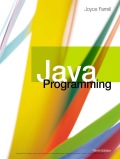
Explanation of Solution
Program:
File name: “Salesperson.java”
//Define a class named Salesperson
public class Salesperson
{
//Declare the private variables
private int id;
private double sales;
/*Define a method named Salesperson
that takes two arguments*/
Salesperson(int idNum, double amt)
{
//Assign the values
id = idNum;
sales = amt;
}
//Define a get method that returns the ID number
public int getId()
{
//Return the value
return id;
}
//Define a get method that returns the sales value
public double getSales()
{
//Return the value
return sales;
}
//Define a set method that takes the ID number
public void setId(int idNum)
{
//Assign the value
id = idNum;
}
//Define a set method that takes the sales value
public void setSales(double amt)
{
//Assign the value
sales = amt;
}
}
File name: “SalespersonSort.java”
//Import necessary header files
import javax.swing.*;
//Define a class named SalespersonSort
public class SalespersonSort
{
//Define a main method
public static void main(String[] args)
{
//Declare an array to store seven Salesperson objects
Salesperson[] salespeople = new Salesperson[7];
//Declare the variables
int x;
int id;
double sales;
String order;
String message = "";
//For loop to be executed until x exceeds 7
for(x = 0; x < salespeople.length; ++x)
{
//Prompt the user to enter an ID number
id = Integer.parseInt(JOptionPane.showInputDialog(null,
"Enter an ID number"));
//Prompt the user to enter the sales value
sales = Double.parseDouble(JOptionPane.showInputDialog(null,
"Enter sales value"));
salespeople[x] = new Salesperson(id, sales);
}
/*Prompt the user to enter the choice of displaying
the objects in order by either ID number or sales value*/
order = JOptionPane.showInputDialog(null,
"By which field do you want to sort?\n" +
"(I)d number or (S)ales");
//If the user enters the choice, ID number
if(order.charAt(0) == 'I')
//Function call
sortById(salespeople);
//Else the user enters the choice, sales value
else
//Function call
sortBySales(salespeople);
//For loop to be executed until x exceeds 7
for(x = 0; x < salespeople...

Trending nowThis is a popular solution!

- Creating Enumerations In this section, you create two enumerations that hold colors and car model types. You will use them as field types in a Car class and write a demonstration program that shows how the enumerations are used. 1. Open a new file in your text editor, and type the following Color enumeration: enum Color {BLACK, BLUE, GREEN, RED, WHITE, YELLOW}; 2. Save the file as Color.java. 3. Open a new file in your text editor, and create the following Model enumeration: enum Model {SEDAN, CONVERTIBLE, MINIVAN}; 4. Save the file as Model.java. Next, open a new file in your text editor, and start to define a Car class that holds three fields: a year, a model, and a color. public class Car { private int year; private Model model; private Color color; 5. Add a constructor for the Car class that accepts parameters that hold the values for year, model, and color as follows: public Car(int yr, Model m, Color c) { year = yr; model = m; color = c; } 6. Add a display()…arrow_forwardThis is the question I am stuck on - Radio station KJAVA wants a class to keep track of recordings it plays. Create a class named Recording that contains fields to hold methods for setting and getting a Recording’s title, artist, and playing time in seconds. Write an application that instantiates five Recording objects and prompts the user for values for the data fields. Then prompt the user to enter which field the Recordings should be sorted by—(S)ong title, (A)rtist, or playing (T)ime. Perform the requested sort procedure, and display the Recording objects. This is what I have so far - public class Recording { private String song; private String artist; private int playTime; public void setSong(String title) { this.song = title; } public void setArtist(String name) { this.artist = name; } public void setPlayTime(int time) { this.playTime = time; } public String getSong() { return song; } public String…arrow_forwardDesign an application that declares two Rectangle objects and sets and displays their values. Design an application that declares a Rectangle object using the second constructor and then displays the object's values Design an application that declares an array of 5 Rectangle objects. Prompt the user for data for each object, and then display all the values. In Pseducode Thanksarrow_forward
