A-1: Let l = [−1, −2, . . . , −10] be an existing list. Construct a new list from l by dividing each even number element in l by 2. Print the new list. Do not use NUMPY library.
A-1: Let l = [−1, −2, . . . , −10] be an existing list. Construct a new list from l by dividing each even
number element in l by 2. Print the new list. Do not use NUMPY library.
A-2: Create a new list containing the following elements using list comprehension:
[1, −1, 2, −2, 3, −3, ..., 9, −9]
A-3: Consider the following coded string. Create a list of all contiguous (connected without space) letters
in the order of their appearance.
"Vjg dguv rtqitcou ctg ytkvvgp uq vjcv eqorwvkpi ocejkpgu ecp rgthqto vjgo
swkemn{0 Cnuq. vjg dguv rtqitcou ctg ytkvvgp uq vjcv jwocp dgkpiu ecp wpfgtuvcpf
vjgo engctn{0 C iqqf guuc{kuv cpf c iqqf rtqitcoogt jcxg c nqv kp eqooqp0"
A-4: In the list obtained after executing task(s) from Part(A-3), remove newline characters (if any).
A-5: For the list obtained after executing task(s) from Part(A-4), for each word in the list do the
following:
Breakdown the word into list of letters.
Convert each of the above letters into integer, using ord() function.
Update the integer values by -2 for all the above integers.
Convert each updated integer value into letter, using chr() function.
Join the updated letters in the order to create new word.
A-6: Join the list of new words obtained after executing task(s) from Part(A-5), into one string of words.
Print the string.
A-7: Create a python program, that does the following:
Ask the user to enter a number from the following choices: 10, 20, 30, 40, 50, 60, 70, 80, 90, 100
Returns the roman numerals and the word form for the entered number.
Hint(A-5): ‘a’ is chr(97), and ord(‘a’) is 97.
Hint(A-7): Use the following list for roman numerals correspondingly to the above numbers:
“X”, “XX”, “XXX”, “XL”, “L”, “LX”, “LXX”, “LXXX”, “XC”, “C”
☞ Note: Solve all the above questions using Python only. Do NOT use Numpy o
![Answer all the following questions:
A-1: Let l = [-1, –2, ...,–10] be an existing list. Construct a new list from 1 by dividing each even
number element in l by 2. Print the new list. Do not use NUMPY library.
A-2: Create a new list containing the following elements using list comprehension:
[1, –1, 2, –2,3, –3, ...,9, –9]
A-3: Consider the following coded string. Create a list of all contiguous (connected without space) letters
in the order of their appearance.
"Vjg dguv rtqitcou ctg ytkvvgp uq vjcv eqorwvkpi ocejkpgu ecp rgthqto vjgo
swkemn{0 Cnuq. vjg dguv rtqitcou ctg ytkvvgp uq vjcv jwocp dgkpiu ecp wpfgtuvcpf
vjgo engctn{0 C iqqf guuc{kuv cpf c iqqf rtqitcoogt jcxg c ngv kp eqooqp0"
A-4: In the list obtained after executing task(s) from Part(A-3), remove newline characters (if any).
A-5: For the list obtained after executing task(s) from Part(A-4), for each word in the list do the
following:
• Breakdown the word into list of letters.
• Convert each of the above letters into integer, using ord() function.
• Update the integer values by -2 for all the above integers.
• Convert each updated integer value into letter, using chr() function.
Join the updated letters in the order to create new word.
A-6: Join the list of new words obtained after executing task(s) from Part(A-5), into one string of words.
Print the string.
A-7: Create a python program, that does the following:
• Ask the user to enter a number from the following choices: 10, 20, 30, 40, 50, 60, 70, 80, 90, 100
• Returns the roman numerals and the word form for the entered number.
Hint(A-5): 'a' is chr(97), and ord('a') is 97.
Hint(A-7): Use the following list for roman numerals correspondingly to the above numbers:
“X", “XX", “XXX",“XL",“L", “LX",“LXX", “LXXX",“XC™, “C"
r Note: Solve all the above questions using Python only. Do NOT use Numpy or any other libraries.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcf5cca0a-4e82-412e-9186-34cd0934c52e%2Fd4ae6726-12be-4a14-a266-eea57dd7bfd8%2Ftas37pr_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 2 images

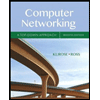
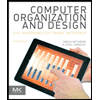
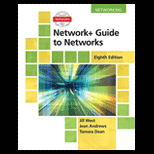
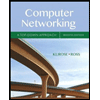
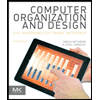
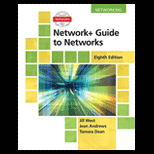
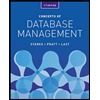
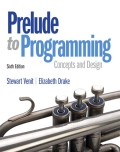
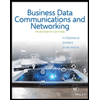