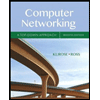
Directions:
Typing make at the command line should create executable named bitflip.
Only bitflip.c and Makefile are included in the input unless permission is given for any other files.
Program should have consistent indentation.
Code should be readable with good variable and function names.
No use of "break or continue statements" except in a switch.
Program must exit with a return code of 0 on success and an error code in other cases.
The program used getopt to parse the command line.
Whenever an error occurs on the command line the user is given the usage statement and an appropriate error message if needed.
If the -o option is used the output will go to a file named as specified without changing the filename at all otherwise it will go to stdout.
-e option works as specified using bitwise operations.
-f option works as specified using bitwise operations.
-a option works as specified using bitwise operations.
-s option works as specified using bitwise operations.
Program outputs only the original value if none of the bitwise options are included.
Program should work as specified with no additional changes or output.
Write a C program called bitflip to run on ocelot which will flip bits in an unsigned integer using the binary representation of the number. The user should input the original integer value between 1 and 20000 inclusive on the command line as well as the desired options. Use an unsigned integer type uint16_t. Output is to the screen or to a file. You must use only bitwise operators for this program. You can shift bits and or use the logical bitwise operators.
usage: bitflip [-e] [-f] [-a] [-s] [-o outputfilename] intval
Output the new integer number(s) depending on which options are requested (all aperations done on the original intval). Do not use any break or continue statements other than in the switch.
- You should use getopt for this program to identify the options.
- The program will always print the starting value on the first line of output and each other value on a new line, clearly identifying it.
- If the -e option is selected then flip all even bits.
- If the -f option is selected then flip all odd bits.
- If the -a option is selected then flip all bits.
- If the -s option is selected then take the bits and switch them around from right to left. (The rightmost bit becomes the leftmost bit.
- If the -o option is selected then print the output to an output file instead of to the screen.
- If an option is included more than once, you can ignore duplicates.
Code should be nicely indented and commented.
Create a simple Makefile to compile your program into an executable called bitflip.
A sample of a run of the program could be something like this:
jdoe@ocelot:~/ 135% bitflip -s -a -e -f 391
Value: 391
Even bits flipped: 21714
Odd bits flipped: 43821
All bits flipped: 65144
Switched all bits: 57728

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- Computer Science C++ Create a text file "input.txt" with a certain amount of integers (you decide how many). Write a program that reads these numbers from the file, adds them, and when you have reached the end of the file, calculates the average of these numbers. Print a message and the average to the console. Code this program twice, demonstrating the two methods to detect the end of the file, part A: reading a value from Instream and storing it (boolean expression) in the while loop part B: using the eof() member functionarrow_forwardcan you do it pythonarrow_forwardUsing a Counter-Controlled whileLoop Summary In this lab, you use a counter-controlled while loop in a Java program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the file named Multiply.java is open. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking Run. Record the output of this program.arrow_forward
- Java Program ASAP ************This program must work in hypergrade and pass all the test cases.********** Please give me a new program becausse when I upload the program to hypergrade it does not pass the test cases. It says 1 out of 4 passed. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are! Everyone was impressed. You'll do well to continue in…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The text files are located in Hypergrade. Also for test case 1 first display Please enter a string to convert to Morse code:\n then you press Enter it should print out \n. Then for test case 2 it should display Please enter a string to convert to Morse code:\n then you type abc it should print out .- -... -.-. \n For test case 3 first display Please enter a string to convert to Morse code:\n then you type This is a sample string 1234.ENTER it should print put - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n. This program down below does not pass the test cases as shown in the screenshot I have provided the correct test case as a screenshot too. Please modify it or create a new program so it paases the test cases. Thank you! For test case 1 it wants only Please enter a string to convert to Morse…arrow_forwardIn this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forward
- The provided file has syntax and/or logical errors. Determine the problem and fix the program. // Program asks user to enter password // If password is not "home", "lady" or "mouse" // the user must re-enter the password using static System.Console; class DebugFive1 { static void Main() { cont string PASS1 = "home"; cont string PASS2 = "lady"; cont string PASS3 = "mouse"; string password; Write("Please enter your password "); password = Console.ReadLine(); while(password != PASS1 || password != PASS2 || password != PASS3) WriteLine("Invalid password. Please enter again. "); password = ReadLine(); WriteLine("Valid password"); } }arrow_forwardCan you help me write the following C++ program: Add a search command to the file viewer created. Thiscommand asks the user for a string and finds the first line that containsthat string. The search starts at the first line that is currently displayedand stops at the end of the file. If the string is found, then the file willbe scrolled down so that when it is redisplayed, the line that contains thestring is the first line at the top of the window.If the string is not found, the program prints the following error messageat the top of the screen:ERROR: string X was not foundwhere X is replaced by the string entered by the user. In that case, theprogram redisplays the lines that the user was viewing before the searchcommand was executed.Modify the program as little as possible. Make sure you assign new responsibilities to the most appropriate components. Use the C++ string operation find. The file viewer code is given below: // FileViewer.cpp #include "FileViewer.h" using namespace…arrow_forward1. Identify the following parameters to complete the specification above. Input parameters? Output parameters? Constant parameters? 2. Attach the input and output of a successful execution of your program below (Hint:Use the snipping tool to take screenshots):arrow_forward
- Using Java PrintWriter, write a program that prompts the user for information and saves it to a text file File name is musicians.txt Use this information below: George,Thoroughgood,54321 Ludwig,Van Beethoven,90111 Edward,Van Halen,12345arrow_forwardIn this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. The source code file already contains the necessary assignment and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie.arrow_forwardCISP 1010, Lab 3, Arrays and C-Strings Introduction This application creates Mad Lib™ haikus based upon user input. Detailed Information The application reads three-line haikus into a two-dimensional array of characters (an array of C-strings, not C++ string objects) from an input file called haikus.dat. Do not ask the user for this file name. Just open it and read its contents into a two-dimensional array. A sample input file with two haikus is shown below. Flowers sit in * Shades of the * tree near * in sunset When begins the * And the * Crocus blooms * any thing The asterisk will actually be in the file. They indicate where words that the user enters go in the haiku. The asterisk on the first line will be replaces by a one syllable noun and on the second line will be replaced by a two syllable adjective. These two askterisks will never be the first character of the line. The asterisk on the third line will always be the first character on the line and will be replaced by a gerund (a…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
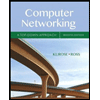
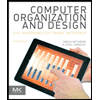
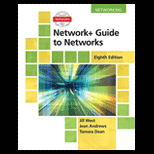
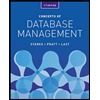
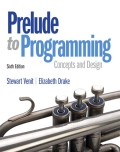
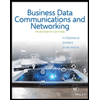