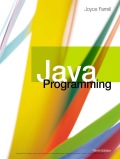
Concept explainers
a.
Explanation of Solution
Program:
File name: “Recording.java”
//Define a class named Recording
public class Recording
{
//Declare the variables
private String song;
private String artist;
private int playTime;
//Define a set method that takes the Recording’s title
public void setSong(String title)
{
//Assign the value
song = title;
}
//Define a set method that takes the Recording’s artist
public void setArtist(String name)
{
//Assign the value
artist = name;
}
/*Define a set method that takes the Recording’s playing time*/
public void setPlayTime(int time)
�...
b.
Explanation of Solution
Program:
File name: “RecordingSort.java”
//Import necessary header files
import javax.swing.*;
//Define a class named RecordingSort
public class RecordingSort
{
//Define a main method
public static void main(String[] args)
{
//Declare an array of five Recording objects
Recording[] recordings = new Recording[5];
//Declare the variables
int x;
String song, artist, timeString, order, message = "";
int time;
//For loop to be executed until x exceeds 5
for(x = 0; x < recordings.length; ++x)
{
//Prompt the user to enter a song for recording
song = JOptionPane.showInputDialog(null,
"Enter a song for recording " + (x + 1));
//Prompt the user to enter an artist for recording
artist = JOptionPane.showInputDialog(null,
"Enter an artist for recording " + (x + 1));
//Prompt the user to enter the time for the recording
timeString = JOptionPane.showInputDialog(null,
"Enter the time for the recording in seconds" + (x + 1));
time = Integer.parseInt(timeString);
//Declare a temp object
Recording temp = new Recording();
//Function Call
temp.setArtist(artist);
temp.setSong(song);
temp.setPlayTime(time);
//Assign the value
recordings[x] = temp;
}
/*Prompt the user to enter the choice of displaying
the objects in order by song title, artist, or playing time*/
order = JOptionPane.showInputDialog(null,
"By which field do you want to sort?\n" +
"(S)ong, (A)rtist, or (T)ime");
//If the user enters a choice, song title
if(order.charAt(0) == 'S')
//Function call
sortBySong(recordings);
//If the user enters a choice, Artist
else
if(order.charAt(0) == 'A')
//Function call
sortByArtist(recordings);
//Else the user enters a choice, playing time
else
//Function call
sortByTime(recordings);
//For loop to be executed until x exceeds 5
for(x = 0; x < recordings.length; ++x)
//Print the message
message = message + "\nartist: " + recordings[x].getArtist() +
" song: " + recordings[x].getSong() + " time: " +
recordings[x].getPlayTime();
JOptionPane.showMessageDialog(null, message);
}
/*Define a method to sort the Recording objects in
artist order*/
public static void sortByArtist(Recording[] array)
{
//Declare the variables
int a,b;
Recording temp;
String stringB, stringBPlus;
int highSubscript = array.length - 1;
//For loop to be executed until a exceeds highSubscript
for(a = 0; a < highSubscript; ++a)
//For loop to be executed until b exceeds highSubscript
for(b = 0; b < highSubscript; ++b)
{
stringB = array[b]...

Trending nowThis is a popular solution!

- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
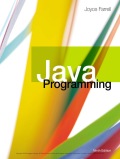
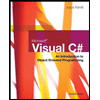
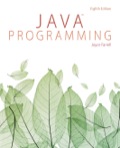