import java.util.Arrays; import java.util.Scanner; public class Game { private Player[] players; private Board board; private int currentPlayerIndex; private int numOfPlayers; private Scanner scanner = new Scanner(System.in); // constructor public Game(){ //create a new board; this.board = new Board(); //setup game; this.setupGame(); //start game; this.play(); } //Game Setup public void setupGame(){ System.out.println("How many players would you like to included in this game? (2-4)"); numOfPlayers = scanner.nextInt(); while (numOfPlayers < 2 || numOfPlayers > 4) { System.out.println("Invalid input. Please enter a number between 2 and 4."); numOfPlayers = scanner.nextInt(); } scanner.nextLine(); String[] playerNames = new String[numOfPlayers]; this.players = new Player[numOfPlayers]; for (int i = 0; i < numOfPlayers; i++) { System.out.println("Enter player " + (i + 1) + " name:"); String newPlayerName = scanner.nextLine(); //Makes sure players don't have the same name while (Arrays.asList(playerNames).contains(newPlayerName)) { System.out.println("Player names cannot be the same. Please enter a different name."); System.out.print("Enter player " + (i + 1) + " name: "); newPlayerName = scanner.nextLine(); } playerNames[i] = newPlayerName; // adds player to array players[i] = new Player(newPlayerName, Integer.toString(i + 1)); } //setup board this.board.boardSetUp(); //set starting player to a random index this.currentPlayerIndex = (int) Math.floor(Math.random() * numOfPlayers); } //Print winner private void printWinner(Player player){ System.out.println("------------------------------------------------"); System.out.println(" | Player " +player.getPlayerNumber()+" which is "+player.getPlayerName() +" wins | "); System.out.println("------------------------------------------------"); } private void playerTurn(Player currentPlayer){ System.out.println("\n---------Player " + currentPlayer.getPlayerNumber() +" turn -----\n"); // so to be within the bounds of the array. int move = currentPlayer.makeMove() - 1; //to check if the move if(this.board.columnFull(move)) { System.out.println("Column is full."); } //To make sure our column numbers are always valid if(move< 0 || move >= this.board.getColumns()){ System.out.println("Invalid column number."); } //update board to add token this.board.addToken(move, currentPlayer.getPlayerNumber()); } } private void play() { while(!this.board.boardFull()){ Player currentPlayer = players[currentPlayerIndex]; //print board this.board.printBoard(); //to make a move this.playerTurn(currentPlayer); //catch block System.out.println("Something went wrong adding a token to the playerTurn function: " + e);; //if a player wins print the current player if(this.board.checkIfPlayerIsWinner(currentPlayer.getPlayerNumber())) { this.board.printBoard(); this.printWinner(currentPlayer); return; // ends game when a player wins; } //alternate current player setPlayerIndex(); } System.out.println("Board is full!"); } //to set current player Index private void setPlayerIndex() { this.currentPlayerIndex = (this.currentPlayerIndex + 1) % numOfPlayers; } } import java.util.Scanner; public class Player { //initial variables private String playerName; private String playerNumber; private static Scanner scanner = new Scanner(System.in); //constructor for player public Player(String playerName, String playerNumber) { this.playerName = playerName; this.playerNumber = playerNumber; } public String getPlayerName() { return this.playerName; } public String getPlayerNumber() { return this.playerNumber; } public int makeMove() { System.out.println("Enter the coloumn num to put your token"); return scanner.nextInt(); } public String toString(){ return "Player " +playerNumber+ " is " + playerName; } }
