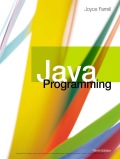
Explanation of Solution
Program:
File name: “SalespersonDatabase.java”
//Import necessary header files
import java.util.*;
//Define a class named SalespersonDatabase
public class SalespersonDatabase
{
//Define main method
public static void main(String[] args)
{
//Create an array of objects
SalesPerson[] salespeople = new SalesPerson[20];
//Declare variables
int x;
int id;
int count = 0;
double sales;
final int QUIT = 999;
char option;
String message = "";
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Prompt the user to enter an option
System.out.print("Do you want to (A)dd, (D)elete, or (C)hange a record or (Q)uit >> ");
option = keyboard.nextLine().charAt(0);
//While condition exists if option entered by the user is not equal to Quit
while(option != 'Q')
{
//If option is to Add a record
if(option == 'A')
count = addOption(salespeople, count);
//If option is to Delete a record
else
if(option == 'D')
count = deleteOption(salespeople, count);
//If option is to Change a record
else
if(option == 'C')
changeOption(salespeople, count);
//Invalid
else
//Print the result
System.out.println("Invalid entry");
//Prompt the user to enter an option
System.out.print("Do you want to (A)dd, (D)elete, or (C)hange a record or (Q)uit >> ");
option = keyboard.nextLine().charAt(0);
}
}
//Define a method for add option
public static int addOption(SalesPerson[] array, int count)
{
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Declare variables
int id;
double sales;
boolean alreadyEntered;
//Check if
if(count == array.length)
//Print the result
System.out.println("Sorry - array is full -- cannot add a record");
//if database is not full
else
{
//Prompt the user to enter the ID of the salesperson
System.out.print("Enter salesperson ID >> ");
id = keyboard.nextInt();
alreadyEntered = false;
for(int x = 0; x < count; ++x)
//Check if ID number already exists
if(array[x].getId() == id)
{
//Print the result
System.out.println("Sorry -- ID number already exists");
alreadyEntered = true;
}
//Check if ID number does not exists
if(!alreadyEntered)
{
//Prompt the user to enter the sales
System.out.print("Enter sales >> ");
sales = keyboard.nextDouble();
array[count] = new SalesPerson(id, sales);
//Increment the count
++count;
}
}
//Display the database values
display(array, count);
keyboard.nextLine();
//Return the value
return count;
}
//Define a method for delete option
public static int deleteOption(SalesPerson[] array, int count)
{
//Create an object for Scanner class
Scanner keyboard = new Scanner(System.in);
//Declare variables
int id;
int position = 0;
//Check if database is empty
if(count == 0)
//Print the result
System.out.println("Cannot delete - no records in database");
//if database is not empty
else
{
//Prompt the user to enter the ID of the salesperson
System.out.print("Enter ID to delete >> ");
id = keyboard.nextInt();
boolean exists = false;
for(int x = 0; x < count; ++x)
//Check if ID number already exists
if(array[x].getId() == id)
{
exists = true;
position = x;
}
//if ID number does not exists
if(!exists)
//Print the result
System...

Trending nowThis is a popular solution!

- you have to write an application that helps with monthly bill payments. You have to write an sql code that creates the appropriate database. As the second part you need to write an application that will allow a user to add a payment, add a contractor, display payments for a given month. Additionally, when a user selects a month, your application should provide information of the expenses he/she need to pay that month. Hint: the selected month is a new entry in the database. Java codesarrow_forward2. load_friendsdb This function does the reverse of save_friendsdb -- it takes a single argument, filename, and opens that specified file for reading. Then it reads the file one line at a time and creates a new friends database to return. Like in the previous function, each line will contain a friend's name followed by a tab, then the friend's height, and then a newline. Sample calls should look like this: >>> load friendsdb("friendsdb.tsv") [{'name': ' bimmy', 'height': 600}, {'name': 'Ian Donald Calvin Euclid Zappa', height': 175}]arrow_forward2. load_friendsdb This function does the reverse of save_friendsdb -- it takes a single argument, filename, and opens that specified file for reading. Then it reads the file one line at a time and creates a new friends database to return. Like in the previous function, each line will contain a friend's name followed by a tab, then the friend's height, and then a newline. Sample calls should look like this: >>> load_friendsdb("friendsdb.tsv") [{'name': ' bimmy', 'height': 600}, {'name': Ian Donald Calvin Euclid Zappa', height': 175}]arrow_forward
- The Save Transaction button depicted in the screen attached is used to save relevant data to the sales table and the salesdetails tables from the depicted schema. When this button is clicked it calls the saveTransaction() function that is within the PosDAO class, it passes to this function an ArrayList of salesdetails object, this list contains the data entered into the jTable which is the products and qty being sold.Write the saveTransaction function. You are to loop through the items and get the total sales, next you are to insert the current date and the total sales into the sales table. Reminder that the sales table SalesNumber field is set to AUTO-INCREMENT, hence the reason for only entering the total sales and current date in sales table.arrow_forwardCompulsory Task Follow these steps: HHyperion Dev ● Create a program that can be used by a bookstore clerk. The program should allow the clerk to: add new books to the database ● O id O update book information O O Create a database called ebookstore and a table called book. The table should have the following structure: 3001 