Write a code in python, You will implement Hashtable using two techniques: Separate Chaining and Linear Probing. Implement Hashtable using Separate Chaining Implement hashtable using Linear Probing Test your both Hashtable classes with instances of Student class; you can have three (3) data members and appropriate functions, including hash() function. Test your both Hashtable classes with instances of Employee class; you can have three (3) data members and appropriate functions, including hash() function.
Write a code in python, You will implement Hashtable using two techniques: Separate Chaining and Linear Probing. Implement Hashtable using Separate Chaining Implement hashtable using Linear Probing Test your both Hashtable classes with instances of Student class; you can have three (3) data members and appropriate functions, including hash() function. Test your both Hashtable classes with instances of Employee class; you can have three (3) data members and appropriate functions, including hash() function.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Write a code in python, You will implement Hashtable using two techniques: Separate Chaining and Linear Probing.
- Implement Hashtable using Separate Chaining
- Implement hashtable using Linear Probing
- Test your both Hashtable classes with instances of Student class; you can have three (3) data members and appropriate functions, including hash() function.
- Test your both Hashtable classes with instances of Employee class; you can have three (3) data members and appropriate functions, including hash() function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
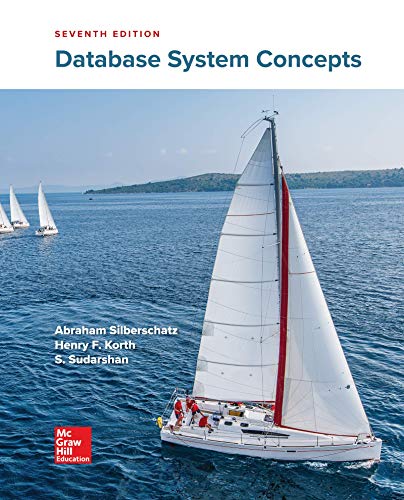
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
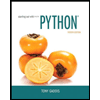
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
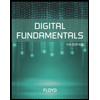
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
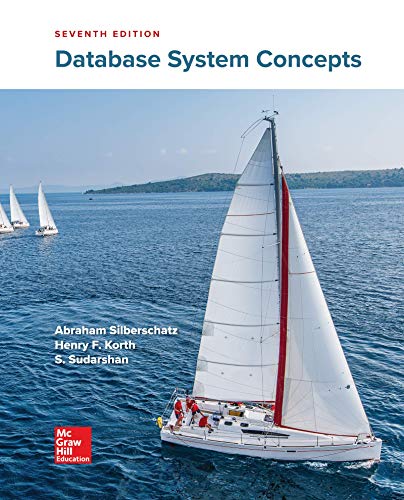
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
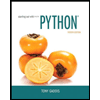
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
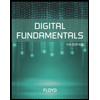
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
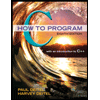
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
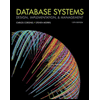
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
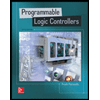
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education