def large_matrix(matrix: list[list[int]]) -> int: """ Returns the area of the rectangle in the area of a rectangle is defined by number of 1's that it contains. The matrix will only contain the integers 1 and 0. >>> case = [[1, 0, 1, 0, 0], ... [1, 0, 1, 1, 1], ... [1, 1, 1, 1, 1], ... [1, 0, 0, 1, 0]] >>> largest_in_matrix(case1) 6"" You must use this helper code: def large_position(matrix: list[list[int]], row: int, col: int) -> int: a = row b = col max = 0 temp = 0 rows = len(matrix) column = len(matrix[a]) while a < rows and matrix[a][col] == 1: temp = 0 while b < column and matrix[a][b] == 1: temp = temp + 1 b = b + 1 column = b a = a + 1 if (a != row+1): temp2 = temp * (a - row) else: temp2 = temp if max < temp2: max = temp2 b = col return max """ remember: Please do this on python you should not use any of the following: dictionaries or dictionary methods try-except break and continue statements recursion map / filter import""'
def large_matrix(matrix: list[list[int]]) -> int:
"""
Returns the area of the rectangle in the area of a rectangle is defined by number of 1's that it contains.
The matrix will only contain the integers 1 and 0.
>>> case = [[1, 0, 1, 0, 0],
... [1, 0, 1, 1, 1],
... [1, 1, 1, 1, 1],
... [1, 0, 0, 1, 0]]
>>> largest_in_matrix(case1)
6""
You must use this helper code:
def large_position(matrix: list[list[int]], row: int, col: int) -> int:
a = row
b = col
max = 0
temp = 0
rows = len(matrix)
column = len(matrix[a])
while a < rows and matrix[a][col] == 1:
temp = 0
while b < column and matrix[a][b] == 1:
temp = temp + 1
b = b + 1
column = b
a = a + 1
if (a != row+1):
temp2 = temp * (a - row)
else:
temp2 = temp
if max < temp2:
max = temp2
b = col
return max
""" remember:
Please do this on python
you should not use any of the following:
- dictionaries or dictionary methods
- try-except
- break and continue statements
- recursion
- map / filter
- import""'

Step by step
Solved in 2 steps with 1 images

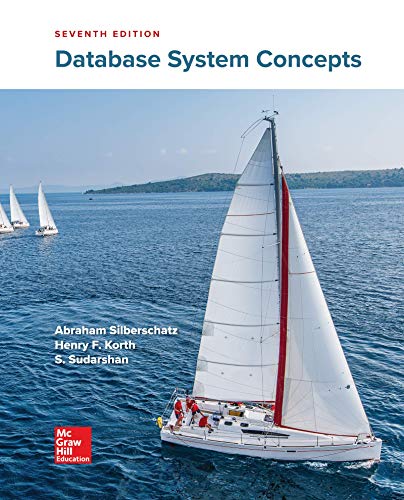
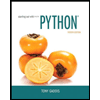
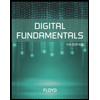
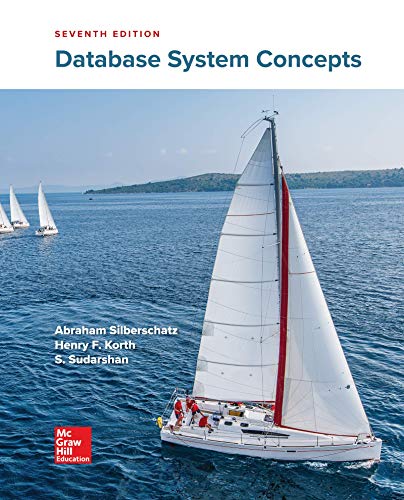
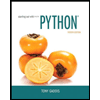
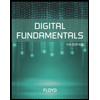
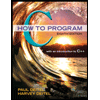
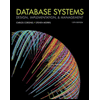
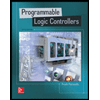