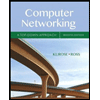
Can someone help me with this?
code:
public class ArrayListFun {
/**
* Counts the number of instances of a particular value in list.
* Returns null if list is null.
*
* @param list
* @param checkNum
* @return the number of Integer occurrences in list equal to checkNum
*/
public static Integer countOccurrences(ArrayList<Integer> list, int checkNum) {
// TODO: FILL IN BODY
return null;
}
/**
* Calculates the sum, product, or mean of all values in list.
* Returns null if list is null.
*
* @param list
* @param operation
* @return the sum, product, or mean of all values in list
*/
public static Integer mathOperation(ArrayList<Integer> list, String operation) {
// TODO: FILL IN BODY
return null;
}
/**
* Converts the 1s and 0s in list (binary value) to its decimal value
*
* Example: 100110 from binary to decimal
*
* 1 * 2^5 +
* 0 * 2^4 +
* 0 * 2^3 +
* 1 * 2^2 +
* 1 * 2^1 +
* 0 * 2^0 =
* 38
*
* For more information on binary, see zyBooks chapter 3.1
*
* Returns null if list is null.
*
* @param list
* @return the decimal value of the binary representation of list
*/
public static Integer binaryToDecimal(ArrayList<Integer> list) {
// TODO: FILL IN BODY
return null;
}
/**
* Returns true if list is a (character) palindrome.
* Returns null if list is null.
*
* @param list
* @return true if the list is a palindrome, else false.
*/
public static Boolean isPalindrome(ArrayList<Character> list) {
// TODO: FILL IN BODY
return null;
}
public static void main(String[] args) {
// TODO: FILL IN BODY
}
}
![Example method arguments:
[2, 0, 5, 2, 2, 1, 1] and operation
// list
// list
"add"
[2, 0, 5, 2, 2, 1, 1] and operation
"multiply"
// list
[2, 0, 5, 2, 2, 1, 1] and operation
"mean"
Example method return values:
13
1
3. (binaryToDecimal) Covert an ArrayList containing only Os and 1s to its corresponding decimal value (index 0 of the ArrayList being the
leftmost bit)
Iterate through each value in list. Add (list value)*(2^j) to a running sum variable. Here 'j' is the index opposite of conventional Java
indexing (ie 0 index is the furthest right index, and length-1 is the furthest left index). Further information on converting from binary to
decimal can be found in zyBooks Table 2.1.2.
• Return the running sum value. However, if list is null, return null.
Example method arguments:
// list
[1, 0, 0, 1,
[1, 0, 0, 1, 1, 1]
1]
// list
Example method return values:
19
39
4. (isPalindrome) Implement a palindrome checker Note: a palindrome is a word or phrase that is spelled the same forwards as it is](https://content.bartleby.com/qna-images/question/a72dae9f-0052-41b5-80f3-bd0e25abf3cd/0bead6b3-aa11-43d2-8d99-d33de4dc1763/rpodwc_thumbnail.png)
![9.11 ***zyLab: ArrayList Fun
The goal of this program is to practice performing some common programming operations using only 1-dimensional ArrayLists.
You will write four methods, each of which perform some operation on an ArrayList. Each method is described below. In main, you will call
your four methods using the provided arguments.
1. (countOccurrences) Count the number of instances of a particular value in an ArrayList
• Iterate through each value in list, incrementing a running sum variable by one for every instance equal to checkNum in list.
• Return the running sum. However, if list is null, return null.
Example method arguments:
[2, 0, 5, 2, 2, 1, 1] and checkNum
[2, 0, 5, 2, 2, 1, 1] and checkNum
// list
2
%3D
// list
3
||
Example method return value:
3
2. (mathOperation) Perform one of three math operations (add, multiply, or mean) on an ArrayList
• Iterate through each value in list.
If operation equals "add", then every iteration of list will add the value at that particular index in list to a running sum variable.
• If operation equals "multiply", then every iteration of list will multiply the value at that particular index in list to a running product
variable.
If operation equals "mean", then every iteration of list will add the value at that particular index in list to a running sum variable. After
iterating through the list, divide that running sum by the number of elements in list. Note that because this method returns an Integer,
the mean may be truncated.](https://content.bartleby.com/qna-images/question/a72dae9f-0052-41b5-80f3-bd0e25abf3cd/0bead6b3-aa11-43d2-8d99-d33de4dc1763/mruv6q7_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Question: Find the second largest number Code below: public static int findSecondLargest(List<Integer> intList) { // code here }arrow_forward//No need for the whole code //just the methods please for both Write a recursive method “int sumPos(Node head)” to calculate the sum of positive integers in a linked list of integers referenced by head. No global variables are allowed. Node is declared as: Node { int value; Node next; } Write a non-recursive method “int sumPos(Node head)” to calculate the sum of positive integers in a linked list of integers referenced by head. No global variables are allowed. Node is declared as: Node { int value; Node next; }arrow_forwardThe following code prints out all permutations of the string generate. Insert the missing statement. public class PermutationGeneratorTester { public static void main(String[] args) { PermutationGenerator generator = new PermutationGenerator("generate"); ArrayList<String> permutations = generator.getPermutations(); for (String s : permutations) { ____________________ } } }arrow_forward
- helparrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardLab 14.1 Beginning to build an Arrazlizt recursively In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a sequence of problems without using while or for loops. Instead we will think recursively and look at the world through a different lens. Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing about recursion is that you can assume you already know how to solve the smaller problem. The goal is to demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are: 00 01 10 11 How can we solve the larger problem with a list of strings of length 2? Add a "0" or "1", right? So here is the solution to the larger problem: 00 + 0 = 000 01 + 0 = 010 10 + 0 = 100 11 +0 = 110 and 00 + 1 = 001 01 + 1 = 011 10 + 1 =…arrow_forward
- PROBLEM STATEMENT: In this problem you will need to insert 5 at the frontof the provided ArrayList and return the list. import java.util.ArrayList;public class InsertElementArrayList{public static ArrayList<Integer> solution(ArrayList<Integer> list){// ↓↓↓↓ your code goes here ↓↓↓↓return new ArrayList<>(); Can you help me with this question The Language is Javaarrow_forwardThe ArrayList is created using the following syntax: String[] array = {"red", "green", "blue"}; ArrayList list Display all the elements of this list using: a) foreach loop b) foreach method with lambda := new ArrayList(java.util.Arrays.asList(array));arrow_forwardJava - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forward
- PYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardAre they equivalent? 1) ArrayList<?> list = new ArrayList(); 2) ArrayList<? extends Object> list = new ArrayList<>();arrow_forwardCan you please answer this fast and with correct answer. Thankyouarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
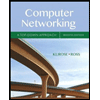
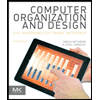
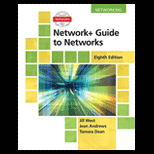
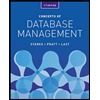
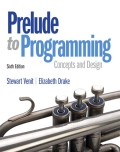
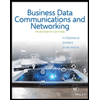