import java.util.Arrays; import java.util.Scanner; public class Game { private Player[] players; private Board board; private int currentPlayerIndex; private int numOfPlayers; private Scanner scanner = new Scanner(System.in); // constructor public Game(){ //create a new board; this.board = new Board(); //setup game; this.setupGame(); //start game; this.play(); } //Game Setup public void setupGame(){ System.out.println("How many players would you like to included in this game? (2-4)"); numOfPlayers = scanner.nextInt(); while (numOfPlayers < 2 || numOfPlayers > 4) { System.out.println("Invalid input. Please enter a number between 2 and 4."); numOfPlayers = scanner.nextInt(); } scanner.nextLine(); String[] playerNames = new String[numOfPlayers]; this.players = new Player[numOfPlayers]; for (int i = 0; i < numOfPlayers; i++) { System.out.println("Enter player " + (i + 1) + " name:"); String newPlayerName = scanner.nextLine(); //Makes sure players don't have the same name while (Arrays.asList(playerNames).contains(newPlayerName)) { System.out.println("Player names cannot be the same. Please enter a different name."); System.out.print("Enter player " + (i + 1) + " name: "); newPlayerName = scanner.nextLine(); } playerNames[i] = newPlayerName; // adds player to array players[i] = new Player(newPlayerName, Integer.toString(i + 1)); } //setup board this.board.boardSetUp(); //set starting player to a random index this.currentPlayerIndex = (int) Math.floor(Math.random() * numOfPlayers); } //Print winner private void printWinner(Player player){ System.out.println("------------------------------------------------"); System.out.println(" | Player " +player.getPlayerNumber()+" which is "+player.getPlayerName() +" wins | "); System.out.println("------------------------------------------------"); } private void playerTurn(Player currentPlayer){ System.out.println("\n---------Player " + currentPlayer.getPlayerNumber() +" turn -----\n"); // so to be within the bounds of the array. int move = currentPlayer.makeMove() - 1; //to check if the move if(this.board.columnFull(move)) { System.out.println("Column is full."); } //To make sure our column numbers are always valid if(move< 0 || move >= this.board.getColumns()){ System.out.println("Invalid column number."); } //update board to add token this.board.addToken(move, currentPlayer.getPlayerNumber()); } } private void play() { while(!this.board.boardFull()){ Player currentPlayer = players[currentPlayerIndex]; //print board this.board.printBoard(); //to make a move this.playerTurn(currentPlayer); //catch block System.out.println("Something went wrong adding a token to the playerTurn function: " + e);; //if a player wins print the current player if(this.board.checkIfPlayerIsWinner(currentPlayer.getPlayerNumber())) { this.board.printBoard(); this.printWinner(currentPlayer); return; // ends game when a player wins; } //alternate current player setPlayerIndex(); } System.out.println("Board is full!"); } //to set current player Index private void setPlayerIndex() { this.currentPlayerIndex = (this.currentPlayerIndex + 1) % numOfPlayers; } } import java.util.Scanner; public class Player { //initial variables private String playerName; private String playerNumber; private static Scanner scanner = new Scanner(System.in); //constructor for player public Player(String playerName, String playerNumber) { this.playerName = playerName; this.playerNumber = playerNumber; } public String getPlayerName() { return this.playerName; } public String getPlayerNumber() { return this.playerNumber; } public int makeMove() { System.out.println("Enter the coloumn num to put your token"); return scanner.nextInt(); } public String toString(){ return "Player " +playerNumber+ " is " + playerName; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
-
-
import java.util.Arrays;import java.util.Scanner;
public class Game {private Player[] players;private Board board;private int currentPlayerIndex;private int numOfPlayers;
private Scanner scanner = new Scanner(System.in);// constructorpublic Game(){//create a new board;this.board = new Board();//setup game;this.setupGame();//start game;this.play();}//Game Setuppublic void setupGame(){
System.out.println("How many players would you like to included in this game? (2-4)");numOfPlayers = scanner.nextInt();while (numOfPlayers < 2 || numOfPlayers > 4) {System.out.println("Invalid input. Please enter a number between 2 and 4.");numOfPlayers = scanner.nextInt();}scanner.nextLine();String[] playerNames = new String[numOfPlayers];this.players = new Player[numOfPlayers];
for (int i = 0; i < numOfPlayers; i++) {System.out.println("Enter player " + (i + 1) + " name:");String newPlayerName = scanner.nextLine();//Makes sure players don't have the same namewhile (Arrays.asList(playerNames).contains(newPlayerName)) {System.out.println("Player names cannot be the same. Please enter a different name.");System.out.print("Enter player " + (i + 1) + " name: ");newPlayerName = scanner.nextLine();}playerNames[i] = newPlayerName;// adds player to arrayplayers[i] = new Player(newPlayerName, Integer.toString(i + 1));}
