formal parameters for the above methods. In the testing class, prompt the user for information to create two objects of the Vehicle class. Let each vehicle drive 200 miles. Print out the left gas for each vehicle. Ex: Vehicle 1 Enter the mpg: 40 Enter the gas left: 10.5 Vehicle 2 Enter the mpg: 35 Enter the gas left: 2.1 Vehicle 1…arrow_forwardComplete the Course class by implementing the courseSize() method, which returns the total number of students in the course. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex. For the following students: Henry Bendel 3.6 Johnny Min 2.9 the output is: Course size: 2 public class LabProgram { public static void main (String [] args) { Course course = new Course(); // Example students for testing course.addStudent(new Student("Henry", "Bendel", 3.6)); course.addStudent(new Student("Johnny", "Min", 2.9)); System.out.println("Course size: " + course.courseSize()); } } public class Student { private String first; // first name private String last; // last name private…arrow_forwardGiven: public class Team { private Player[] players; private int squadSize; public Team(Player[] players) { this.players = players; this.size = players.length; } public Team() { this.players = new Player[4]; this.size = 0; } }How would I go about creating the given methods? (Rating variable in supplied java file.)arrow_forward
- Create a fee invoice application for students attending Valence College, a college in the State of Florida. There are two types of students: graduate and undergraduate. Out-of-state undergraduate students pay twice the tuition of Florida-resident undergraduate students (all students pay the same health and id fees). A graduate student is either a PhD or a MS student. PhD students don't take any courses, but each has an advisor and a research subject. Each Ms and Phd student must be a teaching assistant for one undergraduate course. MS students can only take graduate courses. A course with a Course Number (crn) less than 5000 is considered an undergraduate course, and courses with a 5000 crn or higher are graduate courses. CRN Course Credit Hours. 4587 MAT 236 4 4599 COP 220 3 8997 GOL 124 1 9696 COP 100 3 4580 MAT 136 1 2599 COP 260 3 1997 CAP 424 1 5696 KOL 110 2 7587 MAT 936 5 2599 COP 111 3 6997 GOL 109 1 2696 COP 101 3 5580 MAT 636 2 2099 COP 268 3 4997 CAP 427 1 3696 KOL 910 2…arrow_forwardGiven the following class definition: class Point2d { private int x, y; public Point2d () { X = 0; y = 0; } public Point2d (int i, int j) { X = i; y = j; } public void SetX (int i) { x = i; } public void SetY (int j) { y = j; } public int GetX () { return x; public int GetŸ () { return y; } public String toString() { return '[' + x + ", } + y + ']'; } } Use inheritance to create a class, Point3d, with any additional appropriate data and/or functions.arrow_forwardFind the error(s) in the following code snippet and explain how to correct it (them): 1 class Example { 2 public: Example(int y = 3 10) : data (y) {} 4 int getIncrementedData() const { return ++data; 7 } 8. 9 private: 10 int data; 11 };arrow_forward
- Complete the Kennel class by implementing the following methods: addDog(Dog dog) findYoungestDog() method, which returns the Dog object with the lowest age in the kennel. Assume that no two dogs have the same age. Given classes: Class LabProgram contains the main method for testing the program. Class Kennel represents a kennel, which contains an array of Dog objects as a dog list. (Type your code in here.) Class Dog represents a dog, which has three fields: name, breed, and age. (Hint: getAge() returns a dog's age.) For testing purposes, different dog values will be used. Ex. For the following dogs: Rex Labrador 3.5 Fido Healer 2.0 Snoopy Beagle 3.2 Benji Spaniel 3.9 the output is: Youngest Dog: Fido (Healer) (Age: 2.0)arrow_forwardPart 1 is already done and here it is public class Point { protected double x; protected double y; public Point() { } public Point(double x, double y) { this.x = x; this.y = y; } public double getX() { return x; } public double getY() { return y; } public void setPoint(Point X){ x = X.x; y = X.y; } void makeCopy(Point x){ setPoint(x); } Point getCopy(){ return this; } public void printPoint(){ System.out.println("["+this.x+", "+this.y+"]"); } @Override public String toString() { return "x-Coordinate is " + x + "and y-coordinate is " + y; } public boolean equals(Point X) {…arrow_forward2-7 Given the code below, which lines are valid and invalid.arrow_forward
- Can you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forwardPlease help debug this: Debugging Exercise 4-3, Farrell, Joyce, Java Programming, 9th edition, Cengage Learning public class DebugBox { private int width; private int length; private int height; private FixDebugBox() { length = 1; width = 1; height = 1; } public DebugBox(int width, int length, int height) { width = width; length = length; height = height; } public void showData() { System.out.println("Width: " + width + " Length: " + length + " Height: " + height); } public double getVolume() { double vol = length - width - height; return vol; } }arrow_forwardHi, can i get assistance with this book class in Java please? The comments with the "TODO" is what I need assistance with, thank you! The author class is attached to the question in case there is any relevance for it. public class Book {private String title;private double averageRating;private String ISBN;private int numPages;// TODO: insert an appropriate collection for associating Authors and initialise within constructors public Book(){} public Book(String title, double averageRating, String isbn, int numPages){ this.title = title;this.averageRating = averageRating;this.ISBN = isbn;this.numPages = numPages;}//accessorspublic String getTitle(){return title;}public double getAverageRating(){return averageRating;}public String getISBN(){return ISBN;}public int getNumPages(){return numPages;}// TODO: provide an accessor for the book collection public String getAuthorList(){String authorList ="";// TODO: insert code to return all author names separated by commas if multiple authorsreturn…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
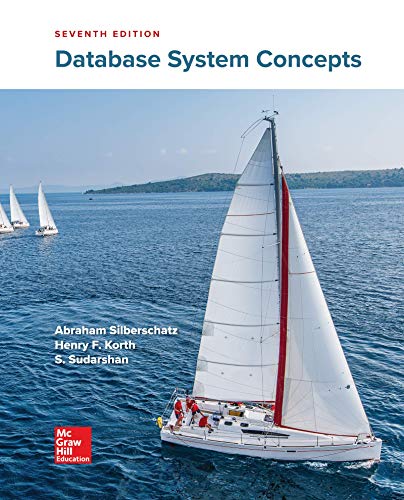
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
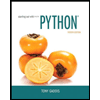
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
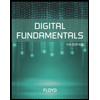
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
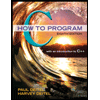
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
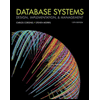
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
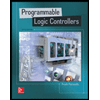
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education