Please fix errors for the java code below. The program is a t square fractal (image below)
Please fix errors for the java code below. The program is a t square fractal (image below)
import javax.swing.*;
import java.awt.*;
import java.awt.geom.*;
//main class
public class SquareFractal {
public static void main(String[] args)
{
FractalFrame tframe = new FractalFrame();
tframe.setTitle("T Square Fractal");
tframe.setVisible(true);
}
}
//JFrame extended in fractal class
class T_Fractal extends JFrame
{
private JPanel panel;
private int twidth, theight;
private int x = 200;
private int limit = 15;
public T_Fractal()
{
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//default height and width
final int Width = 1000;
final int Height = 1000;
setSize(Width, Height);
setLocation(200,50);
panel = new JPanel();
Container t_content = getContentPane();
t_content.add(panel, "Center");
}
public void paint(Graphics graphics) {
super.paint(graphics);
Graphics2D g2 = (Graphics2D) graphics;
twidth = getWidth();
theight = getHeight();
graphics.setColor(Color.WHITE);
int w = (twidth/2)-(x/2);
int h = (theight/2)-(x/2);
graphics.fillRect(w, h, x, x);
if(limit > 0) drawSquare(w,h,graphics);
JOptionPane.showMessageDialog(null, "Fractal Completed");
}
private void drawSquare(int w, int h, Graphics graphics) {
int n = 0;
graphics.fillRect(w-(x/2), h-(x/2), x/2, x/2);
drawSquare(w-(x/2), h-(x/2), graphics, n, 0, x/2);
graphics.fillRect(w+x, h-(x/2), x/2, x/2);
drawSquare(w+x, h-(x/2), graphics, n, 1, x/2);
graphics.fillRect(w+x, h+x, x/2, x/2);
drawSquare(w+x, h+x, graphics, n, 2, x/2);
graphics.fillRect(w-(x/2), h+x, x/2, x/2);
drawSquare(w-(x/2), h+x, graphics, n, 3, x/2);
}
private void drawSquare(int w, int h, Graphics graphics, int n, int origin, int size) {
if(n == limit){
return;
}
n++;
origin = (origin+2)%4;
if(origin != 0) {
graphics.fillRect(w-(size/2), h-(size/2), size/2, size/2);
drawSquare(w-(size/2), h-(size/2),graphics,n,0,size/2);
}
if(origin != 1) {
graphics.fillRect(w+size, h-(size/2), size/2, size/2);
drawSquare(w+size, h-(size/2),graphics,n,1,size/2);
}
if(origin != 2) {
graphics.fillRect(w+size, h+size, size/2, size/2);
drawSquare(w+size, h+size,graphics,n,2,size/2);
}
if(origin != 3) {
graphics.fillRect(w-(size/2), h+size, size/2, size/2);
drawSquare(w-(size/2), h+size,graphics,n,3,size/2);
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

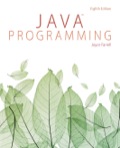
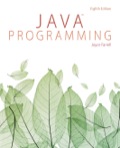