//setup boardthis.board.boardSetUp();//set starting player to a random indexthis.currentPlayerIndex = (int) Math.floor(Math.random() * numOfPlayers);}//Print winnerprivate void printWinner(Player player){System.out.println("------------------------------------------------");System.out.println(" | Player " +player.getPlayerNumber()+" which is "+player.getPlayerName() +" wins | ");System.out.println("------------------------------------------------");}private void playerTurn(Player currentPlayer){System.out.println("\n---------Player " + currentPlayer.getPlayerNumber() +" turn -----\n");// so to be within the bounds of the array.int move = currentPlayer.makeMove() - 1;//to check if the moveif(this.board.columnFull(move)) {System.out.println("Column is full.");}//To make sure our column numbers are always validif(move< 0 || move >= this.board.getColumns()){System.out.println("Invalid column number.");}//update board to add tokenthis.board.addToken(move, currentPlayer.getPlayerNumber());}
}private void play() {while(!this.board.boardFull()){Player currentPlayer = players[currentPlayerIndex];//print boardthis.board.printBoard();//to make a movethis.playerTurn(currentPlayer);//catch blockSystem.out.println("Something went wrong adding a token to the playerTurn function: " + e);;//if a player wins print the current playerif(this.board.checkIfPlayerIsWinner(currentPlayer.getPlayerNumber())) {this.board.printBoard();this.printWinner(currentPlayer);return; // ends game when a player wins;}//alternate current playersetPlayerIndex();}System.out.println("Board is full!");}//to set current player Indexprivate void setPlayerIndex() {this.currentPlayerIndex = (this.currentPlayerIndex + 1) % numOfPlayers;}
}
import java.util.Scanner;
public class Player {//initial variablesprivate String playerName;private String playerNumber;private static Scanner scanner = new Scanner(System.in);//constructor for playerpublic Player(String playerName, String playerNumber) {this.playerName = playerName;this.playerNumber = playerNumber;}
public String getPlayerName() {return this.playerName;}
public String getPlayerNumber() {return this.playerNumber;}public int makeMove() {System.out.println("Enter the coloumn num to put your token");return scanner.nextInt();}public String toString(){return "Player " +playerNumber+ " is " + playerName;}}
-
![public void play()- this method is called to start the game play() method is called to start the game.
• initializes a boolean variable nowinner to true.
• calls the setupGame () method to get the board and player ready for the game.
• initializes an integer variable currentPlayerIndex to 0.
• enters a while loop that continues as long as nowinner is true.
• Inside the while loop, it checks if the board is full using the boardFull() method of the board object.
• If the board is full, it prints a message saying "Board is now full. Game Ends." and returns.
• If the board is not full, it assigns the current player to the players array at the currentPlayerIndex position.
• It then prints a message saying "It is player [player number]'s turn. [player name]"
• It then calls the playerTurn (currentPlayer) method, passing the current player as an argument.
• It then checks if the current player is the winner using the checkIfPlayer Is Thewinner (currentPlayer.getPlayer Number()) method of the
board object.
• If the current player is the winner, it calls the printwinner (currentPlayer) method, passing the current player as an argument.
• It then sets the nowinner variable to false, ending the while loop.
• If the current player is not the winner, it increments the currentPlayer Index variable by 1, and takes the modulus of that value by the
length of the players array. This ensures that the index stays within the range](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cab9c1-3149-4232-8d34-9f213defe2a3%2F2b4a085c-62f5-49bb-b5ea-917236fe7b0c%2Ftlll9r6_processed.jpeg&w=3840&q=75)
Transcribed Image Text:public void play()- this method is called to start the game play() method is called to start the game.
