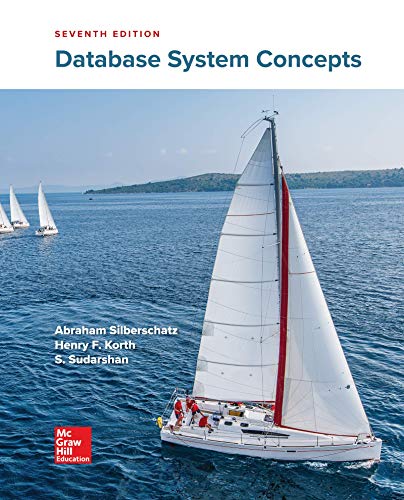
/**
Iluustration of RecursiveTask
*/
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
public class SumWithPool{
public static void main(String[] args) throws InterruptedException,
ExecutionException {
//get the number of avaialbe CPUs
int nThreads = Runtime.getRuntime().availableProcessors();
System.out.println("Available CPUs: " + nThreads);
//create an array of data
int n = 10; //initital data size
if(args.length > 0) // use the user given data size
n = Integer.parseInt(args[0]);
int[] numbers = new int[n];
for(int i = 0; i < numbers.length; i++) {
numbers[i] = i;
}
ForkJoinPool forkJoinPool = new ForkJoinPool(nThreads);
Sum s = new Sum(numbers,0,numbers.length);
long startTime = System.currentTimeMillis(); //start timer
Long result = forkJoinPool.invoke(s);
long endTime = System.currentTimeMillis();
long timeElapsed = endTime - startTime;
System.out.println("Concurrent executing time: " + timeElapsed + "ms");
System.out.println("The conccurent sum: " + result);
//redo the task sequentially
startTime = System.currentTimeMillis(); //start timer
result = sum(numbers);
endTime = System.currentTimeMillis();
timeElapsed = endTime - startTime;
System.out.println("\nSequential executing time: " + timeElapsed + "ms");
System.out.println("The sequential sum: " + result);
}
/** sequential sum */
private static long sum(int[] arr){
long sum = 0;
for(int i = 0; i < arr.length; i++)
sum += arr[i];
return sum;
}
}
/** create a class that handle a task */
class Sum extends RecursiveTask<Long> {
private int low;
private int high;
private int[] array; //only reference to the given data
/** map the attribute to the given data */
public Sum(int[] array, int low, int high) {
this.array = array;
this.low = low;
this.high = high;
}
/** overriding tehe comput method */
protected Long compute() {
if(high - low <= 10) { //do the sum if the block of data is managable
long sum = 0;
for(int i = low; i < high; ++i)
sum += array[i];
return sum;
} else {
int mid = low + (high - low) / 2;
Sum left = new Sum(array, low, mid);
Sum right = new Sum(array, mid, high);
left.fork();
long rightResult = right.compute();
long leftResult = left.join();
return leftResult + rightResult;
}
}
}
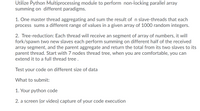

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 6 images

- Write code in Java: - Code must be recursive. import java.util.*; import java.lang.*; import java.io.*; class BinaryToDecimal { // *More methods can be added* public static int binaryToDecimal(String binaryString) { // *Code goes here* } } class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); System.out.print(BinaryToDecimal.binaryToDecimal(input.nextLine())); } }arrow_forwardAnswer it in java languagearrow_forwardWrite a recursive method called reverseString() that takes in a string as a parameter and returns the string in reversed order. The main method is provided to read a string from the user and call the reverseString() method. Ex: If the input of the program is: Hello the reverseString() method returns and the program outputs: Reverse of "Hello" is "olleH". Ex: If the input of the program is: Hello world! the reverseString() method returns and the program outputs: Reverse of "Hello, world!" is "!dlrow, olleH". Hint: Move the first character to the end of the returning string and pass the remaining sub-string to the next reverseString() method call.arrow_forward
- In java if i have this pseudo code that tries to explain how a method will work using recursion how can i turn this into working java codearrow_forwardIn java pls! here is some starter code for this problem: public class ThreadingDivisors { public static void main(String[] args) { long start = System.currentTimeMillis(); int maxDivisors = 0; int answer = 0; for (int n=1; n<100000; n++) { int divisors = getNumDivisors(n); if (divisors > maxDivisors) { maxDivisors = divisors; answer = n; } } System.out.println(answer + " has the most divisors (" + maxDivisors + ")"); long end = System.currentTimeMillis(); System.out.println(end - start + " milliseconds"); } public static int getNumDivisors(int n) { int numDivisors = 0; for (int i=1; i<=n; i++) { if (n % i == 0) { numDivisors++; } } return numDivisors; } }arrow_forwardInfoProcessorTest.java import java.util.