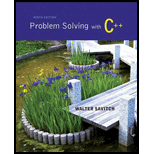
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 6.3, Problem 22STE
Suppose c is a variable of type char. What is the difference between the following two Statements?
cin >> c;
and
cin.get(c);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
i cant use static void Main(string[] args) for this assignment for C#
this is what I have to work with
using System;
using static System.Console;
class GuessingGame
{
static void Main()
{
// Write your main here
}
}
This problem relies on the generation of a random number. You can create a random number that is at least min but less than max using the following statements:
Random ranNumberGenerator = new Random();int randomNumber;randomNumber = ranNumberGenerator.Next(min, max);
Write a program named GuessingGame that generates a random number between 1 and 10. (In other words, in the example above, min is 1 and max is 11.)
Ask a user to guess the random number, then display the random number and a message indicating whether the user’s guess was too high, too low, or correct.
in c++
In this exercise, we will read in a data file of employees (file is furnished to you, “employee.dat”):
Employee SSN
Employee Last Name
Employee First Name
Hours Worked
Pay Per Hour
Personal Income Tax Rate
You assignment’s logic is to:
Read in the records from the file,
Pass the Hours Worked, Pay Per Hour, Personal Income Tax Rate to a single function to determine:
Whether or not overtime pay is computed (anything over 40 hours is overtime at 1.5 times the regular pay). Compute any overtime pay at 1.5 times pay rate
Compute the regular pay at regular hours (40 hours or less)
Compute the gross pay (Regular Pay plus any overtime pay)
Compute the personal income taxes withheld
Compute Net Pay (gross pay minus personal income taxes withheld)
Pass ALL these values BACK to the main calling routine from this one function.
Display:
the Employee SSN,
Last Name, First Name,
Total Hours,
Regular Hours,
Overtime Hours (if any),
Pay Per Hour,
Personal Income Tax Rate,
Regular Pay,…
in Swift Programming: The Big Nerd Ranch Guide (2nd Ed.) e-book: Repeat the silver challenge, but this time make sure that the formatting above matches what you print to the console. You may need to search the documentation for how to represent special characters in String literals (e.g., how do you represent a newline in a String?). You will also want to look at the documentation for the print() function to use a new parameter called terminator. This parameter defaults to printing a new line after each String logged to the console, but you will want to do something else. There are many ways to solve this challenge, but one way would be to print a String with a certain number of empty spaces (it is up to you to figure out how many!) when you print specific zip codes.
Chapter 6 Solutions
Problem Solving with C++ (9th Edition)
Ch. 6.1 - Prob. 1STECh. 6.1 - Prob. 2STECh. 6.1 - Suppose that you are still writing the same...Ch. 6.1 - Prob. 4STECh. 6.1 - Prob. 5STECh. 6.1 - Prob. 6STECh. 6.1 - Suppose bla is an object, dobedo is a member...Ch. 6.1 - Prob. 8STECh. 6.1 - Prob. 9STECh. 6.1 - A program has read half of the lines in a file....
Ch. 6.1 - Prob. 11STECh. 6.2 - Prob. 12STECh. 6.2 - Prob. 13STECh. 6.2 - Prob. 14STECh. 6.2 - What output will be sent to the stuff.dat when the...Ch. 6.2 - Prob. 16STECh. 6.2 - In formatting output, the following flag constants...Ch. 6.2 - Here is a code segment that reads input from...Ch. 6.2 - Prob. 19STECh. 6.2 - Write the definition for a void function called...Ch. 6.2 - (This exercise is for those who have studied the...Ch. 6.3 - Suppose c is a variable of type char. What is the...Ch. 6.3 - Suppose c is a variable of type char. What is the...Ch. 6.3 - Prob. 24STECh. 6.3 - Consider the following code (and assume that it is...Ch. 6.3 - Consider the following code (and assume that it is...Ch. 6.3 - Suppose that the program described in Self-Test...Ch. 6.3 - Consider the following code (and assume that it is...Ch. 6.3 - Prob. 29STECh. 6.3 - Define a function called copyLine that takes one...Ch. 6.3 - Prob. 31STECh. 6.3 - (This exercise is for those who have studied the...Ch. 6.3 - (This exercise is for those who have studied the...Ch. 6.3 - Suppose ins is a file input stream that has been...Ch. 6.3 - Write the definition for a void function called...Ch. 6.3 - Consider the following code (and assume that it is...Ch. 6.3 - Write some C++ code that will read a line of text...Ch. 6 - Write a program that will search a file of numbers...Ch. 6 - Write a program that takes its input from a file...Ch. 6 - a. Compute the median of a data file. The median...Ch. 6 - Write a program that takes its input from a file...Ch. 6 - Write a program that gives and takes advice on...Ch. 6 - Write a program that reads text from one file and...Ch. 6 - Prob. 7PCh. 6 - Write a program to generate personalized junk...Ch. 6 - Write a program to compute numeric grades for a...Ch. 6 - Enhance the program you wrote for Programming...Ch. 6 - Prob. 4PPCh. 6 - Write a program that will correct a C++ program...Ch. 6 - Write a program that allows the user to type in...Ch. 6 - This project is the same as Programming Project 6,...Ch. 6 - This program numbers the lines found in a text...Ch. 6 - Write a program that computes all of the following...Ch. 6 - The text file babynames2012.txt, which is included...Ch. 6 - To complete this problem you must have a computer...Ch. 6 - Write a program that prompts the user to input the...Ch. 6 - The following is an old word puzzle: Name a common...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Using an example of a component that implements an abstract data type such as a stack or a list, show why it is...