• initializes a boolean variable nowinner to true.
• calls the setupGame () method to get the board and player ready for the game.
• initializes an integer variable currentPlayerIndex to 0.
• enters a while loop that continues as long as nowinner is true.
• Inside the while loop, it checks if the board is full using the boardFull() method of the board object.
• If the board is full, it prints a message saying "Board is now full. Game Ends." and returns.
• If the board is not full, it assigns the current player to the players array at the currentPlayerIndex position.
• It then prints a message saying "It is player [player number]'s turn. [player name]"
• It then calls the playerTurn (currentPlayer) method, passing the current player as an argument.
• It then checks if the current player is the winner using the checkIfPlayer Is Thewinner (currentPlayer.getPlayer Number()) method of the
board object.
• If the current player is the winner, it calls the printwinner (currentPlayer) method, passing the current player as an argument.
• It then sets the nowinner variable to false, ending the while loop.
• If the current player is not the winner, it increments the currentPlayer Index variable by 1, and takes the modulus of that value by the
length of the players array. This ensures that the index stays within the range

Transcribed Image Text:public void playerTurn (Player currentPlayer)- method takes in a Player object as a parameter, and is responsible for handling the
actions taken by the player during their turn.
The method starts by trying to execute the following block of code:
• The player is prompted to make a move by calling currentPlayer.makeMove (). This method returns an int value representing the column
the player wants to place their token in.
• The addToken method of the board object is called, passing in the column and the player's name. This method attempts to place the
player's token in the selected column. If the column is full or the column number exceeds the number of columns on the board, an
exception is thrown.
• If the token is successfully placed, the board is printed to show the updated state of the game.
The method uses a try-catch block to handle any exceptions that might be thrown while executing the try block. There are four different
types of exceptions that can be thrown in this case:
InvalidMoveException: Thrown when the column number exceeds the number of columns on the board.
Input MismatchException: Thrown when the player does not provide a valid input for the column number.
Column FullException: Thrown when the selected column is full and cannot accept any more tokens.
Exception: A catch-all exception to handle any other unexpected exceptions that might be thrown.
●
●
●
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
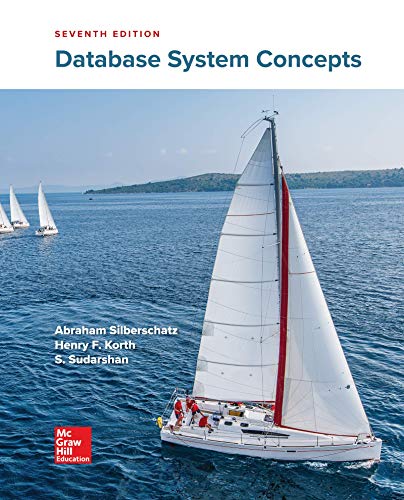
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
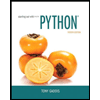
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
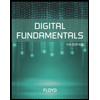
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
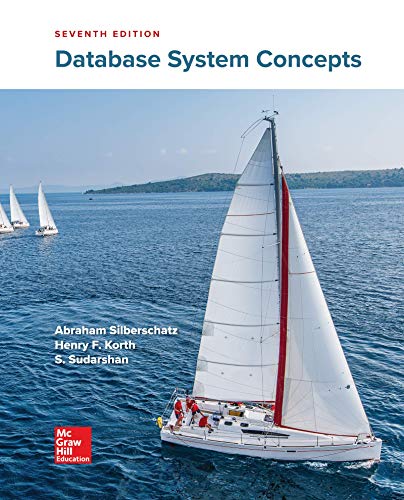
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
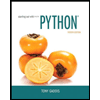
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
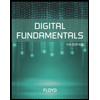
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
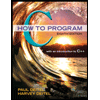
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
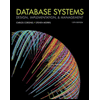
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
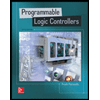
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education