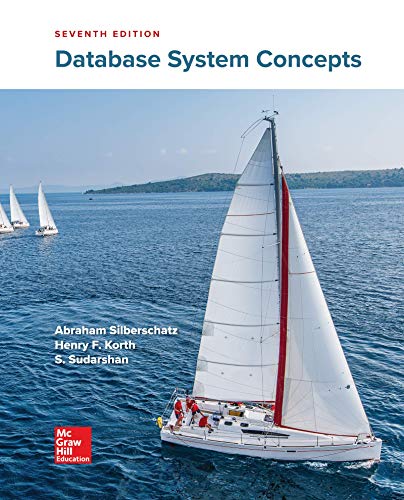
Concept explainers
java
final static Random rand = new Random(); final static int MAX_SIZE = 10; // amount of storage for the intList static int size = 0; // number of values actually in the intList static int[] intList = new int[MAX_SIZE]; /** * @param args the command line arguments */ public static void main(String[] args) { out.println("CPS 151 In-class assignment 2 by _______________________"); setRandom(intList, 100); printList(intList, MAX_SIZE, "\nArray before sorting"); sort(intList); printList(intList, MAX_SIZE, "\nArray after sorting"); } // end main // prints partially filled array with a legend private static void printList(final int[] arr, final int size, final String legend) { out.println(legend); for (int k = 0; k < size; k++) { out.print(" " + arr[k]); } out.println(); } // end printList // move items from pos:size-1 one position down (higher subscripts) private static void shiftDown(int[] arr, int size, int pos) { // Write the code (use code from ICA 1) } // end shiftDown private static void setRandom(int[] arr, final int range) { for (int k = 0; k < arr.length; k++) { arr[k] = rand.nextInt(range); } } // end setRandom private static void sort(int[] arr) { for (int k = 1; k < arr.length; k++) { // assume arr[0:k-1] sorted int save = arr[k]; // arr[k] may be overwritten later, so save that value int pos = findPos(arr, k, save); // find where to insert save in arr[0:k-1] shiftDown(arr, k, pos); arr[pos] = save; // arr[0:k] is now sorted with k+1 values printList(arr, MAX_SIZE, "\nAfter pass " + k); } // end for } // end sort
![±
21:01
६६/-/\\
!
ENG
This assignment uses some concepts developed in ICA 1. In particular, the shiftDown method developed for
ICA 1 can be reused.
When completed, this program will create a random array with 10 integer values and sort it using Insertion sort
technique. Most of the code is written. You should be able to reuse code for shiftDown method. You need to
complete the findPos method, following the description given later.
Submit the source code and screen shot as described in the document "How to submit assignments" through
Isidore. Follow naming conventions as already described.
6
18
35
45
56
77
91
Design of "find Pos" method
The findPos method is used to find the right place to insert a new value in a partially filled array. The partially filled
array is assumed to be sorted in increasing order and if the new value is inserted at the index position (as calculated by
findPos) then the resulting array will remain sorted. This is the method specification given for findPos:
// find the right place for item to be inserted within arr [0:size-1]
private static int findPos (int[] arr, int size, int item) {
(12, 23, 45, 67, 88). Note that there may be more elements in axr but as a
Suppose size = 5 and arr =
partially filled array, only 5 values are relevant. These are the values returned by the method for various possible values
of item.
item
@ A
A- F2
return value from findPos (arr, 5, item)
0
12 Y
W
1
2
3 (new 45 goes after old 45's)
3
34
4
5
You can start a position variable at 0 and keep incrementing it as long as the position is < size and item is >
arr[position]. The final value is the return value from the method. Note: It is also possible to write the loop backward.