- Design an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then display all the values. Design an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then pass the array to a method that determines and displays the two stocks with the highest and lowest price per share.arrow_forwardCreate a class named Salesperson. Data fields for Salesperson include an integer ID number and a double annual sales amount. Methods include a constructor that requires values for both data fields, as well as get and set methods for each of the data fields. Write an application named DemoSalesperson that declares an array of 10 Salesperson objects. Set each ID number to 9999 and each sales value to zero. Display the 10 Salesperson objects.arrow_forwardWrite code that creates a Random object and then assigns a random integer in the range of 1 through 100 to the variable intRandomNumber.arrow_forward
- Create an application named SalesTransactionDemo that declares several SalesTransaction objects and displays their values and their sum. The SalesTransaction class contains the following fields: Name - The salesperson's name (as a string) salesAmount - The sales amount ( as a double) commission - The commission (as a double) RATE - A readonly field that stores the commission rate (as a double). Define a getRate() accessor method that returns the RATE Include three constructors for the class. One constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value times commission rate. The second constructor accepts a name and sales amount, but sets the commission rate to 0. The third constructor accepts a name and sets all the other fields to 0. An overloaded + operatoradds the sales values for two SalesTransaction objects and returns a new SalesTransaction object. In order to prepend the $ to…arrow_forwardExtra 7-2 Add a stopwatch to the Clock application In this exercise, you’ll add a stopwatch feature to a digital clock application. The stopwatch will display elapsed minutes, seconds, and milliseconds. 1. Open the application in this folder: exercises_extra\ch07\clock_stopwatch\ 2. In the JavaScript file, note the $(), displayCurrentTime(), padSingleDigit(), and DOMContentLoaded event handler functions from the Clock application. In addition, note the global variables and starting code for the tickStopwatch(), startStopwatch(), stopStopwatch(), and resetStopwatch() functions. 3. In the tickStopwatch() function, add code that adds 10 milliseconds to the elapsedMilliseconds variable and then adjusts the elapsedMinutes and elapsedSeconds variables accordingly. Then, add code that displays the result in the appropriate span tags in the page. 4. In the startStopwatch() function, add code that starts the stopwatch. Be sure to cancel the default action of the link too. 5. In the…arrow_forwardCreate two enumerations that hold colors and car modeltypes. You will use them as field types in a Car class and write ademonstration program that shows how the enumerations are used.arrow_forward
- Create an application named SalesTransactiobDemo that declares several SalesTransaction objects and displays their values and their sum. The SalesTransaction class contains the following fields:Name - The salesperson name (as a string)salesAmount - The sales amount (as a double)commission - The commission (as a double)RATE - A readonly field that stores the commission rate (as a double). Define a getRate () accessor method that returns the RATEINCLUDE three constructors for the class. On constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value times commission rate. The second constructor accepts a name and sales amount, but sets tge commission rate to 0.The third constructor accepts a name and sets all the other fields to 0.An overloaded + operator adds tge sales values for two SalesTransaction objects and returns a new SalesTransaction object.In order to prepend the $ to the currency…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forwardThis is the question - The developers of a free online game named Sugar Smash have asked you to develop a class named SugarSmashPlayer that holds data about a single player. The class contains the following fields: idNumber - the player’s ID number (of type int) name - the player's screen name (of type String) scores - an array of integers that stores the highest score achieved in each of 10 game levels Include get and set methods for each field. The get method for scores should require the game level to retrieve the score for. The set method for scores should require two parameters—one that represents the score achieved and one that represents the game level to be retrieved or assigned. Display an error message if the user attempts to assign or retrieve a score from a level that is out of range for the array of scores. Additionally, no level except the first one should be set unless the user has earned at least 100 points at each previous level. If a user tries to set a score for a…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
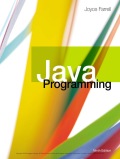
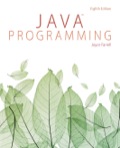