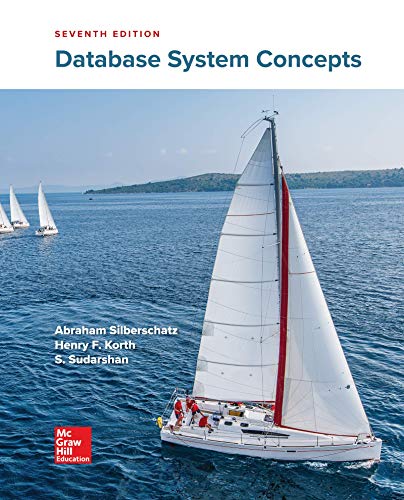
Write a static recursive method in Java called mRecursion that displays all of the permutations of the characters
in a string passed to the method as its argument. For example, the character sequence abc has the
following permutations: acb, bac, bca, cab, cba.
Then Write a static method called getInput that get an
input string from the user and passed it to the mRecursion method written above in a method call.
Please does so using what I already had
//Get input from in to call recursive method
//to display those char permutations
public static String getInput ( ) {
Scanner in = new Scanner(System.in);
String combination = in.nextLine();
System.out.println("Enter string:);
return stringComb;
//Method to show permutations of a desired string
// This only return 3 string combination for some reason
static void myRecursion(String aString) {
//isEmpty check
if ( aString.length() == 0){
System.out.println("Empty String: ");
}
for (int i = 0; i < comb.length(); i++) {
char n = aString.charAt(i);
String toPermute = aString.substring(0, i)+(aString.substring(i+1));
System.out.println(toPermute + ("" +n));
public static void main ( String[] args ) { String word; word = getInput(); myRecursive(word); }

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- John here Need correct Java code for the following with output "Welcome" returns 2arrow_forwardcreat a method in (java) that do the following: This will prompt the user for initial list of letters(a word).Then it will generate all permutations of these letters .Here it has to be mentioned that the permutations can also be of shorter length than the list of letters . For example, if the initial list of letters is "STOP", then "TOP" and "TOPS" are both valid permutations, but "STOPS" isn'tarrow_forwardWrite code in Java: -Must be recursive import java.util.*; import java.lang.*; import java.io.*; //*Analysis goes here* //*Design goes here* class AllPermutation { public static void displayPermutation(String s) { //*Code goes here* } public static void displayPermutation(String s1, String s2) { //*Code goes here* } } //*Driver class should not be changed* class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); AllPermutation.displayPermutation(input.nextLine()); } }arrow_forward
- basic java please Write a method starTheString that accepts a string of any size/length and a number. It should then return a new string that is two copies of the string with a set of stars between them equal to the number. For example, the call of starTheString("Hello", 5) o would return "*****Hello*****" 5 stars - Hello - 5 Stars starTheString ("Goodbye", 3) o would return "***Goodbye***" 3 stars – Goodbye - 3 Starsarrow_forwardWrite the following programs in Java: In the Converter class write the static method, bin2decimal(String bin). The parameter for this method is an unsigned (nonnegative) binary number represented by a string. The method returns the decimal equivalent for the binary parameter, as an integer.If the binary string is invalid (contains characters other than ‘0’ and ‘1’), the method returns -1. Convert from a binary (b) string with up to 16 digits to decimal (d) using the following formula:? = ?0 + ?1 × 2 + ?2 × 22 +⋯+ ?7 × 215where b0, b1, ?2, … ?7 are the binary digits in order of increasing significance. For example, 1101 is: 1 + 0×2 + 1×22 + 1×23 = 13 in decimal. Side note: You may NOT use the decode method of the Long wrapper class instead of this formula. In the Converter class write another static method, english2encrypted(String english). The parameter for this method is a word or sentence represented by a string. The word or sentence should only contain letters (no digits,…arrow_forwardWrite a Java application that calculates the sum of a series of integers that are passedto method sum() using a variable-length argument list. Test your method with severalcalls, each of which with different number of argumentsarrow_forward
- Given a long string use recursion to traverse the string and replace every vowel (A,E,I,O,U) with an @ sign. You cannot change the method header. public String encodeVowels(String s){ // Your code here }arrow_forwardjava Write a public static method named getStringReversed that takes in 1 argument of type String and returns the String in reverse order.arrow_forwardConsider the following recursive method in Java that determines ifa string is a palindrome (i.e., reads the same forward or backwards) or not: Public boolean isPalindrome ( String str ){If( str.length() ≤ 1 )return true;elsereturn ( str.charAt (0) == str.charAt( str.length() – 1 ) &&isPalindrome( str.substring(1..str.length() - 1)));} // end of isPalindrome a. Explain convincingly why the recurrence below for f(n), where n is the length of the string parameter str, is a correct expression for the number of primitive operationsrequired by a call isPalindrome.t(0) = Θ(1)t(1) = Θ(1)t(?) = t(? − 2) + Θ(1)b. Show that t(n) = Θ(n), solves the recurrence for t(n) in (a) above.arrow_forward
- A palindrome is a string that reads the same forward and backward. For example,“deed” and “level” are palindromes. Write an algorithm in pseudocode that testswhether a string is a palindrome. Implement your algorithm as a static method inJava. The method should call itself recursively and determine if the string is apalindrome.Write a main method that reads a string from the user as input and pass it to thestatic method. You need to validate the string and make sure it contains only letters(no digits or special characters are allowed). The main method should displaywhether the string is a palindrome or not. a) Read the string from the user and validate it.b) Create a method that calls itself recursively and returns whether thestring is a palindrome or not.c) Include a test table with at least the following test cases:1. Invalid input. For example, string contains a number or specialcharacter.2. A valid string that is a palindrome.3. A valid string that is not a palindrome.arrow_forwardPlease help me with the below using java. Please also comment the code (explai. What each li e is doing). Please make sure both codes are completed using recursionarrow_forwardGiven a string, write a method that returns the number of occurrences of substrings "baba" or "mama" in the input string recursively. They may overlap. Do not use any loops within your code. Do not use any regular expressions and methods such as matches, split, replaceAll. "Maa mana, Test case 1: countBabaMama ("aba babaa amama ma") 2 Test case 2: countBabaMama ("bababamamama") 4arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
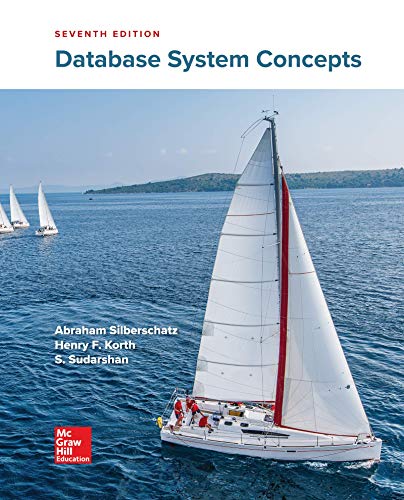
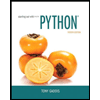
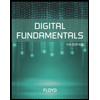
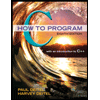
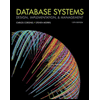
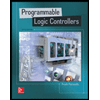