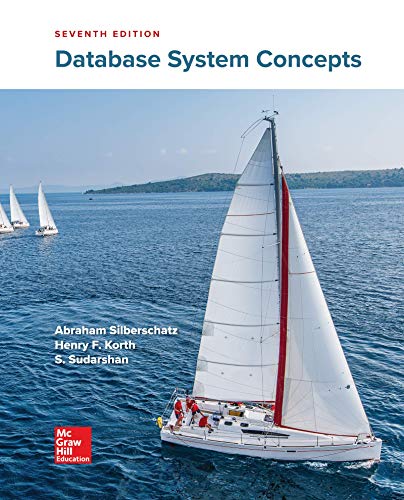
Can you help me write the following C++ program:
Add a search command to the file viewer created. This
command asks the user for a string and finds the first line that contains
that string. The search starts at the first line that is currently displayed
and stops at the end of the file. If the string is found, then the file will
be scrolled down so that when it is redisplayed, the line that contains the
string is the first line at the top of the window.
If the string is not found, the program prints the following error message
at the top of the screen:
ERROR: string X was not found
where X is replaced by the string entered by the user. In that case, the
program redisplays the lines that the user was viewing before the search
command was executed.
Modify the program as little as possible. Make sure you assign new responsibilities to the most appropriate components. Use the C++ string operation find.
The file viewer code is given below:
// FileViewer.cpp
#include "FileViewer.h"
using namespace std;
void FileViewer::display()
{
const string long_separator(50, '-');
const string short_separator(8, '-');
system(clear_command);
if (!error_message_.empty()) {
cout << "ERROR: " + error_message_ << endl;
error_message_.clear();
}
string file_name = buffer_.file_name();
if (file_name.empty())
cout << "<no file opened>\n";
else
cout << file_name << endl;
cout << long_separator << endl;
buffer_.display();
cout << long_separator << endl;
cout << " next previous open quit\n";
cout << short_separator << endl;
}
void FileViewer::execute_command(char command, bool & done)
{
switch (command) {
case 'n': {
buffer_.move_to_next_page();
break;
}
case 'o': {
cout << "file name: ";
string file_name;
getline(cin, file_name);
if (!buffer_.open(file_name))
error_message_ = "Could not open " + file_name;
break;
}
case 'p': {
buffer_.move_to_previous_page();
break;
}
case 'q': {
done = true;
break;
}
}
}
void FileViewer::run()
{
cout << "Window height? ";
cin >> window_height_;
cin.get(); // '\n'
cout << '\n';
buffer_.set_window_height(window_height_);
bool done = false;
while (!done) {
display();
cout << "command: ";
char command;
cin >> command;
cin.get(); // '\n'
execute_command(command, done);
cout << endl;
}
}

Step by stepSolved in 2 steps

- Creates a sales receipt, displays the receipt entries and totals, and saves the receipt entries to a file .Prompt the user to enter the - Item Name Item Quantity Item Price Display the item name, the quantity, and item price, and the extended price (Item Quantity multiplied by Item Price) after the entry is made Save the item name, quantity, item price, and extended price to a file When you create the file, prompt the user for the name they want to give the file Separate the items saved with commas Each entry should be on a separate line in the text file Ask the user if they have more items to enter Once the user has finished entering items Close the file with the items entered Display the sales total If the sales total is more than $100 Calculate and display a 10% discount Calculate and display the sales tax using 8% as the sales tax rate The sales tax should be calculated on the sales total after the discount Display the total for the sales receiptarrow_forwardIn pythonarrow_forwardPlease help with my C++Specifications • For the view and delete commands, display an error message if the user enters an invalid contact number. • Define a structure to store the data for each contact. • When you start the program, it should read the contacts from the tab-delimited text file and store them in a vector of contact objects. •When reading data from the text file, you can read all text up to the next tab by adding a tab character ('\t') as the third argument of the getline() function. •When you add or delete a contact, the change should be saved to the text file immediately. That way, no changes are lost, even if the program crashes laterarrow_forward
- In Python Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents…arrow_forwardDescriptionWrite a program to compute average grades for a course. The course records are in a single file and are organized according to the following format: each line contains a student's first name, then one space, then the student's last name, then one space, then some number of quiz scores that, if they exist, are separated by one space. Each student will have zero to ten scores, and each score is an integer not greater than 100. Your program will read data from this file and write its output to a second file. The date in the output file will be nearly the same as the data in the input file except that you will print the names as last-name, first-name; each quiz score, and there will be one additional number at the end of each line:the average of the student's ten quiz scores.Both files are parameters. You can access the name of the input file with argv[1]. and the name of the output file with argv[2].The output file must be formatted as described below: 1. First and last names…arrow_forwardhere is a text file containing the details of several invoices details. Each invoice uses 3 lines of the file. The first of the three lines is a string giving the date of the invoice, for example “1/5/2021” or “2/6/2021”. The second of the three lines contains double giving the amount, the third line is boolean indicating whether it is paid or not. However, the very first line of the file is an integer number, which says how many invoices are given in the file in the lines which follow it (i.e. how many records will follow). Text file example for two vehicles “invoices.txt” 2 1/5/2021 50.0 true 2/6/2021 20.0 false Write code for a method named processTextFile() which will open the file named ‘invoices.txt’, from which it will read the data of invoices. It will create Invoice objects using this data by calling the constructor that takes the parameters (date, amount, paid) , placing them into an ArrayList invoiceList.arrow_forward
