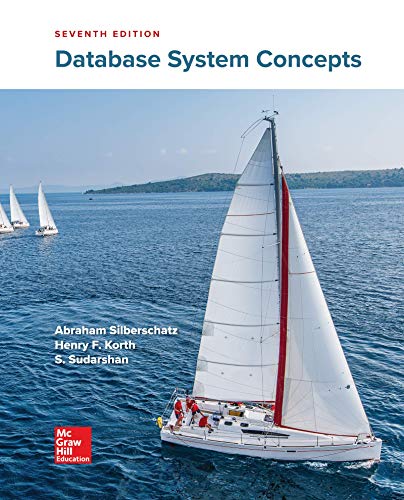
Needs to be coded in JAVA.
01 - Recursive Combinations
Compute the combinations of n things taken k at a time using both iterative and recursive methods. Both methods should use only int types for mathematical operations. If the iterative method continues to mock you, that is ok, the recursive method should not.
You should modify the attached code for your submission.
Reference: https://en.wikipedia.org/wiki/Combination
public class Combination {
private static boolean useFact;
/**
* Computes some combinations.
*
* @param args unused
*/
public static void main(String[] args) {
for (int i = 0; i < 2; i++) {
useFact = (i % 2 == 0);
System.out.println(useFact ? "FACTORIAL METHOD" : "RECURSIVE METHOD");
System.out.println();
combination(2, 2);
combination(3, 2);
combination(4, 3);
combination(10, 2);
combination(52, 5);
combination(60, 4);
combination(75, 3);
combination(100, 4);
System.out.println();
}
}
/**
* A method which switches between the two combination methods
* @param n the number of things
* @param k how many to choose
*/
public static void combination(int n, int k) {
System.out.print(n + " choose " + k + " = ");
try {
if (useFact)
System.out.println(combinationFactorial(n, k));
else
System.out.println(combinationRecursive(n, k));
} catch (ArithmeticException ex) {
System.out.println("LOL!");
}
}
/**
* Computes the factorial of n. Try not to be jealous of glorious
* for loop.
*
* @param n the number whose factorial to compute
* @return n!
*/
private static int fact(int n) {
int prod = 1;
for (int i = 1; i <= n; prod *= i++)
;
return prod;
}
/**
* Computes the number of ways to take n things k at a time
*
* @param n things to choose from
* @param k in a group
* @return the number of ways to take n things k at a time
*/
private static int combinationFactorial(int n, int k) {
return 0;
}
/**
* Computes the number of ways to take n things k at a time
*
* @param n things to choose from
* @param k in a group
* @return the number of ways to take n things k at a time
*/
public static int combinationRecursive(int n, int k) {
return 0;
}
}

Step by stepSolved in 2 steps with 1 images

- 28Recursive methods must always contain a path that does not contain a recursive call. T OR Farrow_forwardsolve q7 only pleasearrow_forwardAg 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forward
- Complete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardtask-5 Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forwardJava - Write an iterative method that calculates the SUM of all integers between 1 and a given integer N (input into the method). Write a corresponding recursive solution. (return answer, don’t print)arrow_forward
- Finish the TestPlane class that contains a main method that instantiates at least two Planes. Add instructions to instantiate your favorite plane and invoke each of the methods with a variety of parameter values to test each option within each method. To be able to test the functionality of each phase, you will add instructions to the main method in each phase.arrow_forwardtask_6 Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forwardAlert dont submit AI generated answer. Write Java program with a recursive method called evenfact(N) which takes in a number N and returns the factorial of the even numbers between the given number N and 2.arrow_forward
- Exercise 1Write a Java method random that generates and returns a random number between 14 and 59(using SecureRandom). Test your method several times using a main functionarrow_forwardPlease help me this using recursion and java. Create a sierpenski triangle. And please comment the code. Do not create the game using hashtaarrow_forwardI want solution with steparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
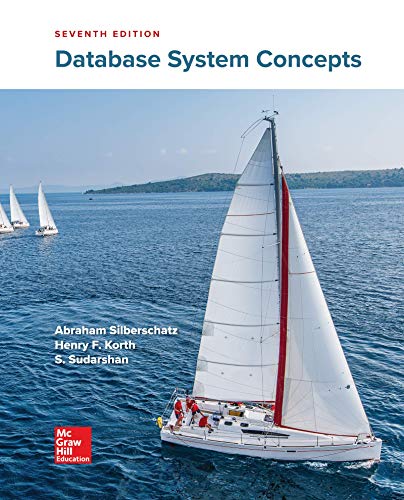
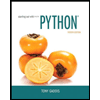
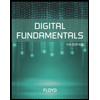
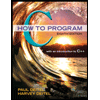
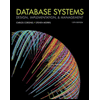
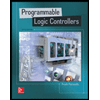