3002 3003 3004 delete books from the database search the database to find a specific book 3005 title A Tale of Two Cities The Lion, the Witch and the Wardrobe Harry Potter and the Philosopher's J.K. Rowling Stone The Lord of the Rings Alice in Wonderland author Charles Dickens 1. Enter book 2. Update book 3. Delete book 4. Search books 0. Exit C. S. Lewis J.R.R Tolkien Lewis Carroll qty 30 40 25 37 12 ● Populate the table with the above values. You can also add your own values if you wish. The program should present the user with the following menu: Copyright © 2021 Hyperion Dev. All rights reserved.arrow_forwardA computer store uses a database to track inventory. The database has a table named Inventory , with the following columns: ProductID INTEGER PRIMARY KEY NOT NULL ProductName TEXT QtyOnHand INTEGER Cost REAL Also assume that the cur variable references a Cursor object for the database. Write Python code that uses the Cursor object to execute an SQL statement that deletes the row in the Inventory table in which ProductID is equal to 12.arrow_forward
- Write an application that creates a database named Personnel. The database should have a table named Employee, with columns for employee ID, name, position, and hourly pay rate. The employee ID should be the primary key. Insert at least five sample rows of data into the Employee table. JAVA PLEASE!arrow_forwardIn java create an application to manage your data base information the database is already created in mysql tables, the tables are fill, So the user can use your database application user friendly Retrieve all data: Given a table name, retrieve all data from the table and present it to the user. Average: Given a table name and a column name, return the average of the column. Here the assumption is that the column type will be numeric (e.g., cost column). Insert: Given a table name, your program should show the column names of that table and ask the users to input new data to the table. In case of errors, your program should directly show the MySQL errors to the users. Assumption 1: Users will enter data according to the database constraints. Assumption 2: Users will input one record at a time. Delete: Given a table name, your program should show the column names of that table and ask the users to input data that they want to delete. Assumption 1: Users will enter data according to the…arrow_forwardBuild an application for a car rental company. It should offer the following features: Maintain a customer database in a file: Register / add new customers. Customer information should include Customer ID number Name Phone number Search customer database by: Customer ID number Name Phone number And print out the matching records Maintain a database in a file: Add a new vehicle to the fleet. Vehicle information should include Model year Make Model name License plate number Customer number who is currently renting vehicle Delete a vehicle from the fleet Rent a vehicle to a registered customer Receive rented vehicle back from customer Search vehicle database by any field: Model year + Make + Model name License plate number Customer number who is currently renting vehicle And print out all matching recordsarrow_forward
- Build an application for a car rental company. It should offer the following features: Maintain a customer database in a file: Register / add new customers. Customer information should include Customer ID number Name Phone number Search customer database by: Customer ID number Name Phone number And print out the matching records Maintain a database in a file: Add a new vehicle to the fleet. Vehicle information should include Model year Make Model name License plate number Customer number who is currently renting vehicle Delete a vehicle from the fleet Rent a vehicle to a registered customer Receive rented vehicle back from customer Search vehicle database by any field: Model year + Make + Model name License plate number Customer number who is currently renting vehicle And print out all matching records ооооarrow_forwardDon't copy from other websties. Objective: To create a JSON for Population census with objects and arrays Scenario: ABC Census Company is maintaining database on the survey taken. Application sends the details of schemes in the form of JSON Help to create a JSON with the information provided. Name Datatype of value Country String State String YearOfSurvey Number TotalPopulation String AgeGroup Object AgeGroup- Object : Name Datatype of value 0-14 Array 15-24 Array 25-44 Array 45-64 Array Above65 Array Array : Name Datatype of value male Number female Number You can assume any data for the mentioned JSON structure, provided it is matching the specified datatypearrow_forwardBlood Donor Management System The blood donation clinic is looking at creating a database to store the donors’ details. The clinic is hoping to start by adding donor names and assign them unique IDs. They then want to add their details and relevant information such as blood type, first name, last name and contact number. Write an interactive program that allows a clinic administrator to store donors’ details on the SQLite 3 database with the following specifications: 2.1 The program must have a menu for the administrator to select one of the following:• Register a donor• Display the record of all registered donors• Update the existing record• Delete a record• Exit the program 2.2 The program should display feedback messages for any successfully executed task and use exception handlers for any errors produced in the code. 2.3 The program must have the following functions:• The donor_register function to add donors• The donor_update function to update the donor details• The donor_remove…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
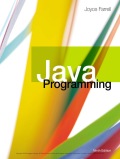
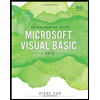