Input Your output Expected output Hello Reverse of "Hello" is "olleH". Reverse of "Hello" is "olleH".
import java.util.Scanner;
public class LabProgram {
// Recursive method to reverse a string
public static String reverseString(String str) {
// Base case: if the string is empty or has only one character, return the string as is
if (str.isEmpty() || str.length() == 1) {
return str;
} else {
// Recursive step: move the first character to the end and reverse the remaining substring
return reverseString(str.substring(1)) + str.charAt(0);
}
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String input, result;
input = scnr.nextLine();
// Call the reverseString() method
result = reverseString(input);
// Output the result
System.out.printf("Reverse of \"%s\" is \"%s\".%n", input, result);
}
}


Step by step
Solved in 4 steps with 2 images

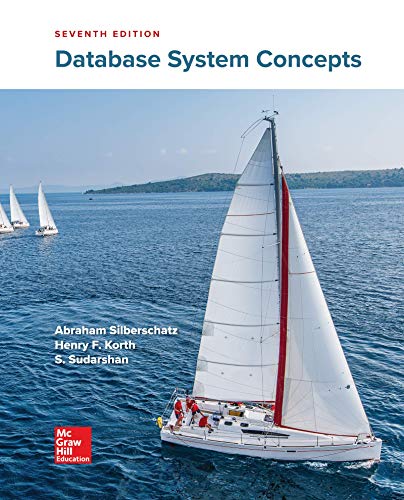
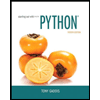
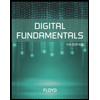
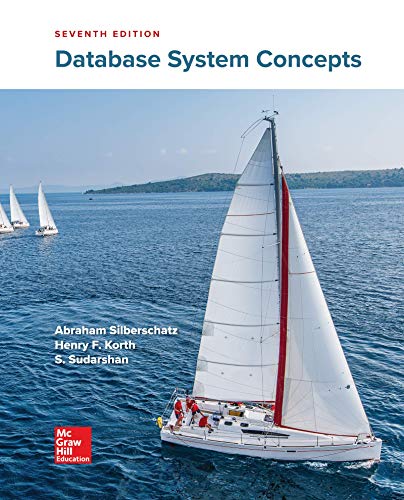
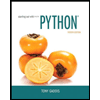
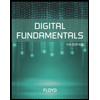
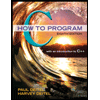
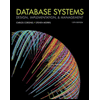
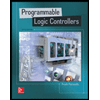