Write a recursive method that takes two strings str and forbidden and removes all characters from the forbidden string from str. JAVA import java.util.Scanner; /** * A recursive method that removes forbidden characters from a string. */ public class CleanUp { public static String clean(String str, String forbidden) { /* Your code goes here */ if (forbidden.contains(first)) { return /* code goes here */ } else { return /* code goes here */ } } public static void main(String[] args) { Scanner in = new Scanner(System.in); while (in.hasNext()) { String str1 = in.next(); String str2 = in.next(); System.out.println(clean(str1, str2)); } } }
Write a recursive method that takes two strings str and forbidden and removes all characters from the forbidden string from str.
JAVA
import java.util.Scanner;
/**
* A recursive method that removes forbidden characters from a string.
*/
public class CleanUp
{
public static String clean(String str, String forbidden)
{
/* Your code goes here */
if (forbidden.contains(first))
{
return /* code goes here */
}
else
{
return /* code goes here */
}
}
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
while (in.hasNext())
{
String str1 = in.next();
String str2 = in.next();
System.out.println(clean(str1, str2));
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

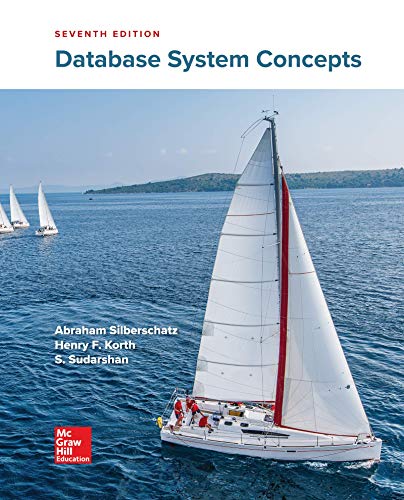
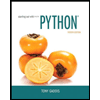
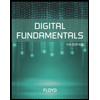
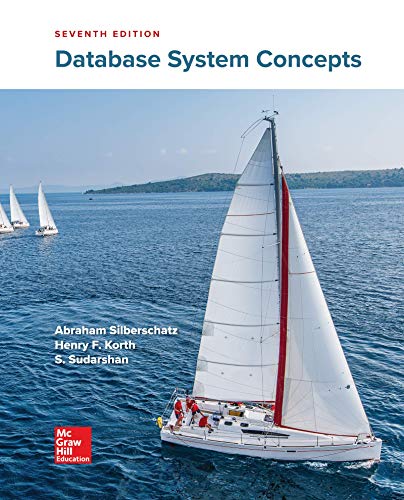
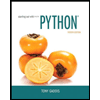
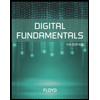
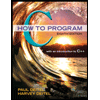
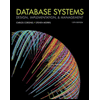
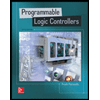