1. def make_grid(w: int, h: int, player_coord: Tuple[int, int], gold_coord: Tuple[int, int]) -> List[List[str]]: """ Given two integers width w and height h, create a list of lists to represent a grid of the given width and height. The coordinates for the player and the gold is also given as two two-element tuples. For each tuple, the first element has the x-coordinate and the second element has the y-coordinate. The player and the gold should be included in the grid in the positions specified by these tuples. The player is represented with the string '(x)' The gold is represented with the string '(o)' All other spaces are represented with the string '(_)' Return this list. >>> make_grid(2, 3, (0, 0), (1, 2)) [['(x)', '(_)'], ['(_)','(_)'], ['(_)', '(o)']] """ 2. def update_grid(grid: List[List[str]], w: int, h: int, px: int, py: int, dx: int, dy: int) -> Optional[Tuple[int, int]]: """ Given the player's current position as px and py, and the directional changes in dx and dy, update the given grid to change the player's x-coordinate by dx, and their y-coordinate by dy. More information: Use the given w and h (representing the grid's width and height) to figure out whether or not the move is valid. If the move is not valid (that is, if it is outside of the grid's boundaries), then NO change occurs to the grid. The grid stays the same, and nothing is returned. If the move IS possible, then the grid is updated by adding dx to the player's x-coordinate, and adding dy to the player's y-coordinate. The new position in the grid is changed to the player icon '(x)', and the old position the player used to be in is changed to an empty space '(_)'. The new x- and y- coordinates of the player is returned as a tuple. This function does NOT create or return a new grid. It modifies the "grid" list that is passed into it directly. >>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']] >>> update_grid(L, 2, 3, 0, 0, 1, 0) (1, 0) >>> L [['(_)', '(x)'], ['(_)', '(o)'], ['(_)', '(_)']] >>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']] >>> update_grid(L, 2, 3, 0, 0, 0, 1) (0, 1) >>> L [['(_)', '(_)'], ['(x)', '(o)'], ['(_)', '(_)']] >>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']] >>> print(update_grid(L, 2, 3, 0, 0, -1, 0)) None >>> L [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']] """
1. def make_grid(w: int, h: int, player_coord: Tuple[int, int],
gold_coord: Tuple[int, int]) -> List[List[str]]:
"""
Given two integers width w and height h, create a list
of lists to represent a grid of the given width and height.
The coordinates for the player and the gold
is also given as two two-element tuples. For each tuple,
the first element has the x-coordinate and the
second element has the y-coordinate. The player
and the gold should be included in the grid in
the positions specified by these tuples.
The player is represented with the string '(x)'
The gold is represented with the string '(o)'
All other spaces are represented with the string '(_)'
Return this list.
>>> make_grid(2, 3, (0, 0), (1, 2))
[['(x)', '(_)'], ['(_)','(_)'], ['(_)', '(o)']]
"""
2. def update_grid(grid: List[List[str]], w: int, h: int, px: int, py: int,
dx: int, dy: int) -> Optional[Tuple[int, int]]:
"""
Given the player's current position as px and py,
and the directional changes in dx
and dy, update the given grid to change the player's
x-coordinate by dx, and their y-coordinate by dy.
More information:
Use the given w and h (representing the grid's width
and height) to figure out whether or not the move is valid.
If the move is not valid (that is, if it is outside
of the grid's boundaries), then NO change occurs to the grid.
The grid stays the same, and nothing is returned.
If the move IS possible, then the grid is updated
by adding dx to the player's x-coordinate,
and adding dy to the player's y-coordinate.
The new position in the grid is changed to the player
icon '(x)', and the old position the player used to be in
is changed to an empty space '(_)'. The new x- and y- coordinates
of the player is returned as a tuple.
This function does NOT create or return a new grid.
It modifies the "grid" list that is passed into it directly.
>>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']]
>>> update_grid(L, 2, 3, 0, 0, 1, 0)
(1, 0)
>>> L
[['(_)', '(x)'], ['(_)', '(o)'], ['(_)', '(_)']]
>>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']]
>>> update_grid(L, 2, 3, 0, 0, 0, 1)
(0, 1)
>>> L
[['(_)', '(_)'], ['(x)', '(o)'], ['(_)', '(_)']]
>>> L = [['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']]
>>> print(update_grid(L, 2, 3, 0, 0, -1, 0))
None
>>> L
[['(x)', '(_)'], ['(_)', '(o)'], ['(_)', '(_)']]
"""
3. Add some sort of scoring

Step by step
Solved in 2 steps with 1 images

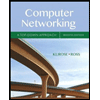
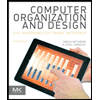
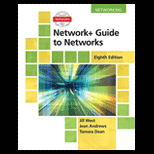
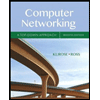
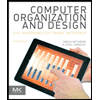
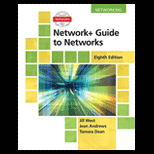
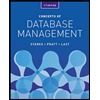
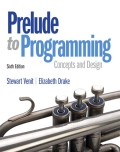
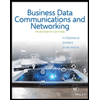