Software Engineering (10th Edition)
Write a complete Java program that reads in a line of keyboard input containing two values of type int (separat...
Absolute Java (6th Edition)
A new class of objects can be created conveniently bythe new class (called the subclass) starts with the charac...
Java How To Program (Early Objects)
Type in and run the program presented in this chapter. Check the programs results by comparing the original fil...
Programming in C
First and Last Design a program that asks the user for a series of names (in no particular order). After the fi...
Starting Out with Programming Logic and Design (4th Edition)
The _____ loop is ideal for situations that require a counter.
Starting Out with C++ from Control Structures to Objects (9th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Which operator can not be overloaded? (A) + (B) - (C) * (D) ::arrow_forwardhelp me towrite with carrow_forwardDevelop a substring operator using operator() in C++. The arguments should be the starting position for the substring and the length of the substring. The result should be a copy of the substring starting at the given position within our own data and ending at most the given length from the start. For example: Object's Value Operator Call Resulting Object's Value "bob wuz here" obj(0,4) "bob " "bob wuz here" obj(8,14) "here" (ran out of characters) "bob wuz here" obj(14,4) "" (nothing there) "bob wuz here" obj(4,1) "w" If the start position doesn't exist, send back an empty string (as we've defined). If there aren't 'length' characters left in our string after the given start position, return what we do have. Don't forget to write a test application to show that your operator works. (Perhaps you could modify the existing String test app?)arrow_forward
- INSTRUCTIONS: Write a C++ script/code to do the given problems. MOVIE PROBLEM: Write a function that checks whether a person can watch an R18+ rated movie. One of the following two conditions is required for admittance: The person is atleast 18 years old. • They have parental supervision. The function accepts two parameters, age and isSupervised. Return a boolean. Example: acceptIntoMovie(14, true) → true acceptIntoMovie(14, false) → falsearrow_forwardin C++ Language can you answer that questionarrow_forwardQuestion 3 Write a program in C, which simulates the game of connect 4, representing the board with coloured tiles, by means of matrices, where the player can choose whether to play with the computer and the computer represents player 2 or with another person, where at the end it shows the winner, the loser or if there was a draw. . Full explain this question very fast solution sent mearrow_forward
- By using Loop in c++, you are responsible to develop a Credit Limit Calculator, which will determine if their customer has exceeded the credit limit on a charge account. For each customer, the following facts are available:- a) Account number b) Balance at the beginning of the month c) Total of all items charged by this customer this month d) Total of all credits applied to this customer's account this month e) Type of Membership: SVIP(S), VIP(V) and Basic(B) Your program should input each for these facts, calculate the new balance (= beginning balance + charges - credit), and determine if the new balance exceeds the customer's credit limit. The customer's credit limit is based on their membership type as below:- Type of Membership SVIP (S) VIP (V) Basic (B) Credit Limit (HK$) 20,000 10,000 5,000 For those customers whose credit limit is exceed, the program should display the customer's account number, type of membership, credit limit, new balance and the message "Credit Limit"…arrow_forwardGiven two variables firstInClass and secondInClass which have already been associated with values, write code which swaps the values to which they are associated. For example, if firstInClass starts with the value "Pat", and secondInClass starts with the value "Wei", firstInClass should wind up associated with "Wei" and secondInClass should wind up associated with "Pat".(use Python)arrow_forwardT/F Can we compare a Boolean to int?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
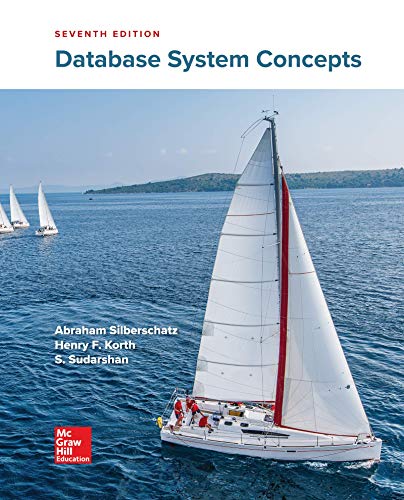
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
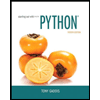
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
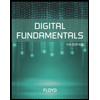
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
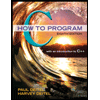
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
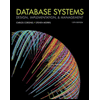
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
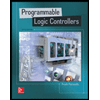
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
What is Abstract Data Types(ADT) in Data Structures ? | with Example; Author: Simple Snippets;https://www.youtube.com/watch?v=n0e27Cpc88E;License: Standard YouTube License, CC-BY