Instructions Today's task is to subclass the TallyCounter class once to make a really useful and "pretty" tally counter. This time you will not only be able to count up, but also decrease the count when someone leaves the room. Not only that, but the updated tostring will output a bit more useful information. The Museum of Natural History needs your help once more. They would like you to help pretty up their tally counter (call it a PrettyTally) and make it more useful in a variety of situations. They need you to subclass the original TallyCounter. They need you to modify it in two ways. First, when they instantiate the PrettyTally, they'd like to specify what they are tallying by passing a string to the constructor that names what they are tallying. Also, they would like to be able to allow for decreasing the count within an exhibit with the use of a decrement method. You will subclass the TallyCounter class and implement a new tally counter called PrettyTally which will meet those requirements. Details for this class are given below. Recall: The TallyCounter keeps track of the count. A TallyCounter has two methods: the Increment() method and the get() method. These methods do the following - Increment(): This method increases the total count by one. This method is used when a new visitor comes to visit Gus. One more visitor, one more push of the TallyCounter button to Increment the count by one. - get(): This method returns an integer count of the number of visitors that have been by to see Gus so far. Details Input In the file PrettyTally.java you will subclass the TallyCounter class. You will add the functionality to decrement the counter. Just like the TallyCounter, PrettyTally must keep track of the count You will create the necessary field members for all needs of the class. PrettyTally constructor will expect a new String parameter tallyObject to keep track of what is being tallied. Processing A PrettyTally will have all the same functionality of the TallyCounter, but it will also have a decrement() method. This method decreases the total count by one. If a visitor chooses to leave an exchibit, we can decrement the count by one. The form of the new tostring should be the current tally count and the name of the objects being tallied, separated by a space. You will need to set up the PrettyTally subclass, including any new field members, constructor and method. The main method of PoDJava will instantiate a new PrettyTally and read in methods to cal.
Java.
Tally counter that increases and decreases. Refer to screenshot.
Here is some code (class PoD):
import java.util.*;
public class PoD {
public static void main (String [] args ) {
Scanner in = new Scanner( System.in );
PrettyTally tally = new PrettyTally(in.next());
while (in.hasNext())
{
String nextTask = in.next();
if (nextTask.equals("increment"))
{
tally.increment();
}
else if (nextTask.equals("toString"))
{
System.out.println(tally);
}
else if (nextTask.equals("decrement"))
{
tally.decrement();
}
}
in.close();
}
}
Class PrettyTally:
public class PrettyTally extends TallyCounter{
}
Class TallyCounter
import java.util.*;
public class TallyCounter {
protected int count=0;
public TallyCounter()
{
this.count = 0;
}
public void increment()
{
this.count++;
}
public int get()
{
return this.count;
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

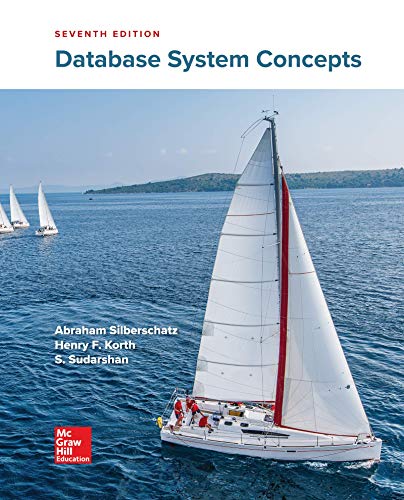
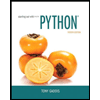
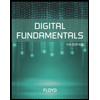
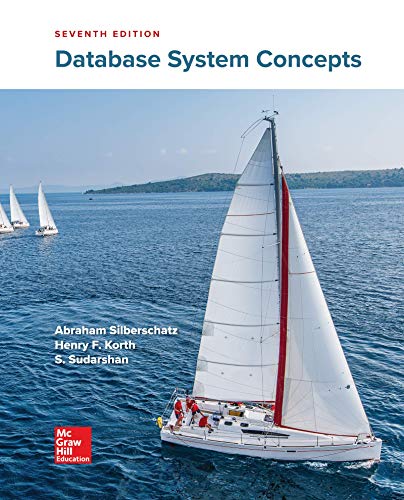
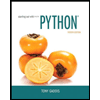
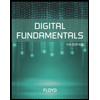
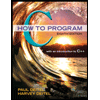
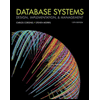
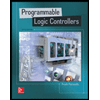