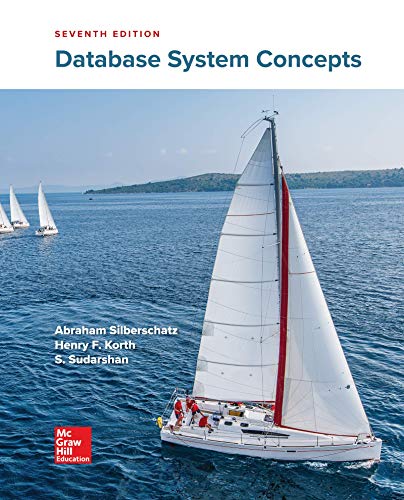
DESCRIBE WHAT THE FOLLWING JAVA CODE DOES:
public class Main{
public static void main(String[] args) {
new MyFrame();
}
}
import javax.swing.*;
public class MyFrame extends JFrame{
MyPanel panel;
MyFrame(){
panel = new MyPanel();
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.add(panel);
this.pack();
this.setLocationRelativeTo(null);
this.setVisible(true);
}
}
import java.awt.*;
import javax.swing.*;
public class MyPanel extends JPanel{
//Image image;
MyPanel(){
//image = new ImageIcon("sky.png").getImage();
this.setPreferredSize(new Dimension(500,500));
}
public void paint(Graphics g) {
Graphics2D g2D = (Graphics2D) g;
//g2D.drawImage(image, 0, 0, null);
g2D.setPaint(Color.blue);
g2D.setStroke(new BasicStroke(5));
g2D.drawLine(0, 0, 500, 500);
//g2D.setPaint(Color.pink);
//g2D.drawRect(0, 0, 100, 200);
//g2D.fillRect(0, 0, 100, 200);
//g2D.setPaint(Color.orange);
//g2D.drawOval(0, 0, 100, 100);
//g2D.fillOval(0, 0, 100, 100);
//g2D.setPaint(Color.red);
//g2D.drawArc(0, 0, 100, 100, 0, 180);
//g2D.fillArc(0, 0, 100, 100, 0, 180);
//g2D.setPaint(Color.white);
//g2D.fillArc(0, 0, 100, 100, 180, 180);
//int[] xPoints = {150,250,350};
//int[] yPoints = {300,150,300};
//g2D.setPaint(Color.yellow);
//g2D.drawPolygon(xPoints, yPoints, 3);
//g2D.fillPolygon(xPoints, yPoints, 3);
//g2D.setPaint(Color.magenta);
//g2D.setFont(new Font("Ink Free",Font.BOLD,50));
//g2D.drawString("U R A WINNER! :D", 50, 50);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- How to fix this error: Error: Main method not found in class ExpatConsultant, please define the main method as: public static void main(String[] args) or a JavaFX application class must extend javafx.application.Application public ExpatConsultant(String dob, String sector, double skillPrice, double taxRate, double airfare) { super(); String[] parts = dob.split("/"); super.setDob(Integer.parseInt(parts[1]), Integer.parseInt(parts[0]), Integer.parseInt(parts[2])); this.skillPrice = skillPrice; this.permitTax = skillPrice * taxRate; this.airfare = airfare; this.sector = sector; this.id = getNextId(); } public String getSector() { return sector; } public String getContact() { return "Reg. Expatriate#" + id; } public int getNextId() { id = nextId; nextId++; return id; } public int getId() { return id; } public double getPay() { double earnings =…arrow_forwardCode for Activity2PayStub: // Activity2PayStub.java import java.util.Scanner; public class Activity2PayStub{ // constants public static final double OVERTIME_RATE = 1.5; public static final double SS_WITHHOLDING = .1; public static final double FEDERAL_TAX = .2; // fields private String name, ssn; private int regHours, overHours; private double hourlyRate, regPay, overRate, overPay, grossPay, ssWith, fedTax, netPay; public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); // create an object of Activity2PayStub class Activity2PayStub a2ps = new Activity2PayStub(); // get inputs a2ps.getInput(keyboard); // compute payments a2ps.calculate(); // display information a2ps.printPayStub(); } /** * method that takes inputs of name, ssn, regular hours, overtime hours * and hourly rate and set the fields to the values input by the user */ public void getInput(Scanner keyboard) {…arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedListNode { private Object date; private LinkedListNode next; // Constructor: public LinkedListNode (Object data) // You also need to define the getter and setter: public LinkedListNode getNext() public void setNext (LinkedListNode next) public Object getData() public void setData(Object data) }arrow_forward
- Question & instructions can be found in images please see images first Starter code import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.text.DecimalFormat; public class GPACalculator extends JFrame{private JLabel gradeL, unitsL, emptyL; private JTextField gradeTF, unitsTF; private JButton addB, gpaB, resetB, exitB; private AddButtonHandler abHandler;private GPAButtonHandler cbHandler;private ResetButtonHandler rbHandler;private ExitButtonHandler ebHandler; private static final int WIDTH = 400;private static final int HEIGHT = 150; private static double totalUnits = 0.0; // total units takenprivate static double gradePoints = 0.0; // total grade points from those unitsprivate static double totalGPA = 0.0; // total GPA (gradePoints / totalUnits) //Constructorpublic GPACalculator(){//Create labelsgradeL = new JLabel("Enter your grade: ", SwingConstants.RIGHT);unitsL = new JLabel("Enter number of units: ", SwingConstants.RIGHT);emptyL = new…arrow_forwardimport bridges.base.ColorGrid;import