*; /** * * Class to process and extract information from a list of lines. * */ public class InfoProcessorTest { /** * * List of lines to process. * */ private ArrayList<String> lines =new ArrayList<String>(); /** * * Creates InfoProcessor with given list of lines. * * @param lines to process * */ public InfoProcessorTest(ArrayList<String> lines) { this.lines = lines; } /** * * Gets the course name from the list of lines. * * First, finds the line that starts with "Course:". * * Second, gets the String on the very next line, which should be the course * name. * * Third, returns the String from the method. * * * * Hint(s): * * - Use the getNextStringStartsWith(String str) method to find the course name * * * * Example(s): * * - If the ArrayList<String> lines contains: "Course:" and "CIT590", and we * call * * getCourseName(), we'll get "CIT590". * * * * - If the ArrayList<String> lines contains: "Course:"…arrow_forward
- Can i get help with this question, java language please 1 class QuicksortTester2 {34 /**5 * Quicksort algorithm (driver)6 */78 public void quicksort( int [ ] a )9 {10 quicksort( a, 0, a.length - 1 );11 }1213 /**14 * Internal quicksort method that makes recursive calls.15 * Uses median-of-three partitioning and a cut-off.16 */17public void quicksort( int [ ] a, int low, int high )19 {20 if( low + CUTOFF > high )21 insertionSort( a );22 else23 { // Sort low, middle, high24 int middle = ( low + high ) / 2;25 if( a[ middle ].compareTo( a[ low ] ) < 0 )26 swapReferences( a, low, middle );27 if( a[ high ].compareTo( a[ low ] ) < 0 )28 swapReferences( a, low, high );29 if( a[ high ].compareTo( a[ middle ] ) < 0 )30 swapReferences( a, middle, high );3132 // Place pivot at position high - 133 swapReferences( a, middle, high - 1 );34 int pivot = a[ high - 1 ];3536 // Begin partitioning37 int i, j;38 for( i = low, j = high - 1; ; )39 {40 while( a[ ++i ].compareTo( pivot ) < 0 )41…arrow_forwardcreate a recursive decent parse that read ( 1 + 2 ) / 3 from a txt file import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner;import java.util.StringTokenizer; public class Lexical {public static void main(String args[]) throws FileNotFoundException{//reading file (Create one and put the expression (2+5/t) or (1-9)*0)Scanner input = new Scanner(new File("Expression.txt"));while(input.hasNextLine()) {//reading next lineString line = input.nextLine();//reading tokens by spaceStringTokenizer strings = new StringTokenizer(line," ");//reading more stringswhile (strings.hasMoreElements()) {//converting object to stringString token = strings.nextElement().toString();switch(token) //switch case{case "(" :System.out.println("Next token is: 25 Next lexeme is (");break;case ")" :System.out.println("Next token is: 26 Next lexeme is )");break;case "=" :System.out.println("Next token is: 20 Next lexeme is =");break;case "+" :System.out.println("Next token is: 21 Next…arrow_forwardimport java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forward
- RPN.java import java.util.Scanner; /** * Reverse Polish Notation calculator. It evaluates * a string with expressions in RPN format and prints * the results. Exampel of a stack use. */public class RPN{ /** * Given a string, return an integer version of * the string. Check if the string contains only * numbers, if so, then does the conversion. If * it does not, it reurns 0. * @param t string with numeric token * @return int version of the numeric token in t */ public int getValue(String t) { if (t.matches("[0-9]+")) { return Integer.parseInt(t); } else { return 0; } } /** * Evaluates a single token in an RPN expression. If it is * a number, it pushes the token to the stack. If it is an * operator, then it pulls 2 numbers from the stack, performs * the operation and pushes back into the stack the result. * @param token to be evaluated * @param stack holding values for…arrow_forwardMachine Problem #4. Design a method-oriented Java program with ArrayList of size 10 having 10 integervalues. Get the sum, average, highest, and lowest value. Class Name: MethodArrayListGradesSample Input/OutputThe Array List values are:86909086888492888888The sum is: 880The average is: 88The highest value is: 92The lowest value is: 84arrow_forwardWrite a Java method using recursion that reorders an integer array in such a way that all the even values comes before the odd values Here is the code to start, fill in the missing part output should be 8 6 2 4 4 4 4 3 5 5 3 7 1 9arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
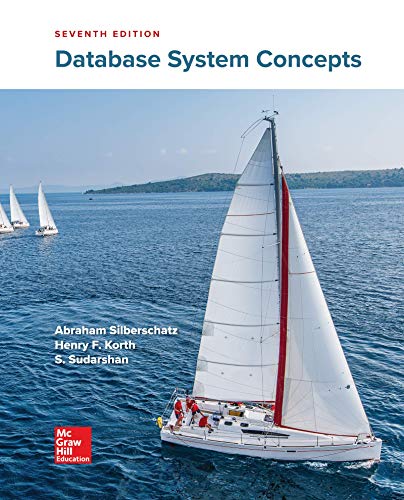
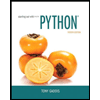
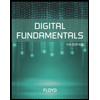
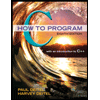
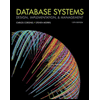
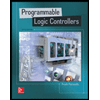