complete TODO's using java: -Main- package edu.luc.cs271.wordcount; import java.util.*; public class Main { public static void main(final String[] args) throws InterruptedException { // set up the scanner so that it separates words based on space and punctuation final Scanner input = new Scanner(System.in).useDelimiter("[^\\p{Alnum}]+"); // TODO complete this main program // 0. create suitable map instance // 1. create a WordCounter instance // 2. use this to count the words in the input // 3. determine the size of the resulting map // 4. create an ArrayList of that size and // 5. store the map's entries in it (these are of type Map.Entry) // 6. sort the ArrayList in descending order by count // using Collections.sort and an instance of the provided comparator (after fixing the latter) // 7. print the (up to) ten most frequent words in the text } } -DescendingByCount- package edu.luc.cs271.wordcount; import java.util.Comparator; import java.util.Map; /** The comparison strategy for sorting the array by the word count. */ public class DescendingByCount implements Comparator> { public int compare(final Map.Entry l, final Map.Entry r) { // TODO turn this into descendi
complete TODO's using java:
-Main-
package edu.luc.cs271.wordcount;
import java.util.*;
public class Main {
public static void main(final String[] args) throws InterruptedException {
// set up the scanner so that it separates words based on space and punctuation
final Scanner input = new Scanner(System.in).useDelimiter("[^\\p{Alnum}]+");
// TODO complete this main program
// 0. create suitable map instance
// 1. create a WordCounter instance
// 2. use this to count the words in the input
// 3. determine the size of the resulting map
// 4. create an ArrayList of that size and
// 5. store the map's entries in it (these are of type Map.Entry<String, Integer>)
// 6. sort the ArrayList in descending order by count
// using Collections.sort and an instance of the provided comparator (after fixing the latter)
// 7. print the (up to) ten most frequent words in the text
}
}
-DescendingByCount-
package edu.luc.cs271.wordcount;
import java.util.Comparator;
import java.util.Map;
/** The comparison strategy for sorting the array by the word count. */
public class DescendingByCount implements Comparator<Map.Entry<String, Integer>> {
public int compare(final Map.Entry<String, Integer> l, final Map.Entry<String, Integer> r) {
// TODO turn this into descending order using the getValue() method on l and r
return -1;
}
}
-WordCounter-

Step by step
Solved in 3 steps with 2 images

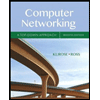
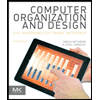
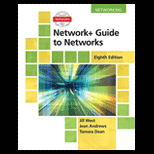
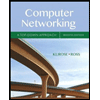
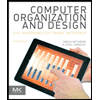
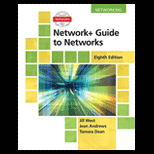
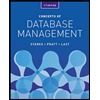
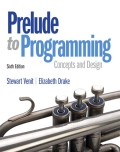
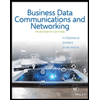