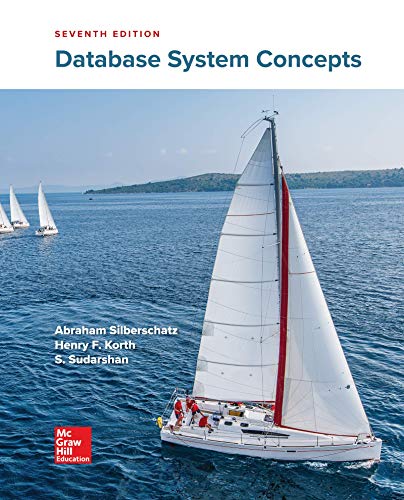
Do not use static variables to implement recursive methods.
USING JAVA:
// P6
public static int countSubstrings(String s1, String s2) {
}
- Write a recursive method countSubstrings that counts the number of non-overlapping occurrences of a string s2 in a string s1. Use the method indexOf() from String class.
- As an example, with s1=”abab” and s2=”ab”, countSubstrings returns 2. With s1=”aaabbaa” and s2=”aa”, countSubstrings returns 2. With s1=”aabbaa” and s2=”AA”, countSubStrings returns 0.
Show the output on the following strings:
- s1 = “Java is a
programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. Java is currently one of the most popular programming languages in use, particularly for client-server web applications, with a reported 10 million users.”
- s2= “Java”.

code : -
public class SubstringCounter {
public static int countSubstrings(String s1, String s2) {
// base case: s1 is empty or s2 is longer than s1
if (s1.isEmpty() || s2.length() > s1.length()) {
return 0;
}
// find the index of the first occurrence of s2 in s1
int index = s1.indexOf(s2);
// if s2 is not found in s1, return 0
if (index == -1) {
return 0;
}
// increment the count by 1 and recursively call countSubstrings
// on the substring of s1 that starts after the first occurrence of s2
// and the same s2 string
return 1 + countSubstrings(s1.substring(index + s2.length()), s2);
}
public static void main(String[] args) {
String s1 = "Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995 as a core component of Sun Microsystems Java platform. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java applications are typically compiled to bytecode (class file) that can run on any Java Virtual Machine (JVM) regardless of computer architecture. Java is a general-purpose, concurrent, class-based, object-oriented language that is specifically designed to have as few implementation dependencies as possible. Java is currently one of the most popular programming languages in use, particularly for client-server web applications, with a reported 10 million users.";
String s2 = "Java";
int count = countSubstrings(s1, s2);
System.out.println("Number of non-overlapping occurrences of \"" + s2 + "\" in \"" + s1 + "\": " + count);
// second test case
s1="abab";
s2="ab";
count = countSubstrings(s1, s2);
System.out.println("\n\n\nNumber of non-overlapping occurrences of \"" + s2 + "\" in \"" + s1 + "\": " + count);
}
}
Step by stepSolved in 2 steps with 1 images

- Solve in javaFXarrow_forwardPlease help me fix the errors in the java program belowarrow_forwardWrite a recursive method that gets three parameters as input: an array of integers called nums, an integer called index,and an integer called The purpose of this method is to return true if value is stored in the array starting at nums[index]. That is, you have to check if value is equal to nums[index] or nums[index +1] or nums[index +2 ] …. nums[nums.length -1]. Do not use loops.(java code)arrow_forward
- The following recursive method get Number Equal searches the array x of 'n integers for occurrences of the integer val. It returns the number of integers in x that are equal to val. For example, if x contains the 9 integers 1, 2, 4, 4, 5, 6, 7, 8, and 9, then getNumberEqual(x, 9, 4) returns the value 2 because 4 occurs twice in x. public static int getNumberEqual(int x[], int n, int val) { if (n< 0) ( return 0; } else { if (x[n-1) == val) { return getNumberEqual(x, n-1, val) +1; } else { return getNumber Equal(x, n-1, val); } // end if ) // end if } // end get Number Equal Demonstrate that this method is recursive by listing the criteria of a recursive solution and stating how the method meets each criterion.arrow_forwardGiven a long string use recursion to traverse the string and replace every vowel (A,E,I,O,U) with an @ sign. You cannot change the method header. public String encodeVowels(String s){ // Your code here }arrow_forwardWrite a static recursive method in Java called mRecursion that displays all of the permutations of the charactersin a string passed to the method as its argument. For example, the character sequence abc has thefollowing permutations: acb, bac, bca, cab, cba. Then Write a static method called getInput that get aninput string from the user and passed it to the mRecursion method written above in a method call.Please does so using what I already had //Get input from in to call recursive method //to display those char permutations public static String getInput ( ) { Scanner in = new Scanner(System.in); String combination = in.nextLine(); System.out.println("Enter string:); return stringComb; //Method to show permutations of a desired string// This only return 3 string combination for some reason static void myRecursion(String aString) { //isEmpty check if ( aString.length() == 0){…arrow_forward
- write a code in java (using recursion)arrow_forwarddef height(words, word): The length of a word is easy enough to define by tallying up its characters. Taking the road less traveled, we define the height of the given word with a recursive rule for the height of the given word to follow from the heights of two words whose concatenation it is. First, any character string that is not one of the actual words automatically has zero height. Second, an actual word that cannot be broken into a concatenation of two nonempty actual words has the height of one. Otherwise, the height of an actual word equals one plus the larger of the heights of the two actual words whose combined concatenation it can be expressed as. To make these heights unambiguous for words that can be split into two non-empty subwords in multiple ways, this splitting is done the best way that produces the tallest final height. Since the list of words is known to be sorted, you can use binary search (available as the function bisect_left in the bisect module) to quickly…arrow_forwardpublic class Main { public static void main(String[] args) { System.out.println("Your factorial is: " + factorial(9)); }} public static int factorial(int number) { if (number == 0) { return 1; } return number * factorial(number -1); } i need help fixing this code involving recursionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
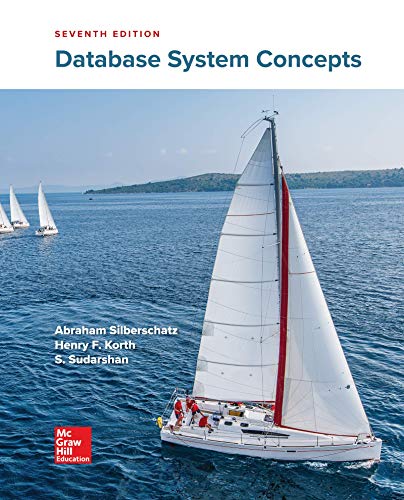
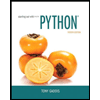
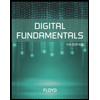
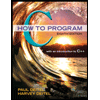
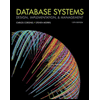
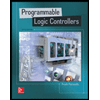