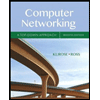
Implement the function below.
void swap(int pos1, int pos2){}
Initial code to be completed:
class ArrayList : public List {
int* array;
int index;
int capacity;
void dyn_all_add(){
int cap = ceil(capacity * 1.5);
array = (int*)realloc(array,cap * sizeof(int));
capacity = cap;
}
void dyn_all_rem(){
int cap = capacity - (capacity/3);
array = (int*)realloc(array,cap * sizeof(int));
capacity = cap;
}
public:
// CONSTRUCTOR
ArrayList() {
capacity = 4;
array = (int*)malloc(capacity);
index = 0;
}
int add(int num) {
if (index == capacity){
dyn_all_add();
}
*(array + index) = num;
index++;
return index;
}
int get(int pos){
if (pos-1 < index){
return *(array + pos-1);
}
return -1;
}
int size(){
return index;
}
void swap(int pos1, int pos2){
}
// WARNING! Do not modify the print method.
// Doing so will nullify your score for this activity.
void print() {
cout << "[";
for (int i = 0; i < capacity; i++) {
if (i < index) {
cout << *(array + i);
} else {
cout << "?";
}
if (i != capacity - 1) {
cout << ", ";
}
}
cout << "]" << endl;
}
};

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- JAVA programming languagearrow_forwardStudent* func () { unique ptr arr[] make_unique ("CSC340") }; // #1 Insert Code int main () ( // #2 Insert Code [ #1 Insert Code]: Write code to keep all the object(s) which element(s) of array arr owns alive outside of the scope of func. [#2 Insert Code]: Write code to have a weak_ptr monitor the object which survived; Then test if it has any owner; Then properly destroy it; Then test again if has any owner; Then destroy the Control Block.arrow_forwardData Structure & Algorithm: Show by fully java coded examples how any two sorting algorithms work. You should test these with various array sizes and run them several times and record the execution times. At least four array sizes are recommended. 15, 20, 25, 30. It is recommended you write the classes and then demonstrate/test them using another class. Discuss the comparative efficiencies of your two chosen sorting algorithms.arrow_forward
- f18 In c++arrow_forwardJava - Gift Exchange *** Please include UML Diagram and notes in code Minimum requirements are: At least 1 loop An Array or ArrayList At least 3 Java classes Use methods I am trying to make it so that the program will: Prompt for the number of people included in exchange - If not even it will state that there has to be an even number and ask for the number of people included again (loops). Prompt to enter a participant's first name Prompt for the participant's age Print out the random matching of participants so that everyone gets a gift and everyone gives a gift. Please include UML Diagramarrow_forward#Steps: Create a Main class. Declare an ArrayList in the main() method and store the given values in it. Create an array called output of the size of the ArrayList. Iterate through for-loop to get each element from the array List and load it into the array using the get() method of the list. Print the array to see the loaded elements. import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { List<String> nameList = new ArrayList<String>(); nameList.add("Robert"); nameList.add("Samson"); nameList.add("Alex"); nameList.add("William"); System.out.println("Elements of List: " + nameList); // Create an array of the same size as nameList Hint: use size() method // Fetch the elements from the nameList and insert into newly created array // Hint: Use get() method to fetch the elements from…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
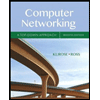
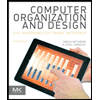
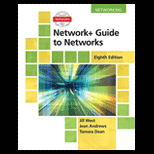
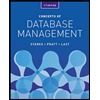
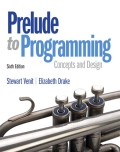
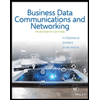