a. Define a Deque interface.
b. Define a LinkedDeque class (Node class)..
c. Define a Test class to test all methods
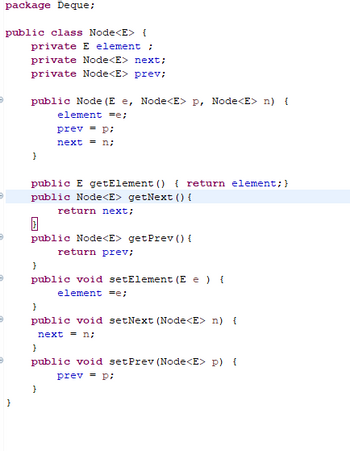
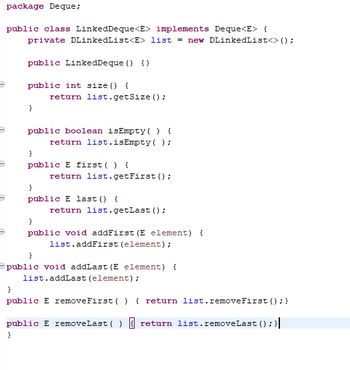

The code you provided looks like a complete and correct implementation of a Deque using a doubly linked list in Java. Here's the breakdown of the provided code:
The
Deque
interface defines the methods for a double-ended queue.The
Node
class represents nodes in the doubly linked list. Each node has an element, a reference to the previous node (prev
), and a reference to the next node (next
).The
LinkedDeque
class implements theDeque
interface using a doubly linked list. It has ahead
, atail
, and asize
to maintain the state of the deque. The methods are implemented as follows:addFirst
andaddLast
add elements to the front and back of the deque, respectively.removeFirst
andremoveLast
remove elements from the front and back of the deque, respectively.isEmpty
checks if the deque is empty.first
andlast
return the elements at the front and back of the deque, respectively.
The
Main
class is used to test the functionality of theLinkedDeque
class. It creates aLinkedDeque
of integers, adds elements, and removes elements to demonstrate how the deque works.
Step by stepSolved in 5 steps with 2 images

- For the Queue use the java interface Queue with the ArrayDeque classFor the Stack use the java Deque interface with the ArrayDeque classFor the LinkedList, use the java LinkedList class Also, when you are asked to create and use a Queue, you can use only those methods pertaining to the general operations of a queue (Enqueue: addLast, Dequeue: removeFirst, and peek() Similarly, when you are asked to create and/or use a stack, you can use only those methods pertaining to the general operations of a Stack (push: addFirst, pop:removeFirst, and peek() ) Your code should not only do the job, but also it should be done as efficiently as possible: fast and uses no additional memory if at all possible Questions Write a method “int GetSecondMax(int[] array)” . this method takes an array of integers and returns the second max value Example: if the array contains: 7, 2, 9, 5, 4, 15, 6, 1}. It should return the value: 9arrow_forwardVERY EASY RPN PROBLEM. read the directions please answer thank you public class Node <T>{ private T data; private Node<T> next; public Node(T data, Node<T> next) { this.data = data; this.next = next; } public T getData() { return data; } public Node<T> getNext() { return next; } } import java.util.Stack; public class StackArray<T> implements Stack<T> { private T [] elements; private int top; private int size; public StackArray (int size) { elements = (T[]) new Object[size]; top = -1; this.size = size; } public boolean empty() { return top == -1; } public boolean full() { return top == size - 1; } public boolean push(T el) { if (full()) return false; else { top++; elements[top] = el; return true; } }…arrow_forward/** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forward
- Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forwardCreate a class Stack. This stack will be implemented using the LinkedList class that has been provided. This stack will hold values of a generic type (<T>). Your Stack should have the following public methods: public void push(int n) public T pop() public T peek() public T size() public boolean isEmpty() public class LinkedList <T> { private Node head; private Node tail; private int size; public LinkedList() { head = null; tail = null; size = 0; } public void append(T data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { tail.next = newNode; } tail = newNode; size++; } public void prepend(T data) { Node newNode = new Node(data); if (head == null) { head = newNode; tail = newNode; } else { newNode.next = head; head = newNode; } size++; } public T getHead() { return head.data; } public T getTail() { return tail.data; } public int size() { return size; } public void removeByValue(T data) { Node current = head; while…arrow_forward
- 1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forwardUse a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods please help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardGiven the tollowiıng class template detinition: template class linkedListType { public: const linked ListType& operator=(const linked ListType&); I/Overload the assignment operator. void initializeList(); /Initialize the list to an empty state. //Postcondition: first = nullptr, last = nullptr, count = 0; linked ListType(); Ildefault constructor //Initializes the list to an empty state. /Postcondition: first = nullptr, last = nullptr, count = 0; -linkedListīype(); Ildestructor /Deletes all the nodes from the list. //Postcondition: The list object is destroyed. protected: int count; //variable to store the number of elements in the list nodeType *first; //pointer to the first node of the list nodeType *last; //pointer to the last node of the list 1) Write initializeList() 2) Write linkedListType() 3) Write operator=0 4) Add the following function along with its definition: void rotate(); I/ Remove the first node of a linked list and put it at the end of the linked listarrow_forward
- Implement the Linked List using head and tail pointer. Interface (.h file) of LinkedList class is given below. Your task is to provide implementation (.cpp file) for LinkedList class. Interface: template<class Type> class LinkedList { private: Node<Type>* head; Node<Type>* tail; <Type> data; public: LinkedList<Type>(); LinkedList<Type>(const LinkedList<Type> &obj); void sortedInsert(Type); Type deleteFromPosition(int position); //deletes the node at the particular position Type delete(int start,int end); //deletes the nodes from starting to ending position bool isEmpty()const; void print()const; ~LinkedList(); }; program in c++.arrow_forwardUse a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forward