OEI
#
3
F3
E
X
+
F4
$
4
с
75%
F5
R
团
F6
T
0
%
50
E
1 / 2
F7
FB
&
7 V
6 17
Y
100
D
U
8
FO](https://content.bartleby.com/qna-images/question/44ae875a-f598-455b-b111-f9246b312123/50ec752b-e4d8-46aa-b774-6ee85c0eae25/hhhxyya_thumbnail.jpeg)
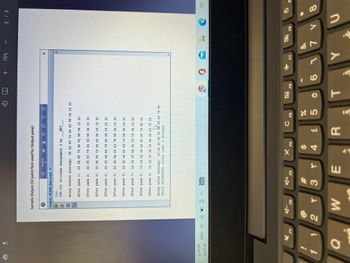

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- java programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export as text file, not print the console's order receipt. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items and…arrow_forwardimport java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forward1. Divisors: In a class Divisors.java, read in a maximum integer n and use nested loops to print a list of divisors for each of 1 through n as shown in the following transcript. Sample transcript (input underlined): Largest integer? 100 1: 1 2: 1 2 3: 1 3 4: 1 2 4 95: 1 5 19 95 96: 1 2 3 4 6 8 12 16 24 32 48 96 97: 1 97 98: 1 2 7 14 49 98 99: 1 3 9 11 33 99 100: 1 2 4 5 10 20 25 50 100arrow_forward
- class Output{public static void main(String args[]){byte a[] = { 65, 66, 67, 68, 69, 70 };byte b[] = { 71, 72, 73, 74, 75, 76 };System.arraycopy(a , 0, b, 0, a.length);System.out.print(new String(a) + " " + new String(b));}} The output of the above Java code is ?arrow_forwardpublic class Main { static int findPosSum(int A[], int N) { if (N <= 0) return 0; { if(A[N-1]>0) return (findPosSum(A, N - 1) + A[N - 1]); else return findPosSum(A, N - 1); } } public static void main(String[] args) { int demo[] = { 11, -22, 33, -4, 25,12 }; System.out.println(findPosSum(demo, demo.length)); } } Consider the recursive function you wrote in the previous problem. Suppose the initial call to the function has an array of N elements, how many recursive calls will be made? How many statements are executed in each call? What is the total number of statements executed for all recursive calls? What is the big O for this function?arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- using System; class TicTacToe { staticint player = 1; staticint choice; staticvoid Print( char[] board); staticvoid Main(string[] args) { Console.WriteLine(); Console.WriteLine($" {board[0]} | {board[1]} | {board[2]} "); Console.WriteLine($" {board[3]} | {board[4]} | {board[5]} "); Console.WriteLine($" {board[6]} | {board[7]} | {board[8]} "); Console.WriteLine(); } staticvoid Main(string[] args) { char[] board = newchar[9]; for (int i = 0; i < 9; i = i + 1) { board[i] = ' '; if( board[i]== 'O') Console.Write("It is your turn to place an X"); } Print(board); board[4] = 'X'; Print(board); board[0] = 'O'; Print(board); board[3] = 'X'; Print(board); board[5] = 'O'; Print(board); board[6] = 'X'; Print(board); board[2] = 'O'; Print(board); } } Hello! I need help with getting my code to compile. It's written in C# and i'm a beginner. it's giving me two errors and i'm not sure how to fix them. Please don't copy paste an answer from google.arrow_forwardJava Programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt (I'm missing the menu items.) Task: When the user selects the items they would like to order on the physical receipt it should display the items as well. Can this be completed? My program is below: import java.util.Scanner; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and prices String[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"}; double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99}; StringusersName; // The user's name, as entered by the user. // Define scanner object Scannerinput=newScanner(System.in); // Welcome message System.out.println("Welcome to AppleStoreRecreation, what is your name:"); usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to…arrow_forwardpublic class Program7 { publicstaticvoidmain(String[]args){ int[]numbers=newint[100]; String[]names={"Maria","Aris","Galin","Galena"}; double[]balances=newdouble[]{2,56,12.57,36.57,57.89}; //length System.out.println(numbers.length); System.out.println(names.length); System.out.println(balances.length); //modifying names[3]="Celine Dion"; System.out.println("__________________________"); //looping for(inti=0;i<names.length;i++){ System.out.println(names[i]); } System.out.println("__________________________"); for(Stringname:names){ System.out.println(name); } //modifying array System.out.println("__________________________"); for(inti=0;i<names.length;i++){ names[i]=names[i]+" Annan"; System.out.println(names[i]); } System.out.println("__________________________"); doubletotal=0.0; for(inti=0;i<balances.length;i++){ total+=balances[i]; } System.out.println("Total Balance: "+ total); } } //calculate the average of the total balancearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
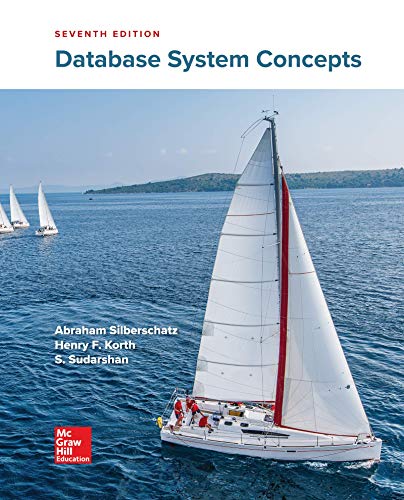
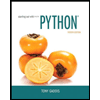
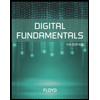
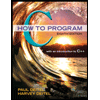
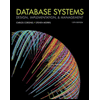
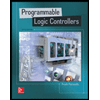