- A bucket list is a collection of goals, dreams, and aspirations that you would like to accomplish within your lifetime. The purpose of this assignment is to develop a program that allows the user to manage their personal bucket list and save it to an XML file bucketlist.xml. For full credit, run the program and create a file with at least three entry elements. Submit a document containing a copy of the source file, a screenshot of the program in action, and the contents of the XML file. File Structure Below is the basic structure (i.e. schema) for the XML file. You may add additional elements. <bucketList> <entry> <rank></rank> <description></description> <status></status> </entry> </bucketList> Note: Before running the program, manually create an XML input file with at least one entry element that contains values in each child element. GUI Requirements At a minimum, your GUI must meet the below requirements. No minimize…arrow_forwardCreate a list of 5 words, phrases and company names commonly found in phishing messages. Assign a point value to each based on your estimate of its likelihood to be in a phishing message (e.g., one point if it's something likely, two points if modereratly, or three points if highly likely). Write an application that scans a file of text for these terms and phrases. For each occurance of a keyword or phrase within the text file, add the assigned point value to the total points for the number of occurences and the point total. Show the point total for the entire message. (Write the program in Java)arrow_forwardSuppose a csv file contains three comma-separated values (strings) on each line (see the leftcolumn of the example table below). Assume, each line of values are, respectively, thewidth, height, and depth of a box. Write a program called box.py and add thefollowing functions to this program.• read_file() takes a csv filename as a parameter that contains the widths, heights, anddepths of all boxes. This function must read the content of the file, store these values ina 2-D list as shown in the right column of the example table below, and return this list.All strings must be converted to integers before they are stored in the list. Example:csv data 2-D list[[15, 6, 3],[3, 7, 4],[6, 9, 3],[15, 6, 11],[6, 5, 5],[13, 10, 9],[9, 10, 3],[14, 5, 4],[4, 6, 11],[12, 10, 9]]• Add another function called max_volume() to this program that takes this 2-D list as aninput parameter. Each item in that list contains 3 integers representing the dimensions ofa box (i.e., width, height, depth). This…arrow_forward
- python please! the first answer i received didn't check out. it is graded by a computer on autolab. im so confusedarrow_forward1. Create a file that contains 20 integers or download the attached file twenty-integers.txttwenty-integers.txt reads:12 20 13 45 67 90 100 34 56 89 33 44 66 77 88 99 20 69 45 20 Create a program that: 2. Declares a c-style string array that will store a filename. 3. Prompts the user to enter a filename. Store the file name declared in the c-string. 4. Opens the file. Write code to check the file state. If the file fails to open, display a message and exit the program. 5. Declare an array that holds 20 integers. Read the 20 integers from the file into the array. 6. Write a function that accepts the filled array as a parameter and determines the MAXIMUM value in the array. Return the maximum value from the function (the function will be of type int). 7. Print ALL the array values AND print the maximum value in the array using a range-based for loop. Use informational messages. Ensure the output is readable.arrow_forwardYou have more flexibility to implement your own function names and logic in these programs. The data files you need for this assignment can obtained from:Right-click and "Save Link As..." HUGO_genes.txt (Links to an external site.) chr21_genes.txt (Links to an external site.) Create an output directory inside your assignment4 directory called "OUTPUT" for result files, so that they will not mix with your programs. Output from your programs will be here! Your program must implement command line options for the infiles it must open, but for testing purposes it should run by default, so if no command line option is passed at the command line, the program will still run. This will help in the grading of your program. Create a Python Module called called my_io.py - You can use the same code from assignment 3's solution. Put this my_io.py Module in a subdirectory named assignment4 inside your assignment4 top-level directory (see the tree below, and see Lecture 7 on how to implement a…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
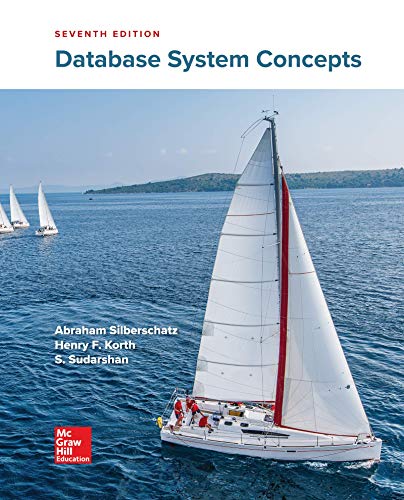
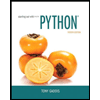
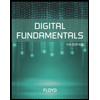
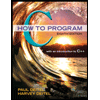
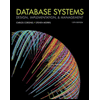
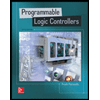