A list of lists of numbers is a list such that all its elements are lists of numbers. Consider the following examples: • [[3,5], [], [1, 1, 1]] is a list of list of numbers; • [ [ [3,5]]], [], [1]] is not a list of list of numbers due to the first element; • [2, [1]] is not a list of list of numbers due to the first element, but note that the tree that encodes this list also encodes [[0,0],[1]] which is a list of list of numbers. Note that an empty list can always be considered a list of numbers and a list of lists of numbers. Answer for EACH of the given trees (a)–(d) the following question: Does it encode a list of numbers? If it does which is the corresponding list of numbers? USE THE ENCODING OF DATATYPES IN D BELOW (a) ((nil.nil).((nil. (nil.nil)).<
A list of lists of numbers is a list such that all its elements are lists of numbers.
Consider the following examples:
• [[3,5], [], [1, 1, 1]] is a list of list of numbers;
• [ [ [3,5]]], [], [1]] is not a list of list of numbers due to the first element;
• [2, [1]] is not a list of list of numbers due to the first element, but note that the tree that encodes this list also encodes [[0,0],[1]] which is a list of list of numbers.
Note that an empty list can always be considered a list of numbers and a list of lists of numbers.
Answer for EACH of the given trees (a)–(d) the following question:
Does it encode a list of numbers? If it does which is the corresponding list of numbers?
USE THE ENCODING OF DATATYPES IN D BELOW
(a) ((nil.nil).((nil. (nil.nil)).<<nil.(nil.nil)).nil)))
(b) (nil.((nil.nil).(({nil.(nil.nil)).nil).nil)))
(c) (((nil.nil).((nil. (nil.nil)).nil)).< (nil.(nil. (nil.nil ))).nil ))
(d) ((nil.nil).((nil.nil).<<<<nil.nil).nil).nil).nil)))

Step by step
Solved in 3 steps

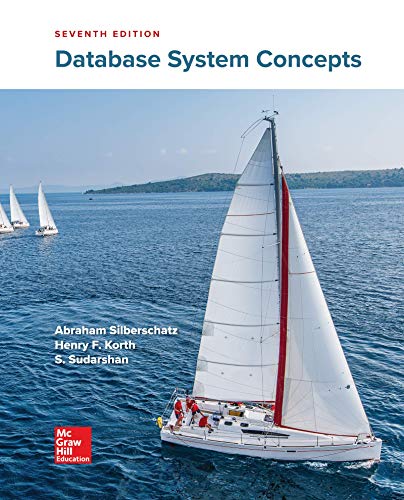
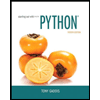
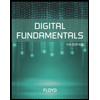
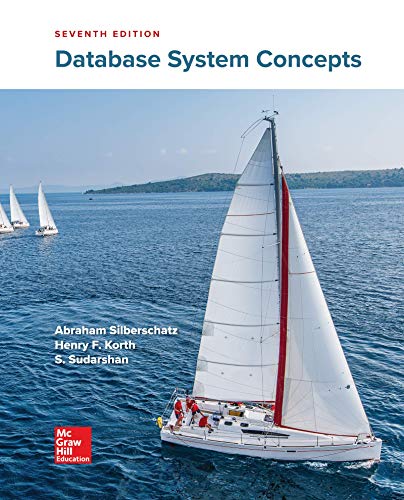
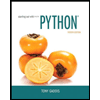
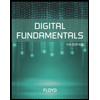
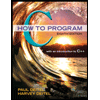
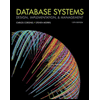
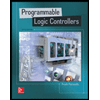