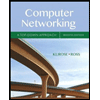
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
COMPLETE THIS CODE and make sure it passes all the test cases.
// EXERCISE 4.1 MYLIST ITERATIVE CATENATE MUTATE
/**
* Catenate two MyLists, listA and listB. Mutate listA.
* @param listA is a MyList object.
* @param listB is a MyList object.
* @return a list consisting of the elements of listA followed by the
* elements of listB.
*/
public static MyList iterCatMutList(MyList listA, MyList listB) {
}
![Complete the method MyList iterCatMutlist (MyList listA, MyList 1istB) iteratively, to return a list
consisting of all elements of listA, followed by all elements of listB.
The method modifies/mutates listA so that it is concatenated with listB.
Use loops.
Test case 1:
[1, 2, 3],
MyList.iterCatMutlist (list1, list2);
list1
list2
[4, 5, 6]
list
list
[1, 2, 3, 4, 5, 6]
list1 → [1, 2, 3, 4, 5, 6]
For example:
Test
Result
My List list1 = MyList.ofEntries (1, 2, 3);
[1, 2, 3, 4, 5, б]
MyList list2 = MyList.ofEntries(4, 5, 6);
[1, 2, 3, 4, 5, 6]
MyList list = MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
MyList list1
MyList.ofEntries();
null
My List list2 = MyList.ofEntries ();
null
MyList list
MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
MyList list1
MyList.ofEntries(5);
[5]
My List list2
MyList.ofEntries();
[5]
%3D
MyList list
MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
My List list1
My List list2
MyList.ofEntries();
[5]
%3D
MyList.ofEntries(5);
null
MyList list = MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);](https://content.bartleby.com/qna-images/question/7933b4d7-dfbc-452b-bf78-981e959f7b6a/1498aea5-d85a-47b8-acd8-3f6a2d4ae416/2os07kg_thumbnail.png)
Transcribed Image Text:Complete the method MyList iterCatMutlist (MyList listA, MyList 1istB) iteratively, to return a list
consisting of all elements of listA, followed by all elements of listB.
The method modifies/mutates listA so that it is concatenated with listB.
Use loops.
Test case 1:
[1, 2, 3],
MyList.iterCatMutlist (list1, list2);
list1
list2
[4, 5, 6]
list
list
[1, 2, 3, 4, 5, 6]
list1 → [1, 2, 3, 4, 5, 6]
For example:
Test
Result
My List list1 = MyList.ofEntries (1, 2, 3);
[1, 2, 3, 4, 5, б]
MyList list2 = MyList.ofEntries(4, 5, 6);
[1, 2, 3, 4, 5, 6]
MyList list = MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
MyList list1
MyList.ofEntries();
null
My List list2 = MyList.ofEntries ();
null
MyList list
MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
MyList list1
MyList.ofEntries(5);
[5]
My List list2
MyList.ofEntries();
[5]
%3D
MyList list
MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
My List list1
My List list2
MyList.ofEntries();
[5]
%3D
MyList.ofEntries(5);
null
MyList list = MyList.iterCatMutlist(list1, list2);
System.out.println(list);
System.out.println(list1);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- starter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardSet and Map class templates are similar in the sense that they both use binary search trees. The difference is Set is used to store only keys while Map is used to store (key, value) pairs. Group of answer choices True Falsearrow_forwardLab 14.1 Beginning to build an Arrazlizt recursively In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a sequence of problems without using while or for loops. Instead we will think recursively and look at the world through a different lens. Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing about recursion is that you can assume you already know how to solve the smaller problem. The goal is to demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are: 00 01 10 11 How can we solve the larger problem with a list of strings of length 2? Add a "0" or "1", right? So here is the solution to the larger problem: 00 + 0 = 000 01 + 0 = 010 10 + 0 = 100 11 +0 = 110 and 00 + 1 = 001 01 + 1 = 011 10 + 1 =…arrow_forward
- Pythong help!arrow_forwardA-1: Let l = [−1, −2, . . . , −10] be an existing list. Construct a new list from l by dividing each evennumber element in l by 2. Print the new list. Do not use NUMPY library.A-2: Create a new list containing the following elements using list comprehension:[1, −1, 2, −2, 3, −3, ..., 9, −9]A-3: Consider the following coded string. Create a list of all contiguous (connected without space) lettersin the order of their appearance."Vjg dguv rtqitcou ctg ytkvvgp uq vjcv eqorwvkpi ocejkpgu ecp rgthqto vjgoswkemn{0 Cnuq. vjg dguv rtqitcou ctg ytkvvgp uq vjcv jwocp dgkpiu ecp wpfgtuvcpfvjgo engctn{0 C iqqf guuc{kuv cpf c iqqf rtqitcoogt jcxg c nqv kp eqooqp0"A-4: In the list obtained after executing task(s) from Part(A-3), remove newline characters (if any).A-5: For the list obtained after executing task(s) from Part(A-4), for each word in the list do thefollowing: Breakdown the word into list of letters. Convert each of the above letters into integer, using ord() function. Update the…arrow_forwardInstructions Write a program to test various operations of the class doublyLinkedList. Your program should accept a list of integers from a user and use the doubleLinkedList class to output the following: The list in ascending order. The list in descending order. The list after deleting a number. A message indicating if a number is contained in the list. Output of the list after using the copy constructor. Output of the list after using the assignment operator. An example of the program is shown below: Enter a list of positive integers ending with -999: 83 121 98 23 57 33 -999 List in ascending order: 23 33 57 83 98 121 List in descending order: 121 98 83 57 33 23 Enter item to be deleted: 57 List after deleting 57 : 23 33 83 98 121 Enter item to be searched: 23 23 found in the list. ********Testing copy constructor*********** intList: 23 33 83 98 121 ********Testing assignment operator*********** temp: 23 33 83 98 121 Your program should use the value -999 to denote the end of the…arrow_forward
- PYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardExplain the differences between a statically allocated array, a dynamically allocated array, and a linked list.arrow_forwardThis is using Data Structures in Javaarrow_forward
- Java - Elements in a Rangearrow_forwardCan you please answer this fast and with correct answer. Thankyouarrow_forwardGiven an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list(this is not graded).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
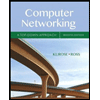
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
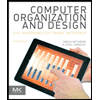
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
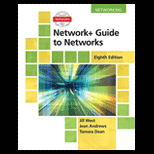
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
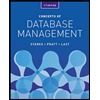
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
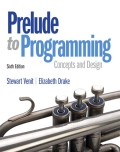
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
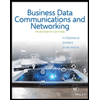
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY