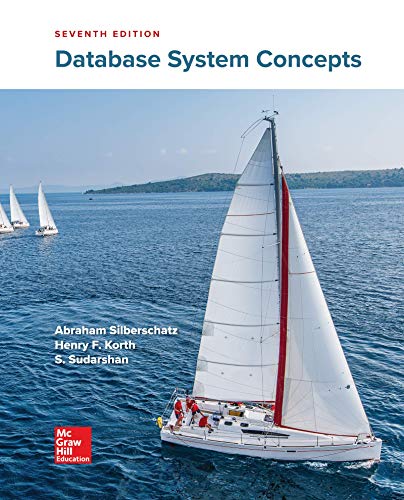
Concept explainers
Enter integer: 4
You entered: 4
4 squared is 16
And 4 cubed is 64!!
Enter another integer:
5
4 + 5 is 9
4 * 5 is 20
===================================
#include <stdio.h>
int main(void) {
int userNum;
int userNum2;
printf("Enter integer:\n");
scanf("%d",&userNum);
printf("You entered: ");
printf( "%d", userNum);
printf("\n");
printf("%d", userNum);
printf( " squared is ");
printf("%d", userNum * userNum);
printf("\n");
printf("And ");
printf("%d", userNum);
printf(" cubed is ");
printf("%d", userNum * userNum * userNum);
printf("!!");
printf("\n" "Enter another integer:\n");
scanf("%d",&userNum2);
printf("\n");
printf("%d", userNum2);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- my_round(number, integer): Based on the built-in round(...) function. Takes an integer or float and returns a rounded float value that is the number rounded to the second argument's decimal place. First argument can be any float or integer. Second argument must be an integer. Examples: my_round(1234.5678, 2) and round(1234.5678, 2) should return 1234.57. my_round(1234.5678, -2) and round(1234.5678, -2) should return 1200.0.arrow_forwardC++ CODE HELP NEEDED NEED MISSING CODE THAT WILL Read an integer as the number of Goat objects. Assign myGoats with an array of that many Goat objects. For each object, call object's Read() followed by the object's Print(). Ex: If the input is 2 5 87 7 71, then the output is: Goat's age: 5 Goat's weight: 87 Goat's age: 7 Goat's weight: 71 Goat with age 7 and weight 71 is deallocated. Goat with age 5 and weight 87 is deallocated. #include <iostream>using namespace std; class Goat { public: Goat(); void Read(); void Print(); ~Goat(); private: int age; int weight;};Goat::Goat() { age = 0; weight = 0;}void Goat::Read() { cin >> age; cin >> weight;}void Goat::Print() { cout << "Goat's age: " << age << endl; cout << "Goat's weight: " << weight << endl;}Goat::~Goat() { // Covered in section on Destructors. cout << "Goat with age " << age << " and weight " <<…arrow_forwardint main(){ long long int total; long long int init; scanf("%lld %lld", &total, &init); getchar(); long long int max = init; long long int min = init; int i; for (i = 0; i < total; i++) { char op1 = '0'; char op2 = '0'; long long int num1 = 0; long long int num2 = 0; scanf("%c %lld %c %lld", &op1, &num1, &op2, &num2); getchar(); long long int maxr = max; long long int minr = min; if (op1 == '+') { long long int sum = max + num1; maxr = sum; minr = sum; long long int res = min + num1; if (res > maxr) { max = res; } if (res < minr) { minr = res; } } else { long long int sum = max * num1; maxr = sum; minr = sum; long long int res = min * num1;…arrow_forward
- JAVA ONLYPlease create a code that checks if 'user' favourite game is in the top 3 games. if the game is in the top 3 then it should say: "common favourite game" if else then "uncommon favourite game"code: TOP_3_GAMES = {'', 'CALL OF DUTY', 'LEAGUE OF LEGENDS', 'VALORANT'}arrow_forwardint main(int argc, char **argv) { float *rainfall; float rain_today; // rainfall has been dynamically allocated space for a floating point number. // Both rainfall and rain today have been initialized in hidden code. // Assign the amount in rain_today to the space rainfall points to. return 0; }arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- #include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardin C code not c++arrow_forward//below is the code I need help with answering the questions in the image// #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for (int i = 0; i < 9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees *e) { printf("%s",…arrow_forward
- //below is the code I need help with answering the questions in the image// #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for (int i = 0; i < 9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees *e) { printf("%s",…arrow_forwardselect all options that appliesarrow_forward#include <iostream>#include <string>#include "hashT.h" using namespace std; class stateData{ friend ostream& operator<<(ostream&, const stateData&); // used to print state data on screen friend istream& operator>>(istream&, stateData&); // used to load data from file public: // setting values to the class object void setStateInfo(string sName, string sCapital, double stateArea, int yAdm, int oAdm); // retrieving data with call-by reference from the class object void getStateInfo(string& sName, string& sCapital, double& stateArea, int& yAdm, int& oAdm); string getStateName(); //return state name string getStateCapitalName(); //return state capital's name double getArea(); //return state's area int getYearOfAdmission();//return state's admision year int getOrderOfAdmission();//return state's order of admission //print stateName,…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
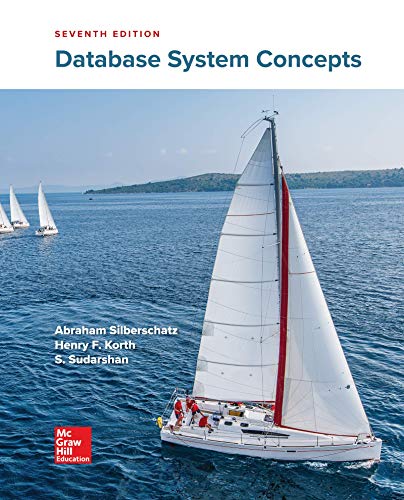
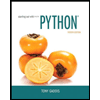
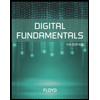
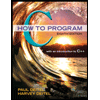
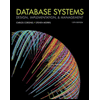
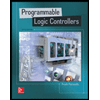