bridges.base.Color;public class Scene {/* Creates a Scene with a maximum capacity of Marks andwith a background color.maxMarks: the maximum capacity of MarksbackgroundColor: the background color of this Scene*/public Scene(int maxMarks, Color backgroundColor) {}// returns true if the Scene has no room for additional Marksprivate boolean isFull() {return false;}/* Adds a Mark to this Scene. When drawn, the Markwill appear on top of the background and previously added Marksm: the Mark to add*/public void addMark(Mark m) {if (isFull()) throw new IllegalStateException("No room to add more Marks");}/*Helper method: deletes the Mark at an index.If no Marks have been previously deleted, the methoddeletes the ith Mark that was added (0 based).i: the index*/protected void deleteMark(int i) {}/*Deletes all Marks from this Scene thathave a given Colorc: the Color*/public void deleteMarksByColor(Color c) {}/* draws the Marks in this Scene over a background…arrow_forwardimport java.util.*;public class MyLinkedListQueue{ class Node { public Object data; public Node next; private Node first; public MyLinkedListQueue() { first = null; } public Object peek() { if(first==null) { throw new NoSuchElementException(); } return first.data; } public void enqueue(Object element) { Node newNode = new newNode(); newNode.data = element; newNode.next = first; first = newNode; } public boolean isEmpty() { return(first==null); } public int size() { int count = 0; Node p = first; while(p ==! null) { count++; p = p.next; } return count; } } } Hello, I am getting an error message when I execute this program. This is what I get: MyLinkedListQueue.java:11: error: invalid method declaration; return type required public MyLinkedListQueue() ^1 error I also need to have a dequeue method in my program. It is very similar to the enqueue method. I'll…arrow_forward
- JAVA CODE: check outputarrow_forwardpackage edu.umsl.casting;public class Main {public static void main(String[] args) {String string = "myString";Object object = new Object();object = string; // What type of casting?string = (String) object; //What type of casting?}} List the two types of casting in the above code. Group of answer choices Implicit and Explicit Generic and Exact Autoboxing and Autounboxing Closed and Openarrow_forwardJava Question- Convert this source code into a GUI application using JOptionPane. Make sure the output looks similar to the following picture. Thank you. import java.util.Calendar;import java.util.TimeZone;public class lab11_5{public static void main(String[] args){//menuSystem.out.println("-----------------");System.out.println("(A)laska Time");System.out.println("(C)entral Time");System.out.println("(E)astern Time");System.out.println("(H)awaii Time");System.out.println("(M)ountain Time");System.out.println("(P)acific Time");System.out.println("-----------------"); System.out.print("Enter the time zone option [A-P]: "); //inputString tz = System.console().readLine();tz = tz.toUpperCase(); //change to uppercase //get current date and timeCalendar cal = Calendar.getInstance();TimeZone.setDefault(TimeZone.getTimeZone("GMT"));System.out.println("GMT/UTC:\t" + cal.getTime()); switch (tz){case "A":tz =…arrow_forward
- public class OfferedCourse extends Course { // TODO: Declare private fields // TODO: Define mutator methods - // setInstructorName(), setLocation(), setClassTime() // TODO: Define accessor methods - // getInstructorName(), getLocation(), getClassTime() } import java.util.Scanner; public class CourseInformation { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Course myCourse = new Course(); OfferedCourse myOfferedCourse = new OfferedCourse(); String courseNumber, courseTitle; String oCourseNumber, oCourseTitle, instructorName, location, classTime; courseNumber = scnr.nextLine(); courseTitle = scnr.nextLine(); oCourseNumber = scnr.nextLine(); oCourseTitle = scnr.nextLine(); instructorName = scnr.nextLine(); location = scnr.nextLine(); classTime = scnr.nextLine(); myCourse.setCourseNumber(courseNumber); myCourse.setCourseTitle(courseTitle);…arrow_forwardimport java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardJava Given main(), complete the SongNode class to include the printSongInfo() method. Then write the Playlist class' printPlaylist() method to print all songs in the playlist. DO NOT print the dummy head node.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
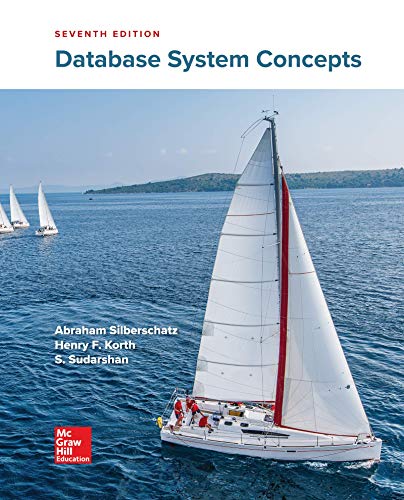
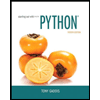
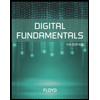
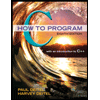
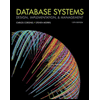
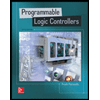