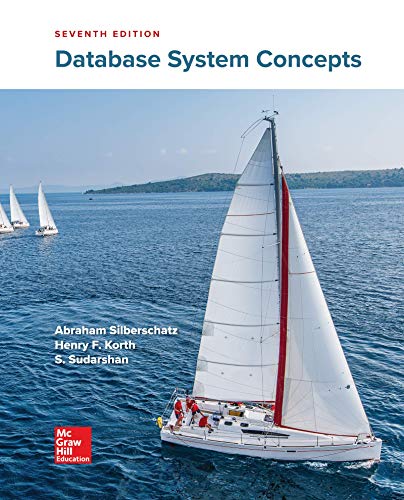
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
- Define a class called Player that implements Comparable <Player> with health and name attributes and has getter/setter methods to retrieve/access them. Define the appropriate constructors and give it a useful toString() method.
- Define a class called LinkedList with an inner nested class Node.
- The Node class has private attributes(Player player; Node next;). Define the appropriate constructor and toString() methods.
- Class LinkedList has two attributes (Node head and int size).
- Define the following LinkedList methods:
- insert(Player p); remove(Player p); toString();
- NOTE: your insert() method must be ordered. This means that your newly added Node should be placed in an ordered fashion such that you resulting linked list is ALWAYS sorted by the players’s health.
For example:
- If Player p has health 2000, then insert() will produce the following linked list: p -> null
- If Player p2 has health 1000, then insert() will produce the following linked list: p2->p->null
- If Player p3 has health 500, then insert() will produce the following linked list:
P3->p2->p->null
- If Player p4 has health 5000, then insert() will produce the following linked list: p3->p2->p->p4-> null
- NOTE 2: YOU SHOULD ALWAYS MAINTAIN ENCAPSULATION! Do NOT insert the Player argument, insert a copy/clone of the Player.
- Create a Driver program to test your application.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Queue example – make this generic, instead of a Queue of Customer. public class Queue { private ArrayList<Customer> line; public Queue() { line = null; } public void enqueue (Customer c) { Customer newc = new Customer (c); // copy constructor plateful.add(newc); } public Customer dequeue (Customer c) { Customer newc = line.get(0); plateful.remove(0); return newc; } } // end of class definitionarrow_forwardJava. Refer to screenshot. There is starter code for this question. public class LinkedList {Node head;Node tail; protected class Node{String data;Node next;} public String removeFirst(){//Complete the implementation of removeFirst} public String toString(){ String result = ""; Node n = head;while(n != null){result += n.data + " -> ";n = n.next;} return result;} }arrow_forwardDefine a class StatePair with two generic types (Type1 and Type2), a constructor, mutators, accessors, and a printInfo() method. Three ArrayLists have been pre-filled with StatePair data in main(): ArrayList<StatePair<Integer, String>> zipCodeState: Contains ZIP code/state abbreviation pairs ArrayList<StatePair<String, String>> abbrevState: Contains state abbreviation/state name pairs ArrayList<StatePair<String, Integer>> statePopulation: Contains state name/population pairs After a ZIP code is read into the program, complete main() to retrieve the correct state abbreviation from the ArrayList zipCodeState. Then use the state abbreviation to retrieve the state name from the ArrayList abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the ArrayList statePopulation and output the pair. Ex: If the input is: 21044the output is: Maryland: 6079602 StatePopulations.javaimport java.util.Scanner;import…arrow_forward
- Implement a Single linked list to store a set of Integer numbers (no duplicate), Using Java Language.• Instance variable• Constructor• Accessor and Update methods 1. Define a Node Class.a. Instance Variables # E element- (generics framework) # Node Next (pointer) - refer to the next node (Self-referential)b. Constructorc. Methods # E getElement() //Return the value of this node. # setElement(E e) // Set value to this node. # Node getNext() //Return the pointer of this node. # setNext(Node n) //Set pointer to this node. # displayNode() //Display information of this node.arrow_forwardAssume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck. We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck. For example, assume a deck contains cards in the following order AC 2C 3C 4C 5C AD 2D 3D 4D 5D After calling tripleCut() on the deck the card, with input a reference to 3C and a…arrow_forwardConsider the following class hierarchy: class Animal { ... } class Mammal extends Animal { ... } class Cat extends Mammal { ... } Write a generic method printArray that takes an array of any type and prints each element of the array. If the element is an instance of Animal or its subclass, the method should call the toString method of the object to print its details. Otherwise, the method should simply print the element. Your method should have the following signature: public static <T> void printArray(T[] array)arrow_forward
