Given the interface (header file) of a parametroc Deque below, write its implementation: Listing 3: A Parameterized Deque Class template class Deque { private : /∗∗ ∗ Forward Declaration of the parametric nested ∗ class representing node objects of the deque ∗/ template class Node; /∗∗ ∗ A pointer to the head node of this deque ∗/ Node∗ head; /∗∗ ∗ A pointer to the rear node of this deque ∗/ Node∗ rear; /∗∗ ∗ The number of nodes that this deque has ∗/ int length ; public : /∗∗ ∗ Constructs an empty Deque ; ∗/ Deque() ; /∗∗ ∗ Copy constructor . ∗/ Deque(const Deque& aDeque); /∗∗ ∗ destructor − returns the deque’s memory to the system←֓ ; ∗/ ̃Deque() ; /∗∗ ∗ Determine whether the deque is empty. ∗ @return true if the the deque is empty; ∗ otherwise, it returns false . ∗/ bool isEmpty () const ; /∗∗ ∗ Inserts an item at the front of the deque. ∗ @param item the value to be inserted . ∗/ void pushFront(T item ) ; /∗∗ ∗ Inserts an item at the back of the deque. ∗ @param item the value to be inserted . ∗/ void pushBack(T item); /∗∗ ∗ Returns the item at the front of a non−empty deque. ∗ @return item at the front of ∗ @throw a DequeException when this deque is empty ∗/ const T& front () const ; /∗∗ ∗ Returns the item at the back ∗ @return item at the back of the queue. ∗ @throw a DequeException when t h i s deque i s empty ∗/ const T& back() const; /∗∗ ∗ Deletes an item from the front of the dequeue. ∗ @return the item at the front of the queue. ∗ @throw a DequeException when this deque is empty. ∗/ T popFront(); /∗∗ ∗ Deletes an item from the back of the dequeue. ∗ @return the item at the back of the queue. ∗ @throw a DequeException when this deque is empty. ∗/ T popBack(); /∗∗ ∗ Allows access to the element at the specified index ∗ @param index the subscript of the element. ∗ @return a reference to an element. ∗ @throw a DequeException when index < 0 ∗ or index > length−1 ∗/ T& operator[](int index); /∗∗ ∗ @return the size of the deque ∗/ int size() const; }; /∗∗ ∗ Definition of the nested Node class ∗/ template template class Deque::Node { private : /∗∗ ∗ The data item in this node ∗/ T data; /∗∗ ∗ The pointer to the previous node ∗/ Node∗ prev; /∗∗ ∗ The pointer to the next node ∗/ Node∗ next; /∗∗ ∗ Makes private members of this class accessible ∗ in the Deque class ∗/ friend class Deque; public : /∗∗ ∗ Constructs a node with a given data value. ∗ @param s the data to store in this node ∗/ Node(T s); };
Given the interface (header file) of a parametroc Deque<T> below, write its implementation:
Listing 3: A Parameterized Deque Class
template <typename T>
class Deque
{
private :
/∗∗
∗ Forward Declaration of the parametric nested
∗ class representing node objects of the deque
∗/
template <typename U> class Node;
/∗∗
∗ A pointer to the head node of this deque
∗/
Node<T>∗ head;
/∗∗
∗ A pointer to the rear node of this deque
∗/
Node<T>∗ rear;
/∗∗
∗ The number of nodes that this deque has
∗/
int length ;
public :
/∗∗
∗ Constructs an empty Deque ;
∗/
Deque() ;
/∗∗
∗ Copy constructor .
∗/
Deque(const Deque<T>& aDeque);
/∗∗
∗ destructor − returns the deque’s memory to the system←֓
;
∗/
̃Deque() ;
/∗∗
∗ Determine whether the deque is empty.
∗ @return true if the the deque is empty;
∗ otherwise, it returns false .
∗/
bool isEmpty () const ;
/∗∗
∗ Inserts an item at the front of the deque.
∗ @param item the value to be inserted .
∗/
void pushFront(T item ) ;
/∗∗
∗ Inserts an item at the back of the deque.
∗ @param item the value to be inserted .
∗/
void pushBack(T item);
/∗∗
∗ Returns the item at the front of a non−empty deque.
∗ @return item at the front of
∗ @throw a DequeException when this deque is empty
∗/
const T& front () const ;
/∗∗
∗ Returns the item at the back
∗ @return item at the back of the queue.
∗ @throw a DequeException when t h i s deque i s empty
∗/
const T& back() const;
/∗∗
∗ Deletes an item from the front of the dequeue.
∗ @return the item at the front of the queue.
∗ @throw a DequeException when this deque is empty.
∗/
T popFront();
/∗∗
∗ Deletes an item from the back of the dequeue.
∗ @return the item at the back of the queue.
∗ @throw a DequeException when this deque is empty.
∗/
T popBack();
/∗∗
∗ Allows access to the element at the specified index
∗ @param index the subscript of the element.
∗ @return a reference to an element.
∗ @throw a DequeException when index < 0
∗ or index > length−1
∗/
T& operator[](int index);
/∗∗
∗ @return the size of the deque
∗/
int size() const;
};
/∗∗
∗ Definition of the nested Node class
∗/
template <typename U>
template <typename T>
class Deque<U>::Node
{
private :
/∗∗
∗ The data item in this node
∗/
T data;
/∗∗
∗ The pointer to the previous node
∗/
Node<T>∗ prev;
/∗∗
∗ The pointer to the next node
∗/
Node<T>∗ next;
/∗∗
∗ Makes private members of this class accessible
∗ in the Deque<T> class
∗/
friend class Deque<U>;
public :
/∗∗
∗ Constructs a node with a given data value.
∗ @param s the data to store in this node
∗/
Node(T s);
};

Step by step
Solved in 3 steps

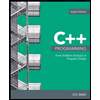
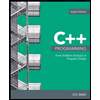