- The Java Programming class has two types – remote and in-person. For remote students, the weighted score comprises of midterm, final, assignments and discussion. The weights for each are 30%, 30%, 30% and 10% respectively. For in-person students, the weighted score comprises of midterm, final and assignments. The weights for each are 30%, 30% and 40%. Write a generic class Students.java which has a constructor that takes three parameters – id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString() method in this class will display these details for any student. A generic method score() will be part of this class and it will be implemented by inherited classes. Write accessors and mutators for all data points. Write two classes RemoteStudents.java and InPersonStudents.java that inherits from Student class. Show the use of constructor from parent class (mind you, RemoteStudents have one additional parameter – discussion). Implement the abstract method…arrow_forwardImplement a JAVA application that keeps track of package deliveries to consumers. Theapplication consists of the following classes:• Item: Create a class called Item. The class has two member variables oftype String named name and description and provides getter and settermethods for both.• Mail (abstract class): Implement an abstract class called Mail . The class hasa member variable of type String named address and a getter method for it.• Letter: Implement a class called Letter that inherits from Mail . The classhas one member variable of type String named contents.• Package: Implement a class called Package that inherits from Mail . Theclass has one member variable of type Item[] named items and a gettermethod for it.• DeliveryTruck: Implement a class called DeliveryTruck with a singlemember variable of type Mail[] of length 10. Add the following method:o void load(Mail mail): This method stores the given mail argumentat the next available spot in the member variable.arrow_forwardImplement a generic priority queue in Java and test it with an abstract Cryptid class and two concrete Cryptid classes, Yeti and Bigfoot. Create a code package called pq and put all the code for this assignment in it. Write the abstract class Cryptid. A Cryptid has a name (a String) and a public abstract void attack() method. Name should be protected. The class implements Comparable<Cryptid>. The compareTo method returns a negative int if the instance Cryptid's name (that is, the name of the Cryptid you called the method on) is earlier in lexicographic order than the name of the other Cryptid (the one passed in as an argument; it returns a positive int if the other Cryptid's name is earlier in lex order, and returns 0 if they are equal (that is, if they have all the same characters.) This part is easy; just have the method call String's compareTo() method on the instance Cryptid's name, with the other Cryptid's name as the argument, and return the result. Extend Cryptid…arrow_forward
- Tree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forward!!! JAVA ONLY!! Create a class Tree to model a binary tree. This class should contain a single Node which is theroot node of the tree, and should have appropriate getters/setters and toString().Again, test out your class by creating a tree and nodes objects; and by calling various methodson the them in the main method.Create the following tree using instances of your classes: HoareJonesFitzgerald AstarteRoscoeBroadfoot CreeseThis tree captures PhD supervision hierarchy: Jones was supervised by Hoare, etc.Write a method traceAndPrint for your Tree class. It should take as a parameter a stringof L and R characters, and, starting at the root, trace a path through the tree, taking a leftchild when encountering L and a right child when encountering R. Each node passed, startingwith the root, should be printed to the console. If directions given are wrong, i.e. a null isencountered, or any other character is passed to the method, an exception should be thrown.The exception should indicate…arrow_forwardJava Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
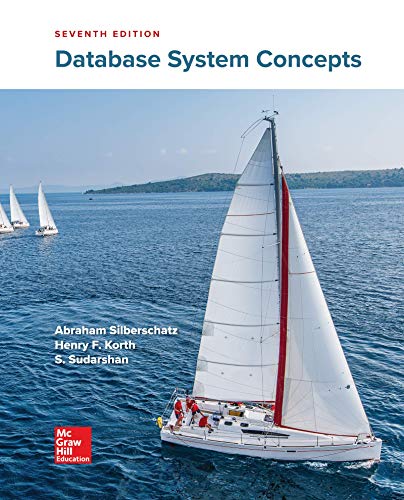
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
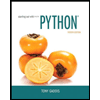
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
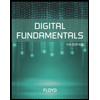
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
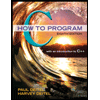
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
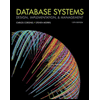
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
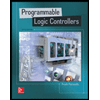
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education